1. Overview
Club Connect is a computer application targeted at student club members at the National University of Singapore (NUS). It aims to make the tedious process of club management easier and more effective. It provides all members of the club with the ability to browse through profiles of other members, participate in polls, and manage club-related tasks.
Since Club Connect is targeted at those who prefer using the keyboard over a mouse, it uses a Command Line Interface (CLI) for input. However, all the output is displayed in a traditional Graphical User Interface (GUI) - which is just computer jargon for a display that includes panes, menus and message boxes.
2. About this portfolio
This project portfolio documents my contributions to the Club Connect project. It includes a summary of features that I implemented and other contributions to the project. Some extracts of my documentation in the Club Connect User Guide and Developer Guide are also included.
3. Summary of contributions
-
Major enhancement: This enhancement added functionality to manage information of club members.
-
What it does: The import feature adds all members in a specified CSV (comma separated values) file to Club Connect. Conversely, the export feature makes it possible to add the details of all members in Club Connect to a CSV file.
-
Justification: During recruitment events (such as the annual Student Life Fair at the National University of Singapore), club representatives collect data of prospective members using software like Microsoft Excel. They will then have to update Club Connect’s database to include the data of the inducted members. The
import
command allows Executive Committee (Exco) members to add all these new members in one go.
For club administration tasks where you need to get a list of all members of the club and their details (signing up all members to NUSync, for example), theexport
command comes in handy. It generates a file containing all the relevant information in a user friendly form. This information can then be easily managed and modified outside the application. -
Highlights: This enhancement is an end-to-end feature and makes use of almost all components of the software. Though the implementation of these features is intuitive and makes use of existing code wherever possible, it was challenging as it required the creation of a CsvUtil class (to handle parsing) from scratch. It is also made robust in order to prevent frustration in users.
-
-
Minor enhancement: This enhancement added support for a profile photo for each member.
-
What it does: This enhancement allows the logged in members to change their profile photo. Similarly, they can choose to remove their profile photo as well.
-
Justification: Since most student clubs have a large number of members, members may find it difficult to identify others by name alone. Having the ability to add a profile photo for yourself makes it much easier for others to identify you when they need to contact you.
-
Highlights: This enhancement is an end-to-end feature and makes use of almost all components of the software, from
Storage
toUi
. It was challenging as the feature had to handle the reading and writing of image files efficiently, and had to allow the application to remain portable. -
Relevant pull requests: #106
-
-
Code contributed: [Functional code] [Test code]
-
Other contributions:
-
Project management on GitHub:
-
Created necessary labels
-
Updated descriptions and labels of PRs (where necessary)
-
Organised and assigned issues
-
Managed release
v1.5rc
(1 release)
-
-
Enhancements to existing features:
-
Documentation:
-
Community:
-
4. Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
4.1. Major Enhancement: Member Data Management
This section contains extracts from the User Guide that are related to management of members' data.
Start of Extract (from User Guide)
Importing members into Club Connect: import
(Since v1.4)
Imports the details of all valid members in the specified CSV file into Club Connect.
Format: 'import CSV_FILE_PATH`
Alias: imp
This command is for Exco members only.
|
You cannot overwrite existing members (i.e. you cannot edit details of members in Club Connect) by using the import command.
|
You can save a Microsoft Excel spreadsheet as a CSV file by changing the file extension while saving the file. |
To get the absolute path to the CSV file, see Absolute path of a file. |
Examples:
-
import C:/Users/John Doe/Desktop/members.csv
Imports all members in the "members.csv" file onJohn Doe
's Desktop to Club Connect. -
import /Users/Jane Doe/Desktop/clubbook.csv
Imports all members in the "clubbook.csv" file onJane Doe
's Desktop to Club Connect.
Figure 22 shows the output of the import
command.
