By: Team W15-B4
Since: Jun 2016
Licence: MIT
- 1. Introduction
- 2. Setting up
- 3. Design
- 4. Implementation
- 4.1. Undo/Redo feature
- 4.2. Account Management
- 4.3. Email Mechanism
- 4.4. Task-Management
- 4.5. Polls-feature
- 4.6. Delete Group Mechanism
- 4.7. Importing Members
- 4.8. Exporting Members
- 4.9. Changing Profile Photo Mechanism
- 4.10. Compress/Decompress Mechanism
- 4.11. Auto-Complete Command Mechanism
- 4.12. [Proposed] Data Encryption
- 4.13. Logging
- 4.14. Configuration
- 5. Documentation
- 6. Testing
- 7. Dev Ops
- Appendix A: Suggested Programming Tasks to Get Started
- Appendix B: Product Scope
- Appendix C: User Stories
- Appendix D: Use Cases
- Appendix E: Non Functional Requirements
- Appendix F: Glossary
- Appendix G: Instructions for Manual Testing
- G.1. Launch and Shutdown
- G.2. Deleting a member
- G.3. Adding a task
- G.4. Assigning a task
- G.5. Deleting a task
- G.6. Changing a task’s status
- G.7. Changing a task’s assignee
- G.8. Deleting a group
- G.9. Adding a poll
- G.10. Deleting a poll
- G.11. Voting in a poll
- G.12. Viewing results of polls
- G.13. Hiding results of polls
- G.14. Compressing member cards
- G.15. Decompressing member cards
- G.16. Finding members
- G.17. Importing members
- G.18. Exporting members
- G.19. Changing profile photo
- G.20. Removing profile photo
- G.21. Deleting a tag
1. Introduction
Club Connect is a computer application targeted at student club members at the National University of Singapore (NUS). It aims to make the tedious process of club management easier are more effective. It provides features that are essential for a club to function smoothly such as user authentication, assigning tasks, email blasts, conducting polls, and importing and exporting member details.
This guide provides the necessary information for developers who are interested in contributing to the application. If you are a novice developer, it is recommended that you read through this guide sequentially. The guide will thoroughly explain all aspects of this project- from the steps required to set up the project on your computer, to the implementation details of some features of this application.
To familiarise yourself with the source code it is recommended that you try out the tasks in Appendix A, Suggested Programming Tasks to Get Started. If you are an experienced developer, you may wish to jump to Section 3.1, “Architecture” to begin understanding the software architecture.
2. Setting up
The steps that you need to follow to set up the Club Connect project on your computer are listed below.
2.1. Prerequisites
Install the following on your computer before you begin the project setup:
-
JDK
1.8.0_60
or laterHaving any Java 8 version is not enough.
This app will not work with earlier versions of Java 8. -
IntelliJ IDE
IntelliJ by default has Gradle and JavaFx plugins installed.
Do not disable them. If you have disabled them, go toFile
>Settings
>Plugins
to re-enable them.
2.2. Setting up the project in your computer
Follow these steps to set up the project on your computer:
-
Fork this repo, and clone the fork to your computer
-
Open IntelliJ (if you are not in the welcome screen, click
File
>Close Project
to close the existing project dialog first) -
Set up the correct JDK version for Gradle
-
Click
Configure
>Project Defaults
>Project Structure
-
Click
New…
and find the directory of the JDK
-
-
Click
Import Project
-
Locate the
build.gradle
file and select it. ClickOK
-
Click
Open as Project
-
Click
OK
to accept the default settings -
Open a console and run the command
gradlew processResources
(Mac/Linux:./gradlew processResources
). It should finish with theBUILD SUCCESSFUL
message.
This will generate all resources required by the application and tests.
2.3. Verifying the setup
Perform the following steps to verify that the project has been set up properly:
-
Run the
seedu.club.MainApp
and try a few commands -
Run the tests to ensure they all pass.
2.4. Configurations to do before writing code
Go through these steps before you begin contributing to the project.
2.4.1. Configuring the coding style
This project follows oss-generic coding standards. IntelliJ’s default style is mostly compliant with ours but it uses a different import order from ours. To rectify,
-
Go to
File
>Settings…
(Windows/Linux), orIntelliJ IDEA
>Preferences…
(macOS) -
Select
Editor
>Code Style
>Java
-
Click on the
Imports
tab to set the order-
For
Class count to use import with '*'
andNames count to use static import with '*'
: Set to999
to prevent IntelliJ from contracting the import statements -
For
Import Layout
: The order isimport static all other imports
,import java.*
,import javax.*
,import org.*
,import com.*
,import all other imports
. Add a<blank line>
between eachimport
-
Optionally, you can follow the UsingCheckstyle.adoc document to configure IntelliJ to check style-compliance as you write code.
2.4.2. Updating documentation to match your fork
After forking the repo, links in the documentation will still point to the CS2103JAN2018-W15-B4/main
repo.
If you plan to develop this as a separate product (i.e. instead of contributing to CS2103JAN2018-W15-B4/main
) , you should replace the URL in the variable repoURL
in DeveloperGuide.adoc
and UserGuide.adoc
with the URL of your fork.
2.4.3. Setting up CI
Set up Travis to perform Continuous Integration (CI) for your fork. See UsingTravis.adoc to learn how to set it up.
After setting up Travis, you can optionally set up coverage reporting for your team fork (see UsingCoveralls.adoc).
Coverage reporting could be useful for a team repository that hosts the final version but it is not that useful for your personal fork. |
Optionally, you can set up AppVeyor as a second CI (see UsingAppVeyor.adoc).
Having both Travis and AppVeyor ensures your App works on both Unix-based platforms and Windows-based platforms (Travis is Unix-based and AppVeyor is Windows-based) |
2.4.4. Getting started with coding
When you are ready to start coding:
-
Get some sense of the overall design by reading Section 3.1, “Architecture”.
-
Take a look at Appendix A, Suggested Programming Tasks to Get Started.
3. Design
This section describes the architecture and 6 components that are part of the Club Connect project.
The .pptx files used to create diagrams in this document can be found in the diagrams folder.
To update a diagram, modify the diagram in the .pptx file, select the objects of the diagram, and choose Save as picture .
|
3.1. Architecture
The Architecture Diagram (Refer to Figure 1) explains the high-level design of the App. Given below is a quick overview of each component.
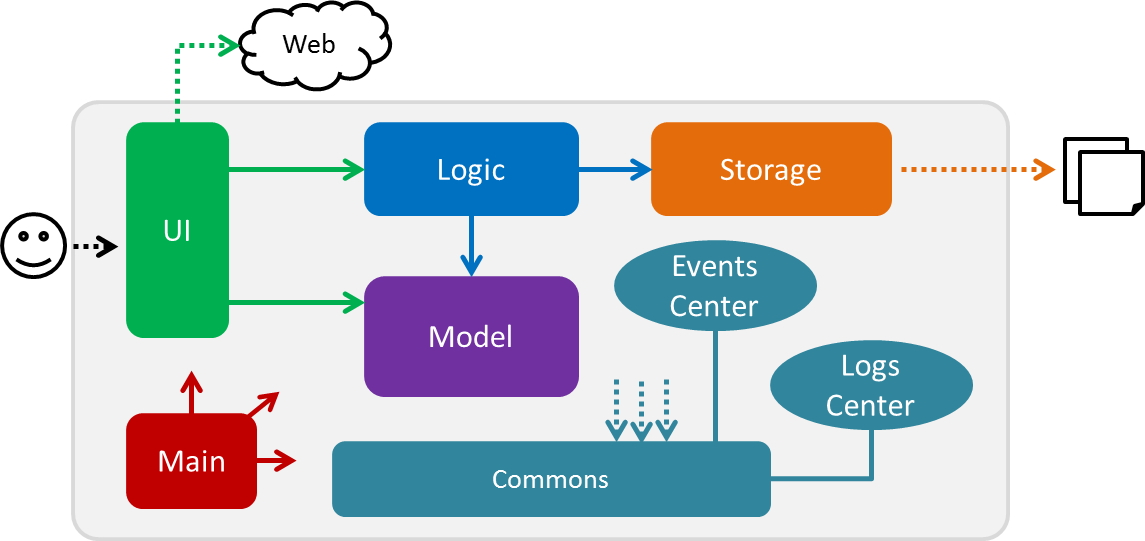
Figure 1. Architecture Diagram
Main
is a component that has only one class called MainApp
.
It is responsible for the following:
-
At app launch: Initializes the components in the correct sequence and connects them up with each other.
-
At shut down: Shuts down the components and invokes cleanup method where necessary.
Commons
represents a collection of classes used by multiple other components.
Two of those classes play important roles at the architecture level. Classes used by multiple components are in the seedu.club.commons
package.
-
EventsCenter
: This class (written using Google’s Event Bus library) is used by a component to communicate with other components using events (i.e. a form of Event Driven design). -
LogsCenter
: Used by many classes to write log messages to the App’s log file.
The rest of the App consists of four components.
Each of the four components:
-
Defines its API in an
interface
with the same name as the Component. -
Exposes its functionality using a
{Component Name}Manager
class.
For example, the Logic
component (Refer to Figure 2) defines it’s API in the Logic.java
interface and exposes its functionality using the LogicManager.java
class.
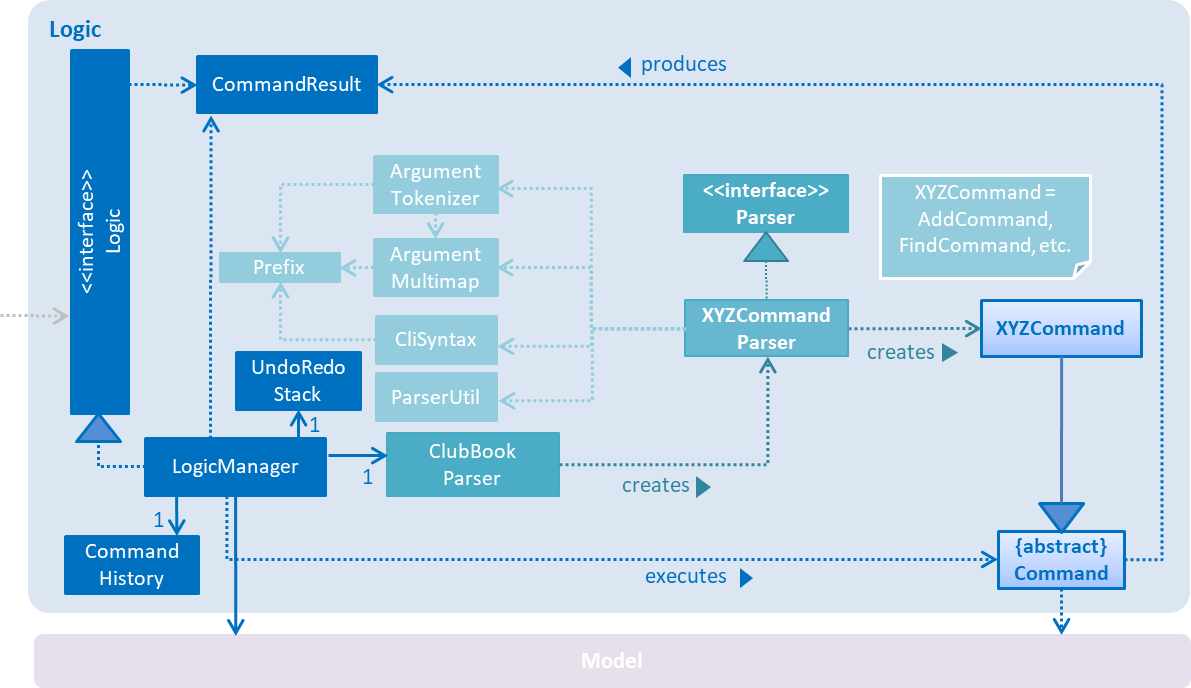
Figure 2. Class Diagram of the Logic Component
Events-Driven nature of the design
The Sequence Diagram below (Figure 3) shows how the components interact in the scenario where the user issues the command delete 1
.
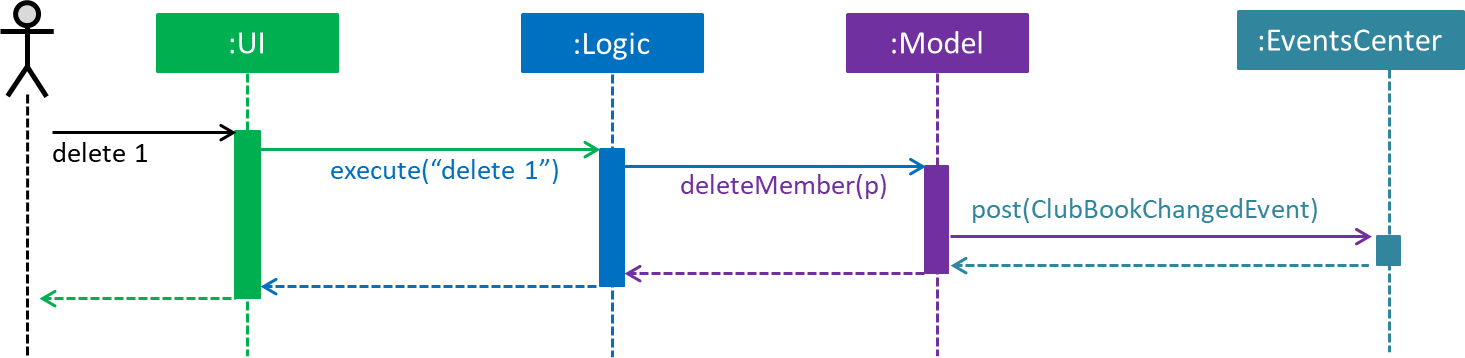
Figure 3. Component interactions for delete 1
command (part 1)
Note how the Model simply raises a ClubBookChangedEvent when the Club Book data is changed, instead of asking the Storage to save the updates to the hard disk.
|
The diagram below (Figure 4) shows how the EventsCenter
reacts to that event, which eventually results in the updates being saved to the hard disk and the status bar of the UI being updated to reflect the 'Last Updated' time.
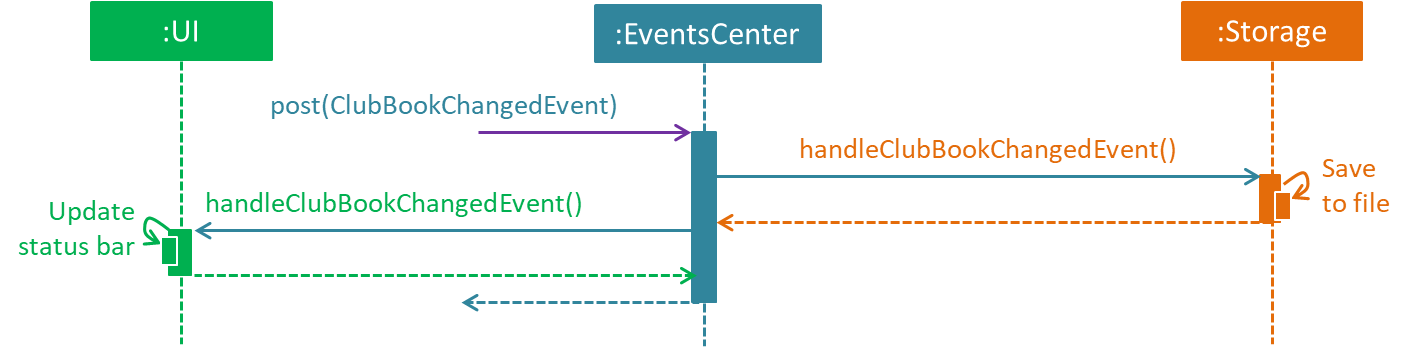
Figure 4. Component interactions for delete 1
command (part 2)
Note how the event is propagated through the EventsCenter to the Storage and UI without Model having to be coupled to either of them.