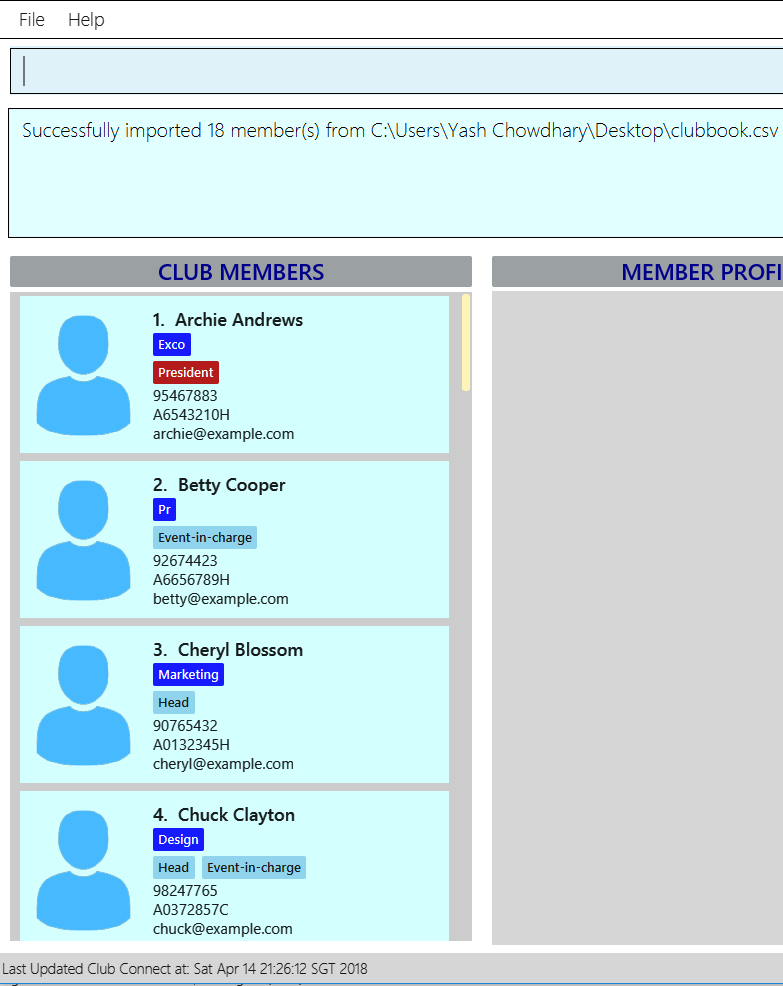
Exporting Club Connect members: export
(Since v1.3)
Exports the data of all members in Club Connect to a CSV file.
Format: 'export CSV_FILE_PATH`
Alias: exp
You can import the generated CSV file from Microsoft Excel to get an even better view of the data. |
To get the absolute path to the CSV file, see Absolute path of a file. |
Examples:
-
export C:/Users/John Doe/Desktop/members.csv
Exports all members in Club Connect to the "members.csv" file onJohn Doe
'sDesktop
. -
export C:/Users/Jane Doe/Desktop/clubbook.csv
Exports all members in Club Connect to the "clubbook.csv" file onJane Doe
'sDesktop
.
End of Extract (from User Guide)
4.2. Minor Enhancement: Profile Photo
This section contains extracts from the User Guide that are related to profile photos.
Start of Extract (from User Guide)
Changing your profile photo : changepic
(Since v1.2)
Changes the photo displayed on your profile to the photo specified by the path.
Format: changepic PHOTO_PATH
Aliases: pic
, profilepic
Profile photos are displayed with a 4:3 height to width ratio in Club Connect. |
It may take longer to set your profile photo to an image whose file size is above 1 MB. |
To get the absolute path to the photo, see Absolute path of a file. |
Examples:
-
changepic C:/Users/John Doe/Desktop/john_doe.jpg
Changes your profile picture to the "john_doe.jpg" image onJohn Doe
'sDesktop
. -
changepic C:/Users/Admin/Downloads/CathyRay.png
Changes your profile picture to the "CathyRay.png" image in yourDownloads
folder.
Figure 11 shows the output of the changepic
command.
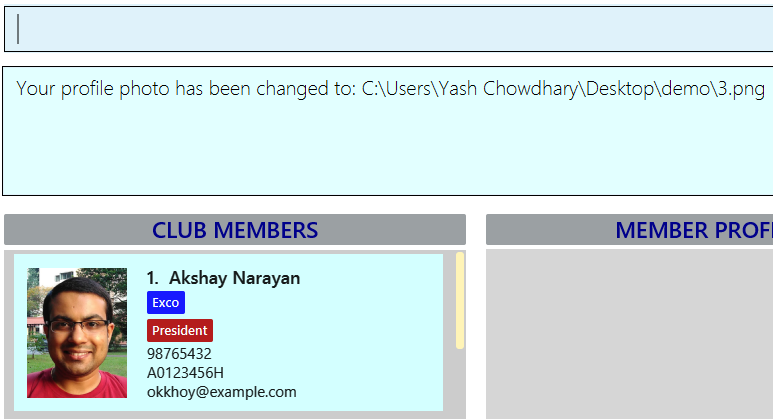
Removing your profile photo : removepic
(Since v1.5)
Removes your profile photo and sets it back to Club Connect’s default profile photo.
Format: removepic
Aliases: rmpic
, defaultpic
, delpic
This command cannot be undone. You will have to set your profile photo by using the changepic command again.
|
If you have not set a profile photo, your profile photo will still be the default photo. |
End of Extract (from User Guide)
5. Contributions to the Developer Guide
Given below are sections that I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
5.1. Major Enhancement: Member Data Management
This section contains an extract from the Developer Guide that is related to management of members' data.
Start of Extract (from Developer Guide)
Importing Members
Current Implementation
The import
mechanism of Club Connect is facilitated by the ImportCommand
class and is event-driven.
It allows members to add the list of members from a CSV file to Club Connect at one go.
To facilitate this, it makes use of the CsvUtil
class, which converts Member
objects to CSV format.
The ImportCommand
extends from the UndoableCommand
class, and only consists of a File
attribute.