This is an example of how this Event Driven approach helps us reduce direct coupling between components.
|
The sections below describe the four components in greater detail.
3.2. UI component
API : Ui.java
The UI consists of a MainWindow
that is made up of parts e.g.CommandBox
, ResultDisplay
, MemberListPanel
, StatusBarFooter
, BrowserPanel
etc. All these, including the MainWindow
, inherit from the abstract UiPart
class.
The UI
component uses JavaFx UI framework.
The layouts of these UI parts are defined in matching .fxml
files that are in the src/main/resources/view
folder.
For example, the layout of the MainWindow
is specified in MainWindow.fxml
The UI
component:
-
Executes user commands using the
Logic
component. -
Binds itself to some data in the
Model
so that the UI can auto-update when data in theModel
changes. -
Responds to events raised from various parts of the App and updates the UI accordingly.
Refer to Figure 5 for the structure of the UI component.
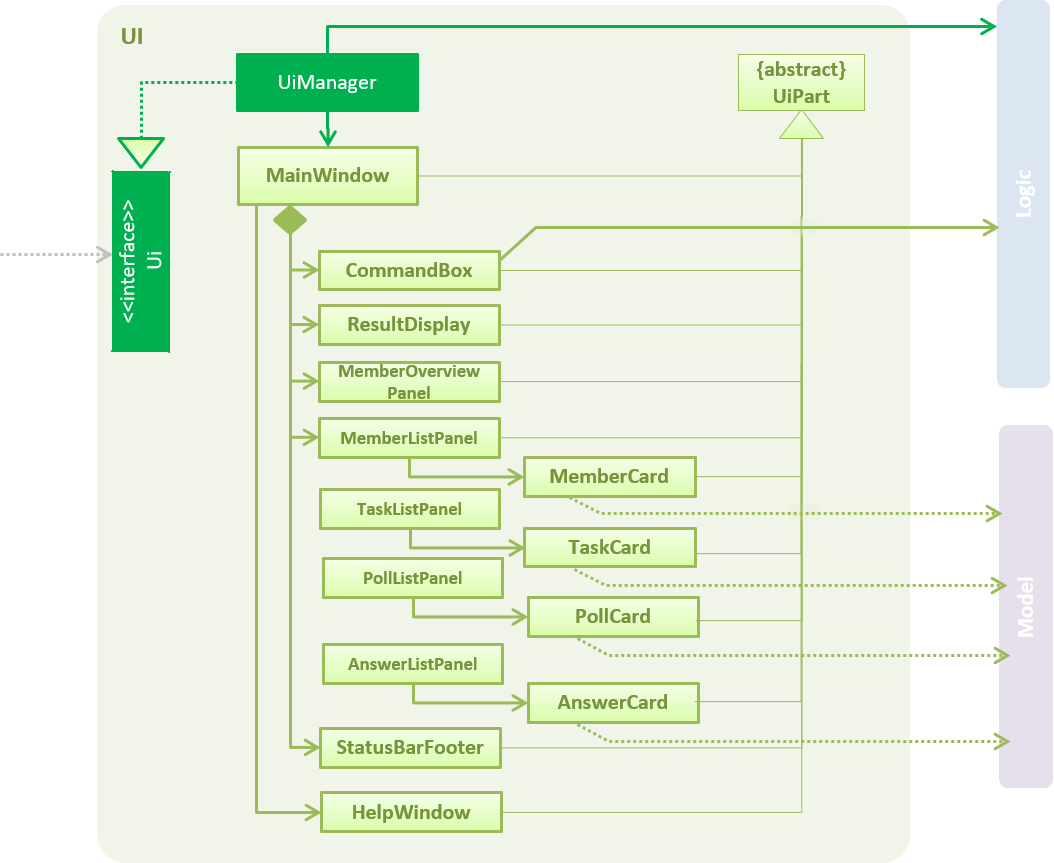
Figure 5. Structure of the UI Component
3.3. Logic component
API :
Logic.java
-
Logic
uses theClubBookParser
class to parse the user command. -
This results in a
Command
object which is executed by theLogicManager
. -
The command execution can affect the
Model
(e.g. adding a member) and/or raise events. -
The result of the command execution is encapsulated as a
CommandResult
object which is passed back to theUi
.
Refer to Figure 6 for the structure of the Logic component.
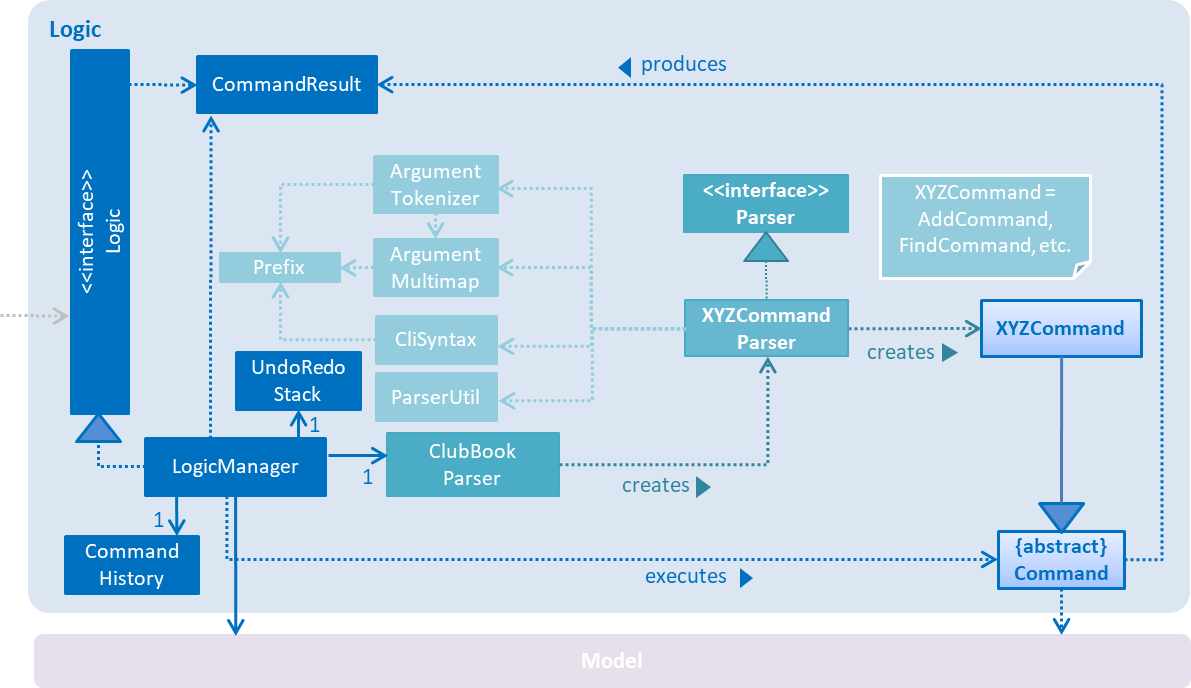
Figure 6. Structure of the Logic Component
Figure 7 below shows finer details concerning XYZCommand
and Command
depicted in Figure 6.
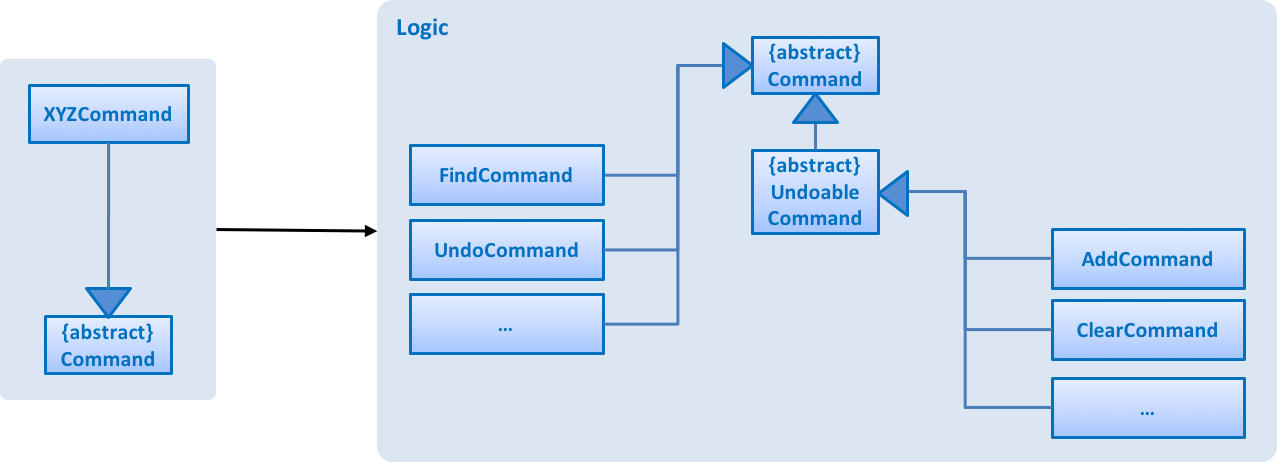
Figure 7. Structure of Commands in the Logic Component.
Given below (Figure 8) is the Sequence Diagram for interactions within the Logic
component for the execute("delete 1")
API call.
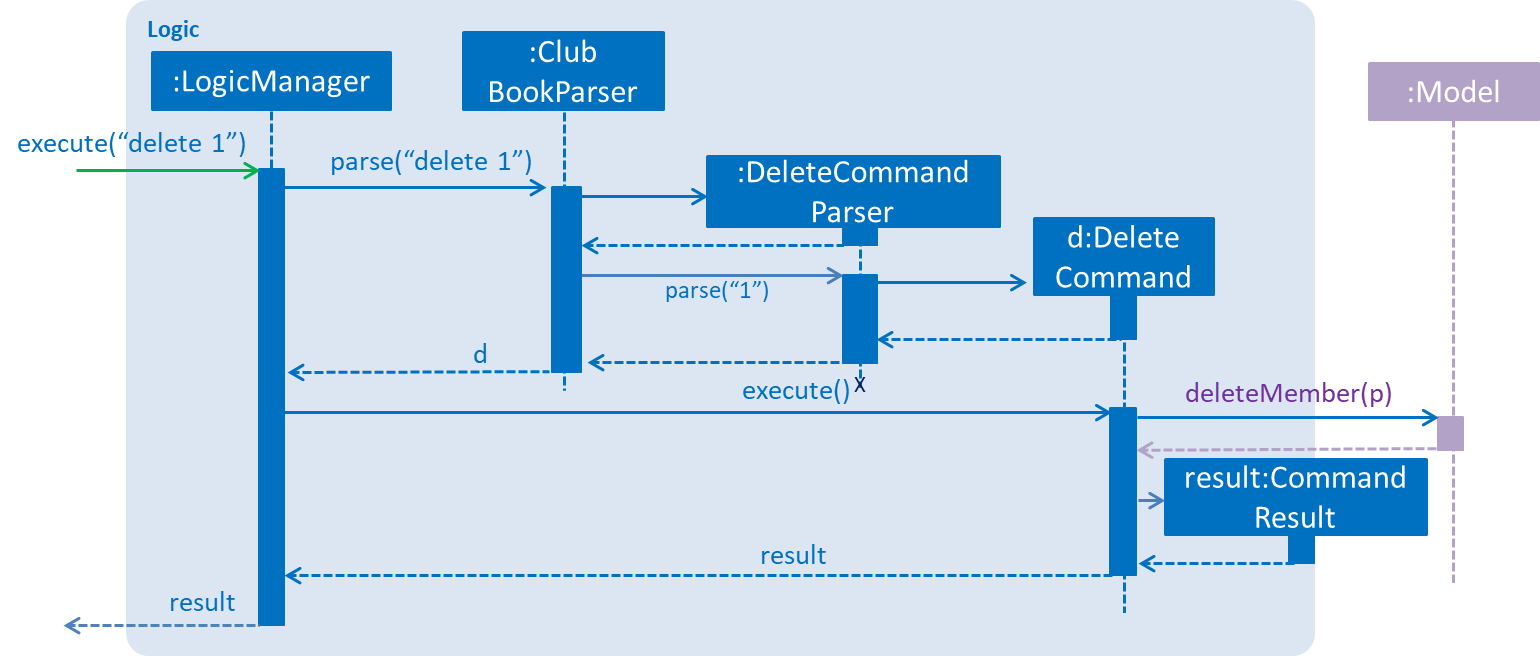
Figure 8. Interactions Inside the Logic Component for the delete 1
Command
3.4. Model component
API : Model.java
The Model
:
-
stores a
UserPref
object that represents the user’s preferences. -
stores the Club Book data.
-
exposes an unmodifiable
ObservableList<Member>
that can be 'observed' For example, the UI can be bound to this list so that the UI automatically updates when the data in the list changes. -
does not depend on any of the other three components.
Refer to Figure 9 for the structure of the Model component.
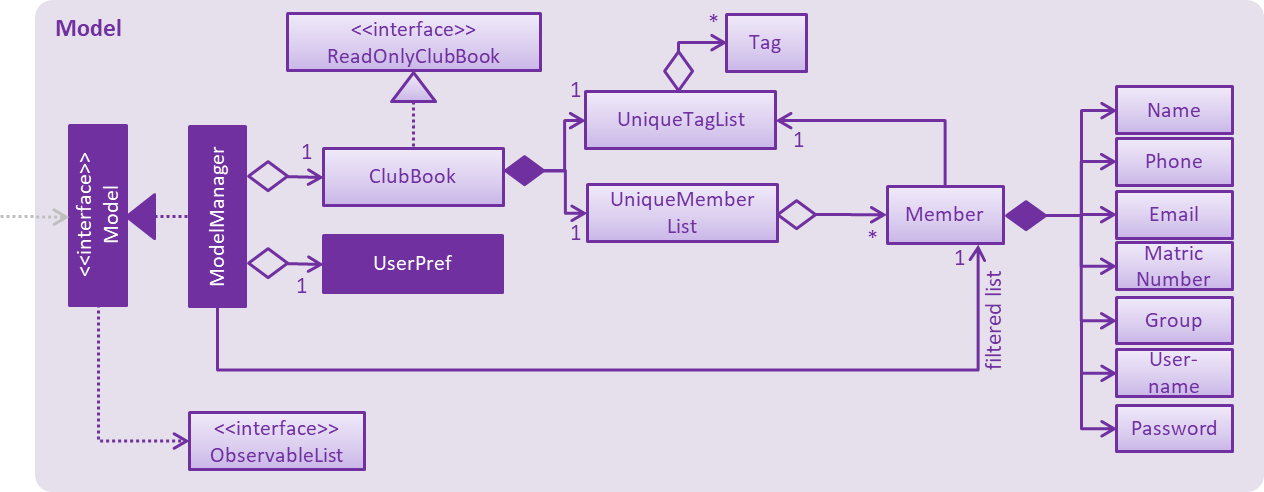
Figure 9. Structure of the Model Component
3.5. Storage component
API : Storage.java
The Storage
component:
-
can save
UserPref
objects in json format and read it back. -
can save the Club Book data in xml format and read it back.
Refer to Figure 10 for the structure of the Storage component.