This is illustrated in the UML diagram shown in Figure 61.
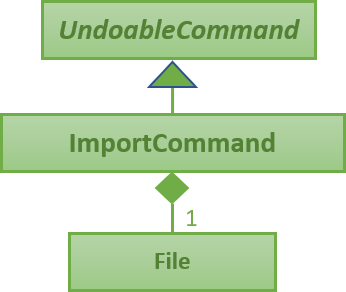
Figure 61. ImportCommand UML Diagram
The import
command involves the use of multiple components of the Club Connect application.
Figure 62 (below) shows the high-level Sequence Diagram for the import
command.
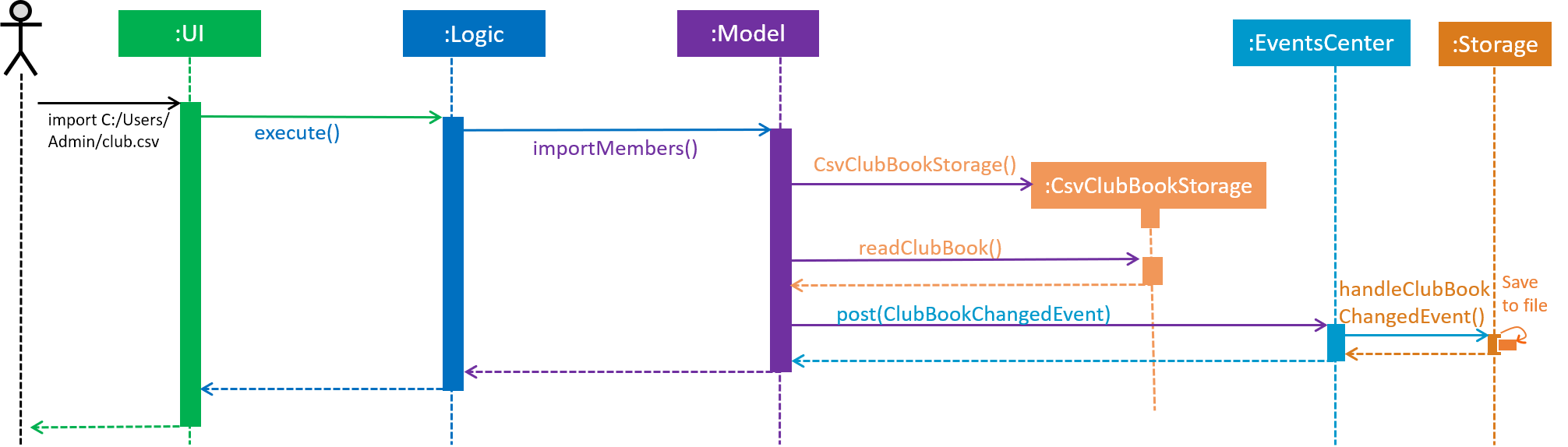
Figure 62. High Level Sequence Diagram for the import C:/Users/Admin/club.csv
command
Logic
is responsible for parsing the import
command.
It returns an ImportCommand
object after parsing the CSV file path.
The ImportCommand#execute()
method invokes the importMembers()
method from model
.
The Model
component invokes the readClubBook()
method of the CsvClubBookStorage
object to get the list of Member
objects that are to be added to Club Connect. The members from the list are then sequentially added to the club book.
If a DuplicateMatricNumberException
is encountered while adding a member, then that member is not added to the club book
and execution continues. This way, even if there are a few members with invalid data values, the import command will
still work for those members with valid data. This implementation is shown in the code snipped below:
public int importMembers(File importFile) throws IOException {
CsvClubBookStorage csvStorage = new CsvClubBookStorage(importFile);
UniqueMemberList importedMembers = csvStorage.readClubBook();
int numberMembers = 0;
for (Member member: importedMembers) {
try {
clubBook.addMember(member);
numberMembers++;
} catch (DuplicateMatricNumberException dmne) {
//...logging...
}
}
indicateClubBookChanged();
return numberMembers;
}
The conversion of data from the CSV file to Member
objects is facilitated by the CsvUtil
class. Part of the implementation is shown here:
public static UniqueMemberList getDataFromFile(File file) throws IOException {
UniqueMemberList importedMembers = new UniqueMemberList();
String data = FileUtil.readFromFile(file);
String[] membersData = data.split("\n");
for (int i = 1; i < membersData.length; i++) { //membersData[0] contains column headers
try {
Member member = getMember(membersData[i]);
importedMembers.add(member);
} catch (Exception e) {
//...logging...