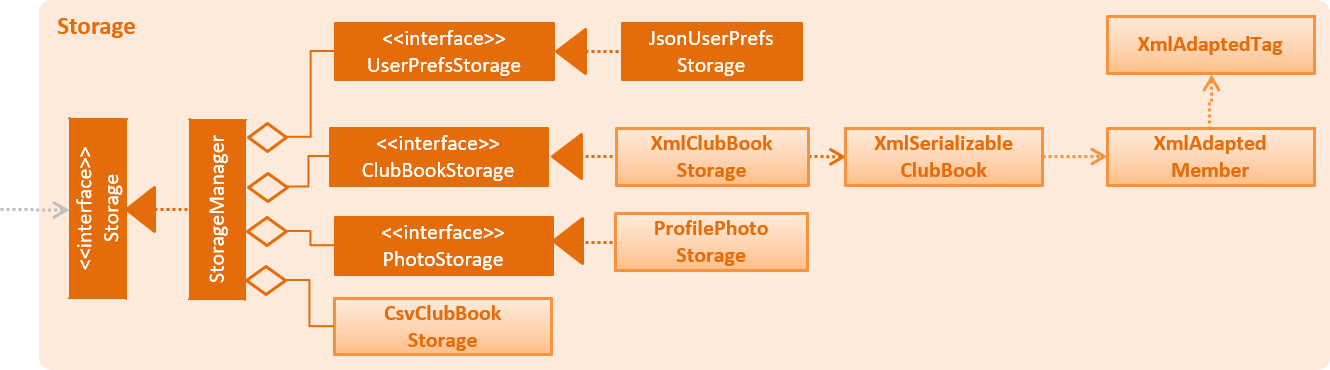
Figure 10. Structure of the Storage Component
4. Implementation
This section describes some noteworthy details on how certain features are implemented.
4.1. Undo/Redo feature
4.1.1. Current Implementation
The undo/redo mechanism is facilitated by an UndoRedoStack
, which resides inside LogicManager
.
It supports undoing and redoing of commands that modifies the state of the club book (e.g. add
, edit
).
Such commands will inherit from UndoableCommand
.
UndoRedoStack
only deals with UndoableCommands
. Commands that cannot be undone will inherit from Command
instead.
The following diagram (Refer to figure 11) shows the inheritance diagram for commands:
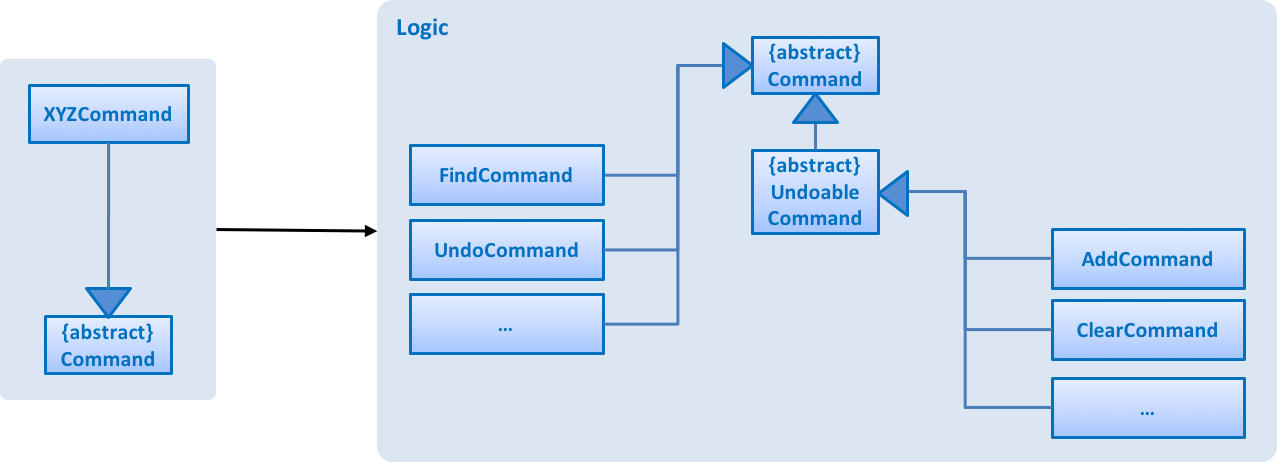
Figure 11. Inheritance Diagram for commands
As you can see from the diagram (Figure 11), UndoableCommand
adds an extra layer between the abstract Command
class and concrete commands that can be undone, such as the DeleteCommand
.
Note that extra tasks need to be done when executing a command in an undoable way, such as saving the state of the club book before execution.
UndoableCommand
contains the high-level algorithm for those extra tasks while the child classes implement the details of how to execute the specific command.
Note that this technique of putting the high-level algorithm in the parent class and lower-level steps of the algorithm in the child classes is also known as the template pattern.
Commands that are not undoable are implemented this way:
public class ListCommand extends Command {
@Override
public CommandResult execute() {
// ... list logic ...
}
}
With the extra layer, the commands that are undoable are implemented this way:
public abstract class UndoableCommand extends Command {
@Override
public CommandResult execute() {
// ... undo logic ...
executeUndoableCommand();
}
}
public class DeleteCommand extends UndoableCommand {
@Override
public CommandResult executeUndoableCommand() {
// ... delete logic ...
}
}
Suppose that the user has just launched the application. The UndoRedoStack
will be empty at the beginning.
The user executes a new UndoableCommand
- delete 5
, to delete the 5th member in the club book.
The current state of the club book is saved before the delete 5
command executes.
The delete 5
command will then be pushed onto the undoStack
(the current state is saved together with the command).
(Refer to Figure 12)

Figure 12. Initial state of undoStack
and `redoStack`
As the user continues to use the program, more commands are added into the undoStack
.
For example, the user may execute add n/David …
to add a new member (Refer to Figure 13).
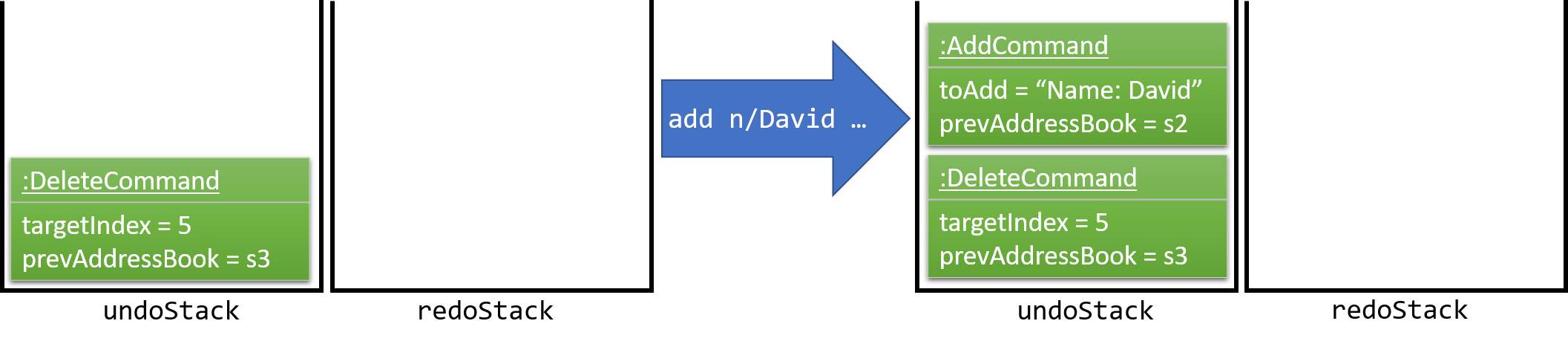
Figure 13. State of undoStack
and redoStack
after executing add
command
If a command fails its execution, it will not be pushed to the UndoRedoStack at all.
|
The user now decides that adding the member was a mistake, and decides to undo that action using undo
.
We will pop the most recent command out of the undoStack
and push it back to the redoStack
.
We will restore the club book to the state before the add
command executed (Refer to figure 14).
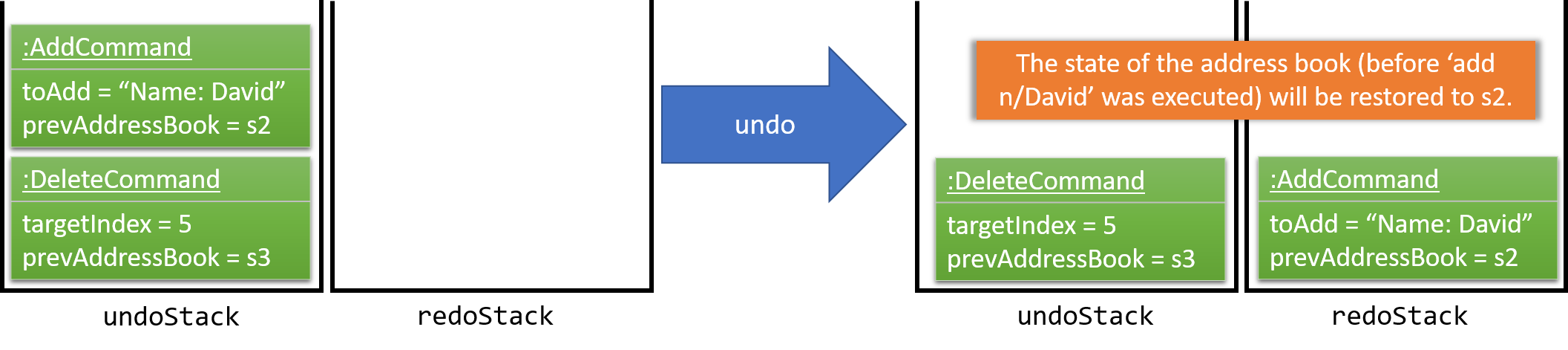
Figure 14. State of undoStack
and redoStack
after executing undo
command.
If the undoStack is empty, then there are no other commands left to be undone, and an Exception will be thrown when popping the undoStack .
|
The following sequence diagram (Figure 15) shows how the undo operation works:
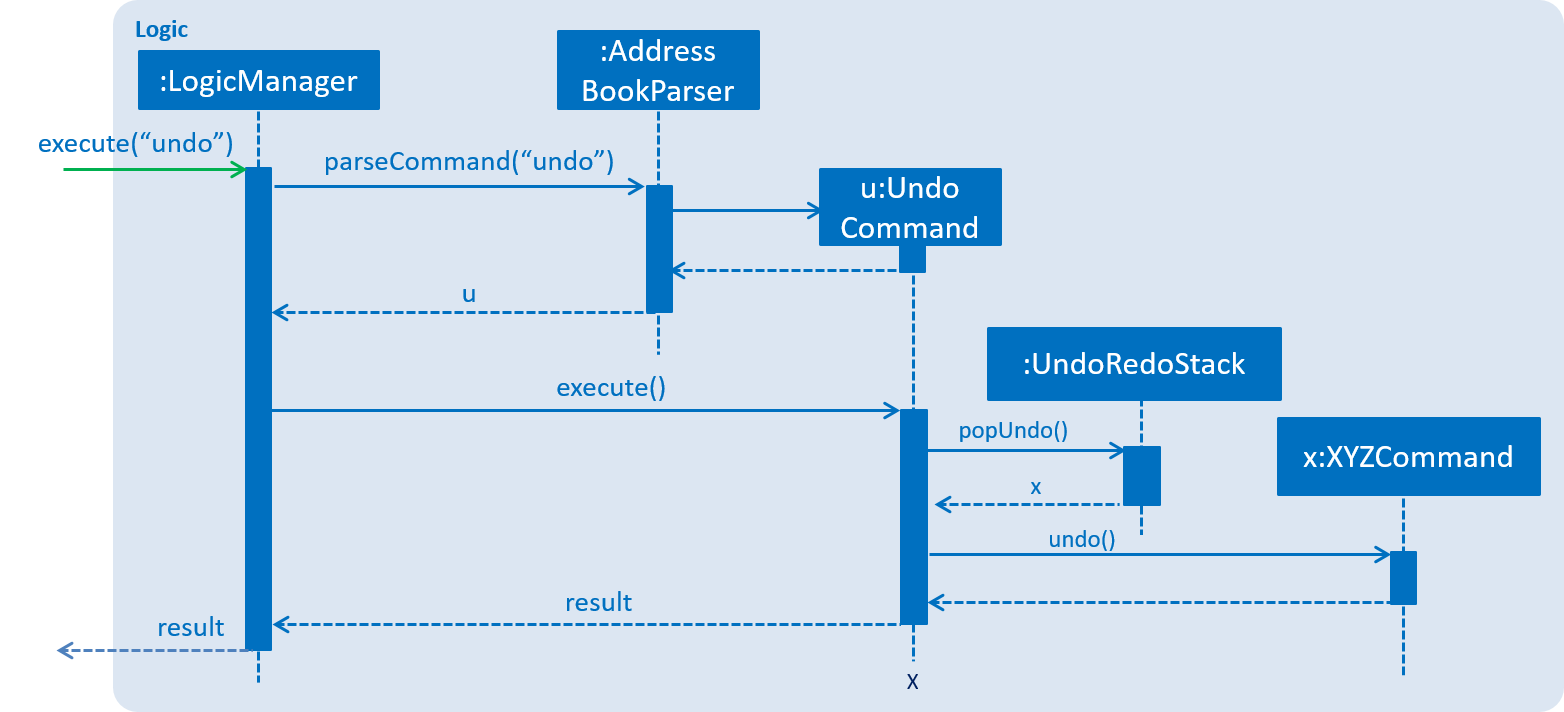
Figure 15. Sequence Diagram of undo
command
The redo does the exact opposite (pops from redoStack
, push to undoStack
, and restores the club book to the state after the command is executed).
If the redoStack is empty, then there are no other commands left to be redone, and an Exception will be thrown when popping the redoStack .
|
The user now decides to execute a new command, clear
. As before, clear
will be pushed into the undoStack
.
This time the redoStack
is no longer empty.
It will be purged as it no longer make sense to redo the add n/David
command (this is the behavior that most modern computer applications follow). (Refer to figure 16)

Figure 16. State of undoStack
and redoStack
after executing clear
command.
Commands that are not undoable are not added into the undoStack
.
For example, list
, which inherits from Command
rather than UndoableCommand
, will not be added after execution (Refer to Figure 17):

Figure 17. State of undoStack
and redoStack
after executing list
command.
The following activity diagram (Figure 18) summarize what happens inside the UndoRedoStack
when a user executes a new command:
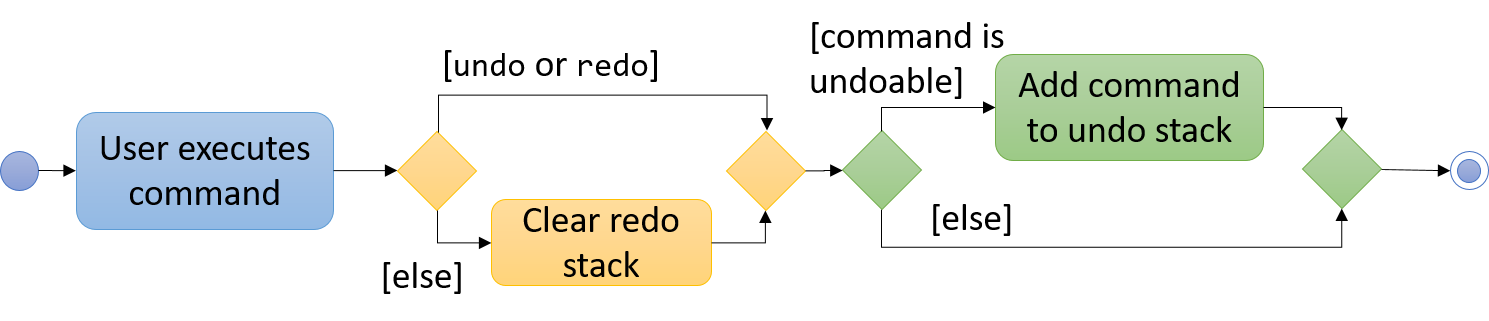
Figure 18. Activity Diagram for Undo-Redo
4.1.2. Design Considerations
Aspect: Implementation of UndoableCommand
-
Alternative 1 (current choice): Add a new abstract method
executeUndoableCommand()
.-
Pros: We will not lose any undone/redone functionality as it is now part of the default behaviour. Classes that deal with
Command
do not have to know thatexecuteUndoableCommand()
exist. -
Cons: Hard for new developers to understand the template pattern.
-
-
Alternative 2: Just override
execute()
.-
Pros: Does not involve the template pattern, easier for new developers to understand.