}
}
return importedMembers;
}
Design Considerations
Aspect: Implementation of ImportCommand
-
Alternative 1 (current choice): Implement parser for CSV files ourselves
-
Pros: Greater control over what the format in the file to be imported from should be
-
Cons: Harder to implement, less flexible in terms of how data can be stored in the file as the data values are not binded to tags
-
-
Alternative 2: Use existing libraries (such as OpenCSV and Jackson)
-
Pros: More flexible in terms of how the data can be stored in the import file
-
Cons: Security issues or bugs in the external library cannot be fixed, licensing issues, difficult to use due to poor documentation
-
Aspect: Input file format
-
Alternative 1 (current choice): Import from a CSV file
-
Pros: Easier for users to create and modify their data in a CSV file
-
Cons: Implementation becomes more complex and may become less robust as support for parsing data from CSV files has to be added.
-
-
Alternative 2: Import from a XML file
-
Pros: Easier to implement and parse data, as the code already supports the reading of data from XML format
-
Cons: Harder for non-technical users to create and modify their data in a XML file
-
End of Extract (from Developer Guide)
5.2. Minor Enhancement: Changing Profile Photo
This section contains an extract from the Developer Guide that is related to changing your profile photo.
Start of Extract (from Developer Guide)
Changing Profile Photo Mechanism
Current Implementation
The changepic
mechanism of Club Connect is facilitated by the ChangeProfilePhotoCommand
class and is event-driven.
It allows members to modify their profile photos displayed in the application.
To facilitate this, it makes use of the ProfilePhoto
class.
Currently, the ChangeProfilePhotoCommand
extends from the Command
class, and not from UndoableCommand
.
Refer to Figure 66 for the UML diagram.
The ProfilePhoto
class consists of a String
attribute to store the file path of the profile photo.
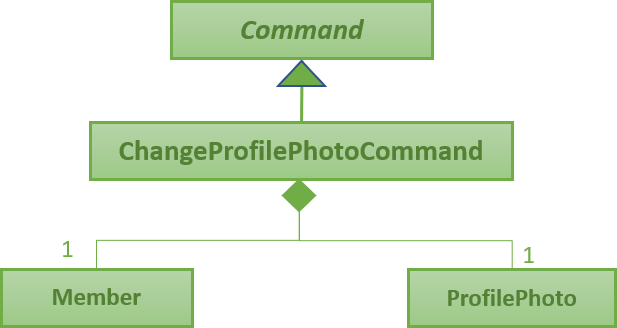
Figure 66. ChangeProfilePhotoCommand UML Diagram
The displaypic
command involves the use of multiple components of Club Connect.
Figure 67 (below) shows the interactions between these components.
As you can see, the ChangeProfilePhotoCommand
is driven by the ProfilePhotoChangedEvent
.
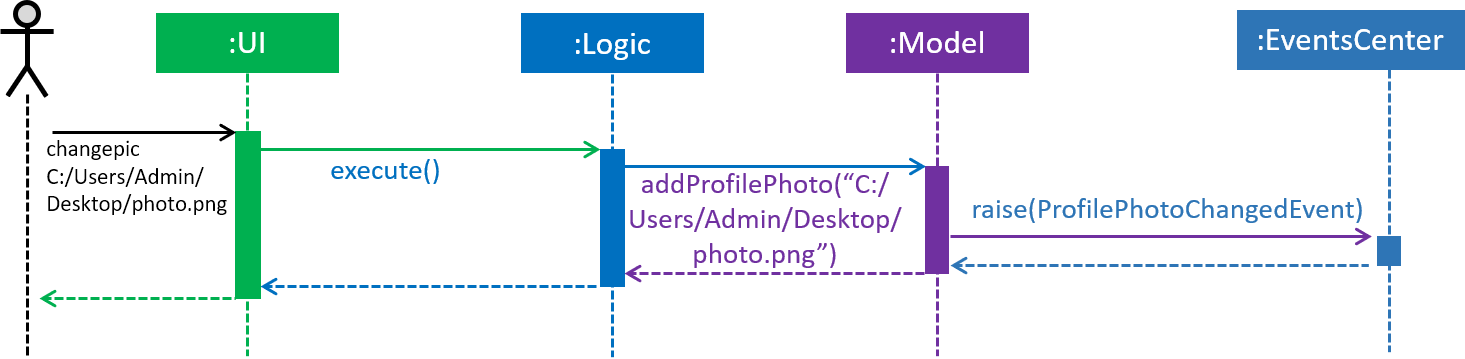
Figure 67. High Level Sequence Diagram for the changepic C:/Users/Admin/Desktop/photo.png
command
ChangeProfilePhotoCommandParser is responsible for parsing the changepic
command.
It returns a ChangeProfilePhotoCommand
object after parsing the photo file path.
Figure 68 depicts the Sequence Diagram for interactions within the Logic
component for the execute("changepic C:/Users/Admin/Desktop/ photo.png")
API call.