-
Cons: Classes that inherit from
UndoableCommand
must remember to callsuper.execute()
, or lose the ability to undo/redo.
-
Aspect: How undo & redo executes
-
Alternative 1 (current choice): Saves the entire address book.
-
Pros: Easy to implement.
-
Cons: May have performance issues in terms of memory usage.
-
-
Alternative 2: Individual command knows how to undo/redo by itself.
-
Pros: Will use less memory (e.g. for
delete
, just save the member being deleted). -
Cons: We must ensure that the implementation of each individual command is correct.
-
Aspect: Type of commands that can be undone/redone
-
Alternative 1 (current choice): Only include commands that modify the club book (
add
,clear
,edit
)-
Pros: We only revert changes that are hard to change back (the view can easily be re-modified as no data is * lost).
-
Cons: User might think that undo also applies when the list is modified (undoing filtering for example), * only to realize that it does not do that, after executing
undo
.
-
-
Alternative 2: Include all commands
-
Pros: Might be more intuitive for the user.
-
Cons: Users have no way of skipping such commands if they just want to reset the state of the club * book and not the view. Additional Info: See our discussion here.
-
Aspect: Data structure to support the undo/redo commands
-
Alternative 1 (current choice): Use separate stack for undo and redo
-
Pros: Easy to understand for new Computer Science student undergraduates to understand, who are likely to be * the new incoming developers of our project.
-
Cons: Logic is duplicated twice. For example, when a new command is executed, we must remember to update * both
HistoryManager
andUndoRedoStack
.
-
-
Alternative 2: Use
HistoryManager
for undo/redo-
Pros: We do not need to maintain a separate stack, and can just reuse what is already in the codebase.
-
Cons: Requires dealing with commands that have already been undone: We must remember to skip these commands. Furthermore, the "Single Responsibility" and "Separation of Concerns" principles are violated as
HistoryManager
now needs to do two * different things.
-
4.2. Account Management
Account Management are authentication which members can store unique data into, logs into their own account to modify their account details and also start the Club Connect by Signing up the first user.
4.2.1. Current Implementation
Account Management mechanism is facilitated by several command classes in Club Connect.
Signing up
signup
mechanism is fascilitated by the SignUpCommand
class.
It allows the setting up of Club Connect.
SignUpCommand can only be executed once. Only can be executed again after clearing Club Connect. |
The SignUpCommand
extends for Command
. It is not an undoable command.
Figure 19 (shown below) depicts the UML representation of the SignUpCommand
.
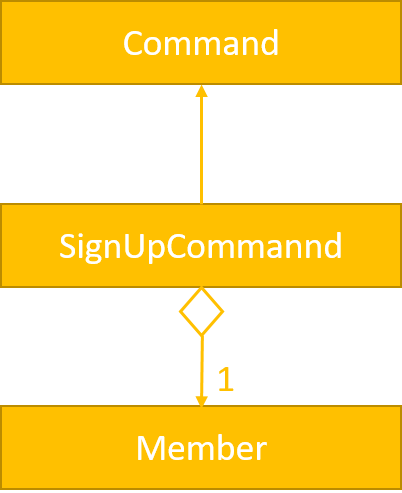
Figure 19. UML Diagram of SignUpCommand
.
Parsing of command is performed by SignUpCommandParser
, which returns a SignUpCommand
object after parsing Name, Phone, Email, Matric Number, [Tags].
Figure 20 below shows the sequence diagram of the SignUpCommandParser
.
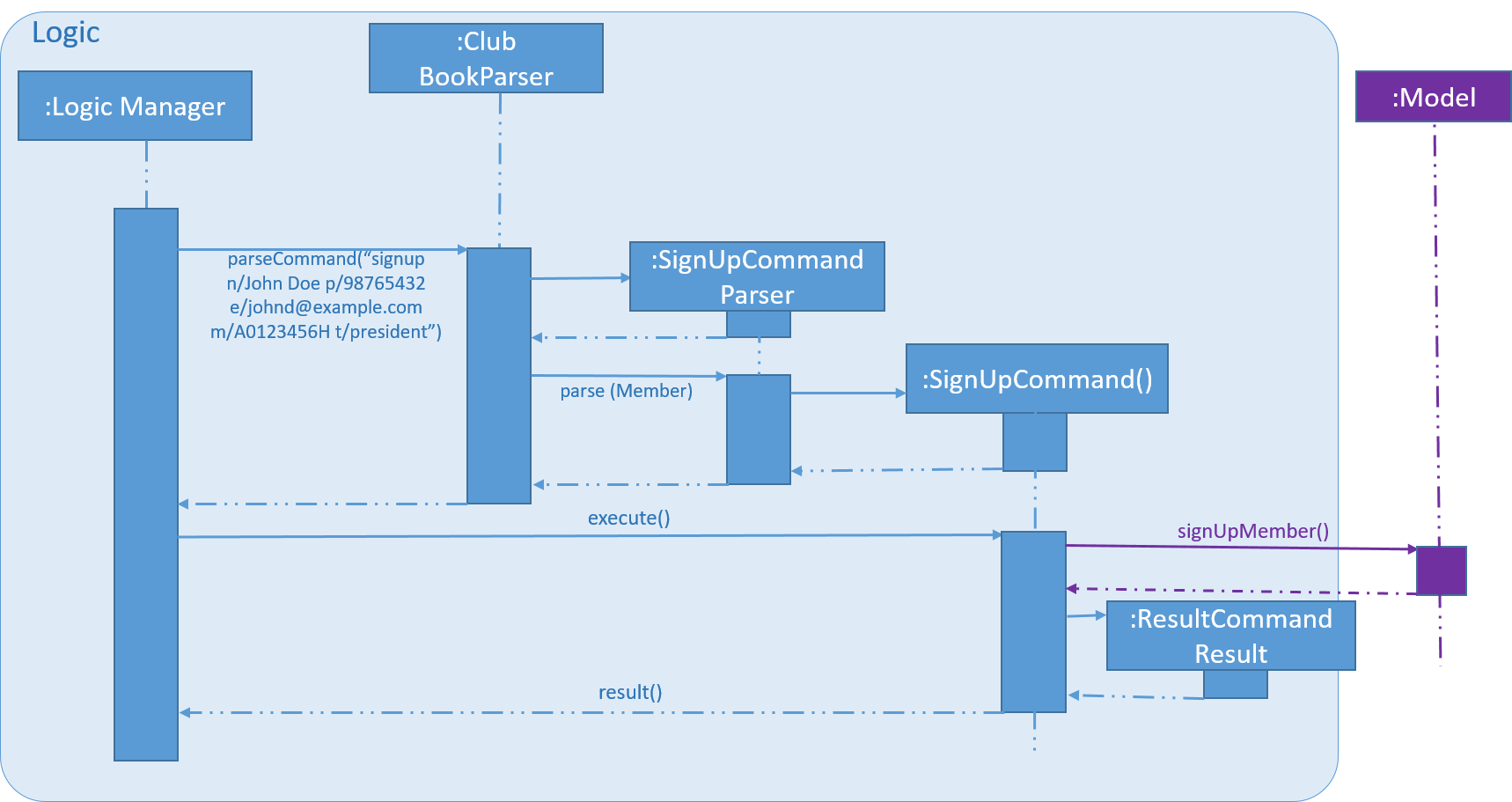
Figure 20. Sequence Diagram for the parsing of `SignUpCommandParser.`
Figure 21 below shows the high-level sequence diagram of the command execution.