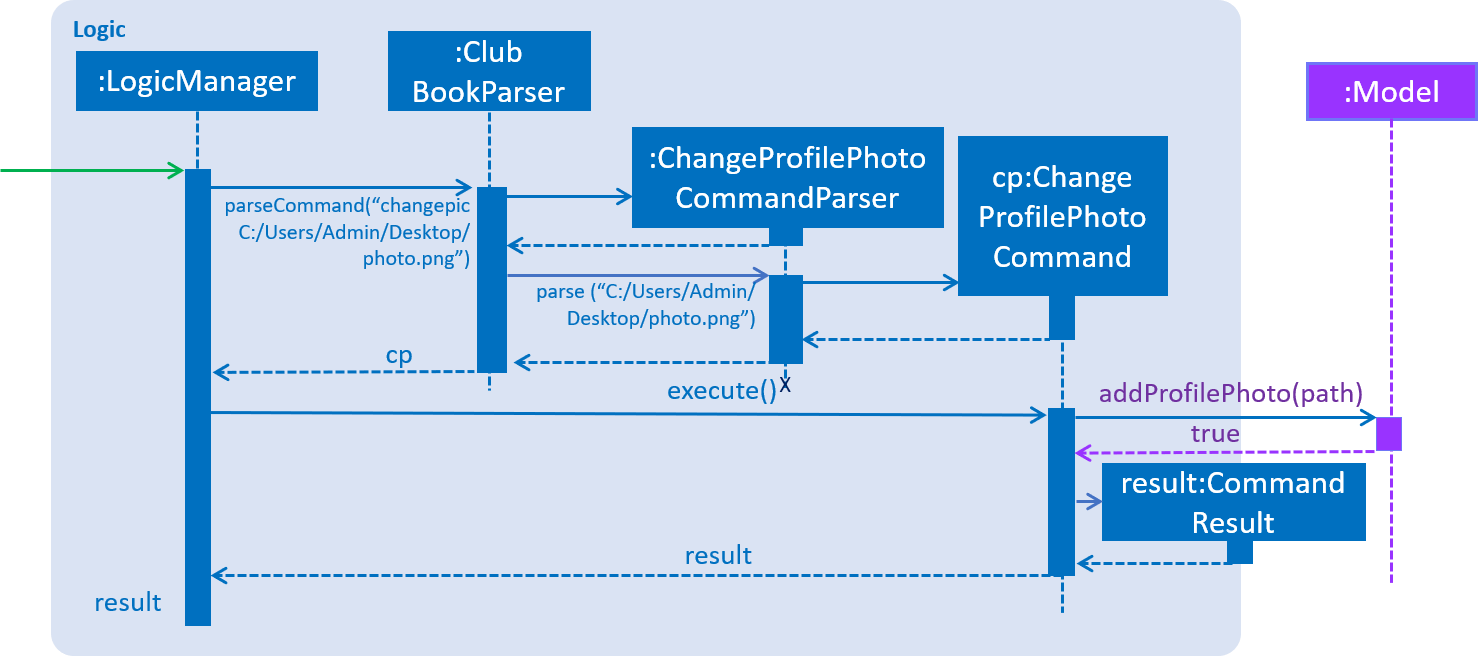
_Figure 68. Interactions Inside the Logic Component for the changepic C:/Users/Admin/Desktop/photo.png
command
The ChangeProfilePhotoCommand#execute()
method invokes the addProfilePhoto()
method from model
.
Complying with the rules of abstraction, the Logic
component calls on Model
to handle the internal details for updation.
The actual reading of the profile photo from the path provided is done by the Storage
component.
It copies the photo as a bitmap image file (.bmp) to the Club Connect application’s resources.
The code used for reading and copying the file is as follows:
@Override
public void copyOriginalPhotoFile(String originalPhotoPath, String newPhotoName) throws PhotoException {
BufferedImage originalPhoto = null;
try {
logger.info("Profile Photo is being read from " + originalPhotoPath);
URL photoUrl = new URL(URL_PREFIX + originalPhotoPath);
newPath = SAVE_PHOTO_DIRECTORY + newPhotoName + PHOTO_FILE_EXTENSION;
InputStream photoStream = photoUrl.openStream();
createPhotoFileCopy(photoStream, newPath);
} catch (IOException ioe) {
// ... exception handling ...
}
}
public void createPhotoFileCopy(InputStream photoStream, String newPath) throws PhotoWriteException {
// ... logging ...
try {
FileUtil.createDirs(new File(SAVE_PHOTO_DIRECTORY));
Files.copy(photoStream, Paths.get(newPath), StandardCopyOption.REPLACE_EXISTING);
} catch (IOException ioe) {
// ... exception handling ...
}
}
The logged in member’s details are then updated to include this new profile photo.
The photo specified by the path is set to the ImageView
object by the following code in the Ui
component:
private ImageView profilePhoto;
private void setProfilePhoto(Member member) {
Image photo;
String photoPath = member.getProfilePhoto().getPhotoPath();
if (photoPath.equals(EMPTY_STRING) || photoPath.equals(DEFAULT_PHOTO_PATH)) {
photo = new Image(MainApp.class.getResourceAsStream(DEFAULT_PHOTO_PATH), photoWidth, photoHeight, false, true);
} else {
photo = new Image("file:" + photoPath, photoWidth, photoHeight, false, true);
}
profilePhoto.setImage(photo);
}
The actual displaying of the profile photo is done by using this fxml code:
<HBox alignment="CENTER_LEFT">
<ImageView fx:id="profilePhoto" fitWidth="100" fitHeight="130">
<HBox.margin>
<Insets left="7.5" bottom="7.5" right="5.0" top="7.5" />
</HBox.margin>
</ImageView>
</HBox>
Design Considerations
Aspect: Implementation of ChangeProfilePhotoCommand
-
Alternative 1 (current choice): Logged in member can only change his/her own profile photo
-
Pros: Easy to implement, makes intuitive sense
-
Cons: There is no way for Exco members to ensure that members have appropriate profile photos
-
-
Alternative 2: Exco members can change any member’s profile photo
-
Pros: Exco members have the ability to exercise control over members' profile photos
-
Cons: Implementation becomes more complicated
-
Aspect: Source files of profile photos
-
Alternative 1 (current choice): Make a copy the source image provided to the applications resources
-
Pros: Application becomes portable and independent from the rest of the system. Members can delete the original file from the computer, without affecting the Club Connect Application
-
Cons: Changes made to the original source images are not reflected in the application
-
-
Alternative 2: Always read the profile photo from the file path provided
-
Pros: Changes made in the source image are reflected in the application
-
Cons: Application becomes highly dependent on the system, in terms of profile photos
-
End of Extract (from Developer Guide)
Appendix A: Other Sections in the User Guide
Given below are other sections that I contributed to the User Guide. |
A.1. Delete tag feature
Start of Extract (from User Guide)
Deleting a tag : deletetag
(Since v1.1)
Deletes the specified tag from all members in Club Connect.
Format: deletetag t/TAG
Aliases: rmtag
, deltag
This command is for Exco members only.
|
Examples:
-
deletetag t/Treasurer
Deletes theTreasurer
tag for all members tagged withTreasurer
in Club Connect. -
deletetag t/EventInCharge
Deletes theEventInCharge
tag for all members tagged withEventInCharge
in Club Connect.
Figure 19 shows the output of the deletetag
command.
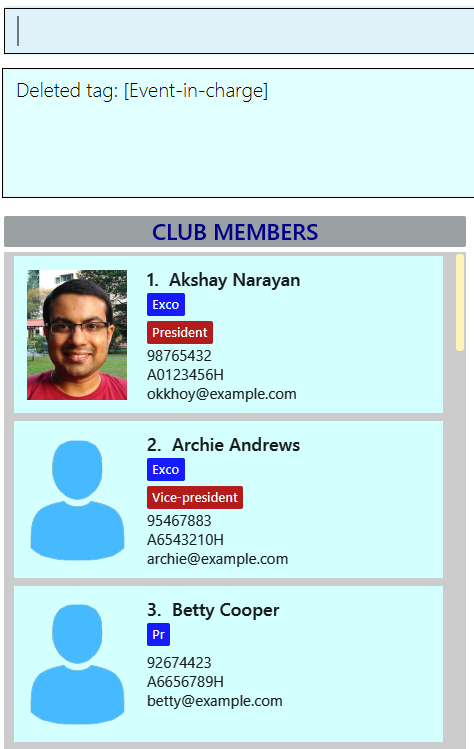
End of Extract (from User Guide)
A.2. General additions
Start of Extract (from User Guide)
Here are some things to take note of before you begin using Club Connect.
Valid Entries
-
Names of members should only contain alphabets, numbers and spaces. The name should not be blank and cannot begin with a space (" ").
-
Phone numbers can only contain numbers, and should be at least 3 digits long.
-
Email IDs of members should be of the format: username@emailservice.com and should not contain spaces.
-
Matriculation Numbers should follow the format of those at NUS. So, they must begin with a letter, followed by 7 digits and should end with a letter.
-
Groups should only contain letters and digits. They must not be empty.
-
Tags should also only contain letters, digits and hyphens. They cannot be empty, and cannot begin with a hyphen ("-").
Tips
Not satisfied with your productivity while using Club Connect? Can’t remember the command names? Here are some tips and tricks:
-
Data transfer to another computer
-
Install the Club Connect app on the other computer.
-
Overwrite the empty data file it creates (
clubbook.xml
) with the data file from your previousClub Connect
folder.
-
-
Alternative command names
If you do not like the default command name or feel that it is too long, you can use one of its aliases to execute the command instead.Example: The
changepic
command usespic
as an alias. So, both commands shown below can be used change your profile picture to the "john_doe.jpg" image on John Doe’s Desktop.
changepic C:/Users/John Doe/Desktop/john_doe.jpg
pic C:/Users/John Doe/Desktop/john_doe.jpg
-
Absolute path of a file
To get the absolute path of a file on Windows, follow these steps:-
Right-click on the file and select
Properties
. This will bring up a window containing the properties of the file. -
Locate the path in the
General
section, next to the keywprdLocation
. -
Add the complete name of the file (e.g. "/file_name.jpg") to the end of this location to get the absolute path to the file.
To get the absolute path of a file on MacOS, follow these steps:
-
Select the file and press Command+I to open the information window for it.
-
Locate the path in the
General
section, next to the keywordWhere
. -
Add the complete name of the file (e.g. "/file_name.jpg") to the end of this location to get the absolute path to the file.
-
-
CSV format for
import
In order to successfully import data of members from the specified file, it has to follow the format shown in Figure 28.Figure 4. Required format of data in the fileThe columns in the import file should be in the same order as shown in the figure above. All the tags of a member should be in a single cell, separated by commas (","). Also, to successfully import the data of a member, you have to make sure that their details conform to the constraints. Figure 29 shows some examples of invalid entries with the corresponding reasons in red.
Figure 5. Invalid format of data in the file (reasons are shown in red) -
Member Profile
In order for the member profile to look as intended when a member is selected using theselect
command, ensure that:-
The name of selected member does not exceed the width of the panel.
-
The tags of selected member can be displayed in one line.
-
Coming in v2.0
-
Encrypt data files :
encrypt
Encryption is the process of encoding information in such a way that only authorized parties can access it and others cannot.
By encrypting Club Connect’s data files, you can ensure that others will not be able to read members' information if they open the files. Do note, however, that this may slightly affect performance. -
Chat with any member :
chat INDEX
You can message other members in real time without ever needing to leave the Club Connect application. -
Group Chats :
gchat GROUP_NAME
Tired of sending the same message to multiple members? The Group Chat feature allows you to have conversations as a group so that everyone is kept in the loop. -
Submit anonymous feedback :
feedback
Not satisfied with certain aspects of the club? Afraid to speak up?
Fret not, Club Connect provides you with a platform to voice your opinions. And yes, we guarantee your anonymity.
End of Extract (from User Guide)
Appendix B: Other Sections in the Developer Guide
Given below are other sections that I contributed to the Developer Guide. |
Start of Extract (from Developer Guide)
Exporting Members
Current Implementation
The export
mechanism of Club Connect is facilitated by the ExportCommand
class and is event-driven.
It allows members to export the list of members in Club Connect as a CSV file.
It makes use of the CsvUtil
class to convert Member
objects to CSV format.
The ExportCommand
extends from the Command
class, as it is not an undoable command and only consists of a File
attribute.
Figure 63 depicts the UML diagram for the ExportCommand
class.
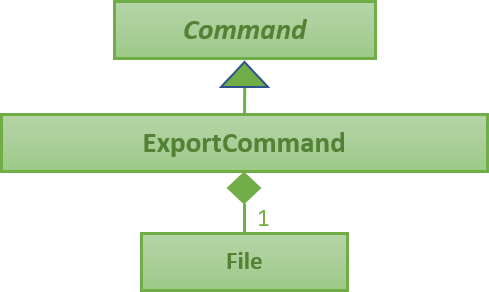
Figure 63. ExportCommand UML Diagram
The export
command uses multiple components of the Club Connect application.
The Sequence Diagram below (Figure 64) shows the interactions between some of these components.
As you can see, the ExportCommand
is driven by the NewExportDataAvailableEvent
.
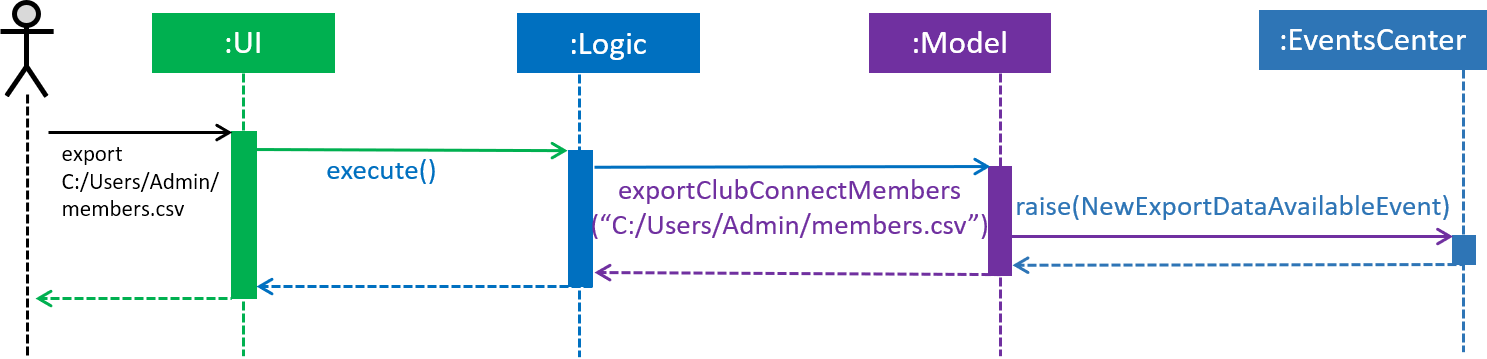
Figure 64. High Level Sequence Diagram for the export C:/Users/Admin/members.csv
command
This event is handled by the Storage
component as shown in Figure 65.
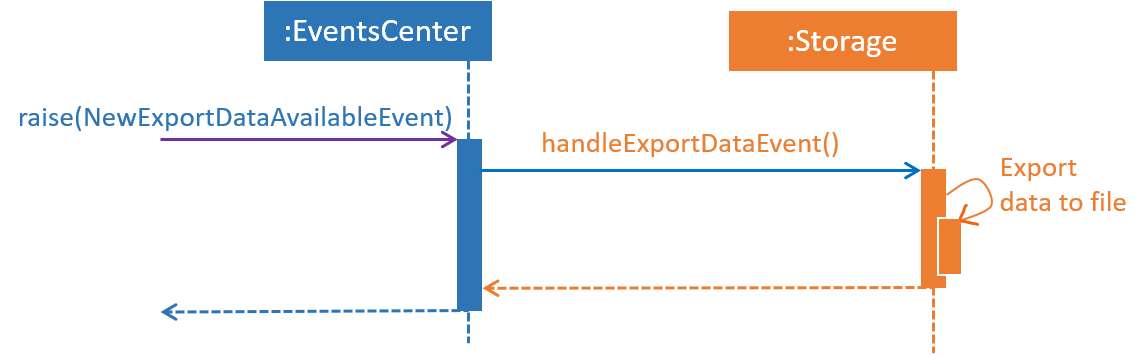
_Figure 65. High Level Sequence Diagram showing how the Storage
component handles the NewExportDataAvailableEvent
Storage
makes use of a CsvClubBookStorage
object to write data to the file specified by the export path.
This is shown in the code snippet below:
public void exportDataToFile(String data, File exportFile) throws IOException {
csvClubBookStorage.setClubBookFile(exportFile);
// ... logging ...
csvClubBookStorage.saveData(data);
}
Then, the csvClubBookStorage
object gets the column headers for the file from the CsvUtil
class:
public void saveData(String data, File file) throws IOException {
// ... logging ...
String columnHeaders = CsvUtil.getHeaders();
String dataToExport = columnHeaders + data;
CsvFileStorage.saveDataToFile(file, dataToExport);
}
Finally, the CsvFileStorage
class saves the data to the file by using the writeToFile
method from the FileUtil
class.
public static void saveDataToFile(File file, String data) throws IOException {
FileUtil.writeToFile(file, data);
}
Design Considerations
Aspect: Output file format
-
Alternative 1 (current choice): Export to a CSV file
-
Pros: More user-friendly in terms of understandability, generated file is compatible with the
import
command -
Cons: Harder to implement as support for converting data of
Member
to CSV format needs to be added
-
-
Alternative 2: Export to a XML file
-
Pros: Easier to implement and as the code already supports the writing of data in XML format
-
Cons: Raw file provides a less intuitive view of data, generated file is not compatible with the
import
command
-
Glossary
- Abstraction
-
In Object-oriented Programming, abstraction is the mechanism by which users are provided with only the functionality, and not the implementation details. So, abstraction provides users with information on what an object does, rather than how it does it.
- Attribute
-
An attribute is a type of detail of a member. For example, an attribute of a member could be phone number, email, matriculation number and so on.
- Bitmap Image File
-
Bitmap Image file (BMP) is a file format that stores bitmap graphics data. It is device independent and you do not need a graphics adapter to display it. Images stored as BMP files can be losslessly compressed.
- Club
-
A student organisation or association at the National University of Singapore. These include (but are not limited to) Faculty/Non-Faculty clubs, Academic/Non-Academic Societies, Interest Groups and Sports groups. Some examples include Computing Club, Mathematics Society and Basketball Varsity Team.
- Club Book
-
The internal database of Club Connect that stores member, task and poll information.
- CLI
-
Acronym for Command Line Interface. It is a purely text-based interface for software. User respond to visual prompts by typing single commands into the interface and receive results as text as well. An example of CLI would be MS-DOS.
- CSV
-
A comma-separated values (CSV) file is a text file that uses a comma (",") to separate values. This allows data to be saved in a table structured format.
- Entry
-
A value added to a member’s attribute.
- Exco member
-
A member who is part of the Executive Committee of the club. Exco members are seen as the leaders of the club. Exco members can execute certain commands and view features that are not available to other members of the club.
An Exco member is still regarded as a member. |
- GUI
-
Acronnym for Graphical User Interface. In a GUI, the software interface consists of graphical icons, menus and/or other visual indicators to display information. Users can typically interact with these graphics, rather than just using text in the command line. For example, all Windows operating systems have a GUI.
- Mainstream OS
-
Windows, Linux, Unix, OS-X
- Member
-
One of the members who compose a group organization. They are the target users of our application.
- Private contact detail
-
A contact detail that is not meant to be shared with others
- Profile
-
Visual display of the information (attributes and entries) of a member.
End of Extract (from Developer Guide)