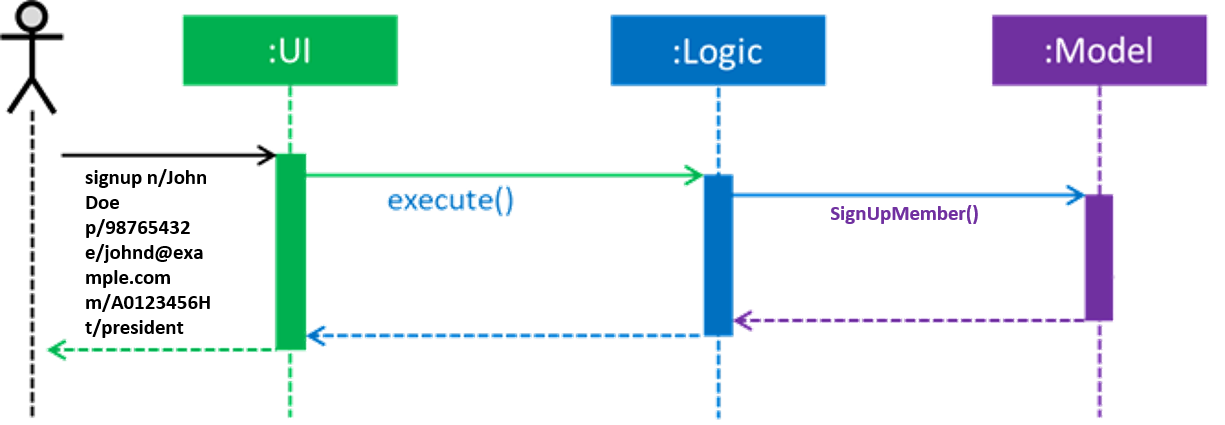
Figure 21. High-Level Sequence diagram of signing up a member.
Logging in
login
mechanism is facilitated by the LogInCommand
class.
It allows Exco
members to use Exco
privileges in the Club Book. It also allows both Members
and Exco
to have their own account.
The LogInCommand
consists of the following fields:
-
Username - Username of the member.
-
Password - Password of the member.
The LogInCommand
extends for Command
. It is not an undoable command.
Figure 22 (shown below) depicts the UML representation of the LogInCommand
.
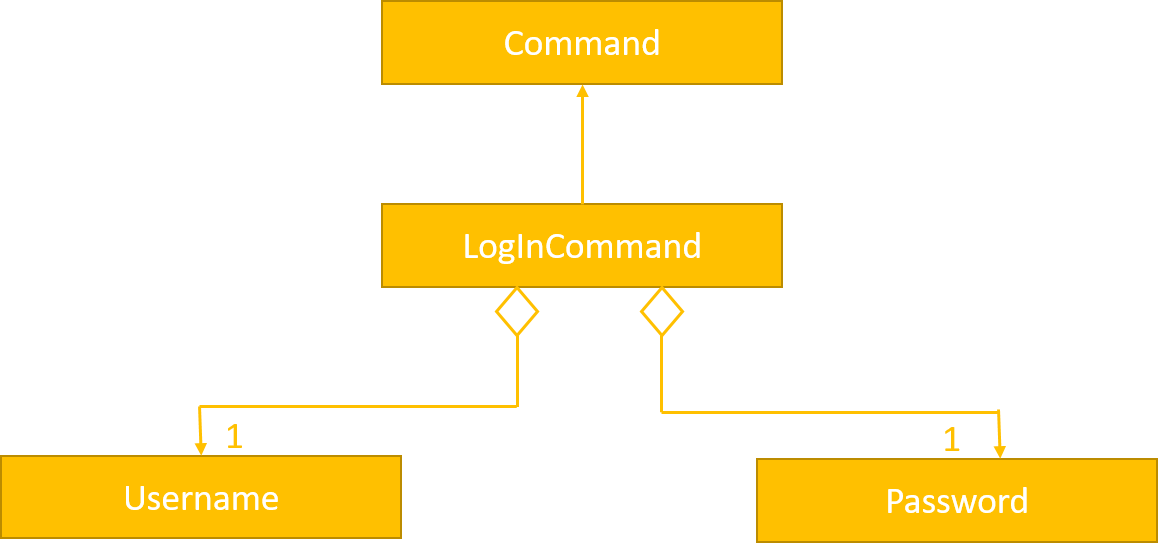
Figure 22. UML Diagram of LogInCommand
.
Parsing of command is performed by LogInCommandParser
, which returns a LogInCommand
object after parsing Username and Password object.
Figure 23 below shows the sequence diagram of the LogInCommandParser
.
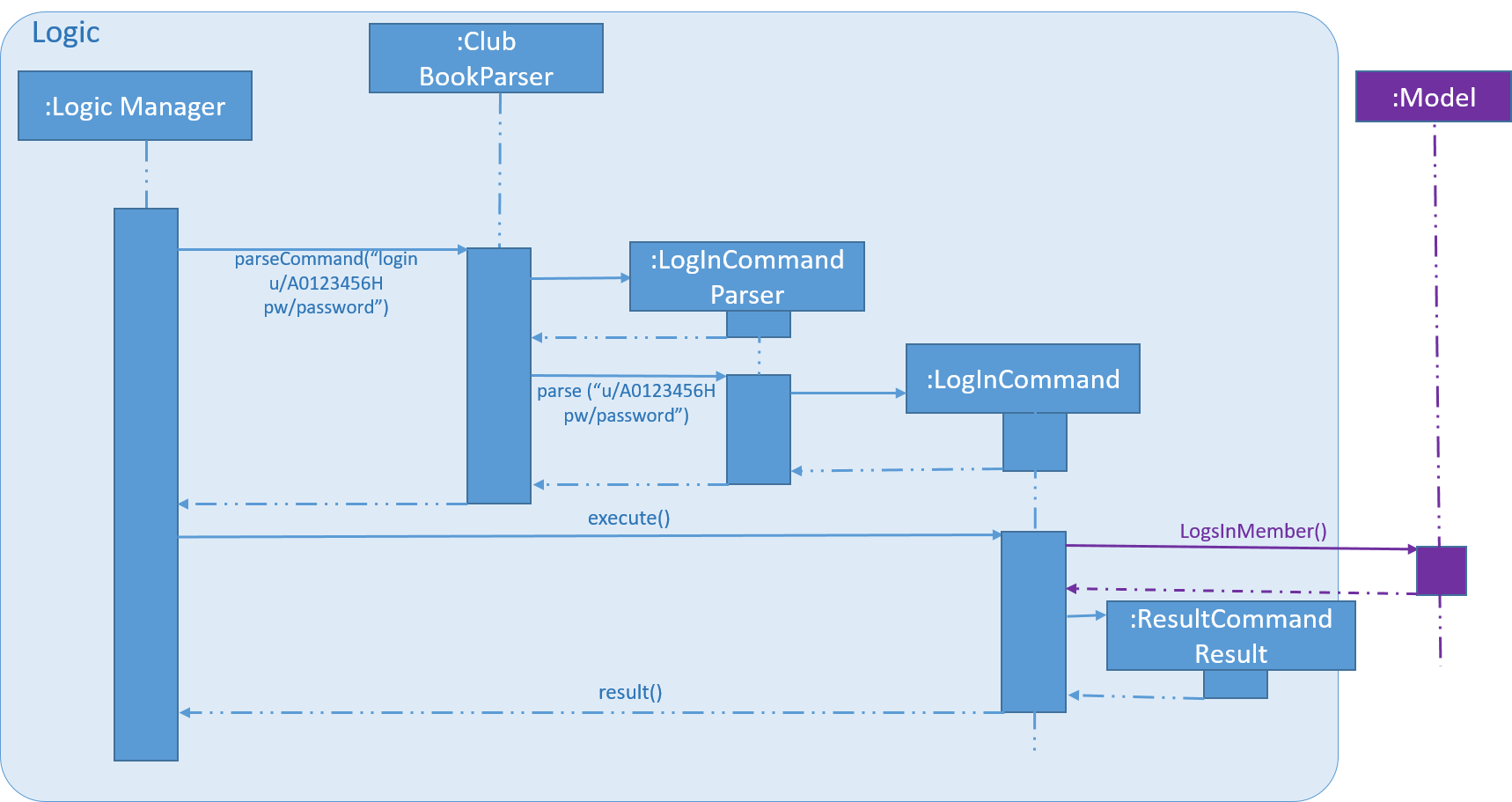
Figure 23. Sequence diagram of LogInCommandParser
.
Figure 24 below shows the high-level sequence diagram of the command execution.
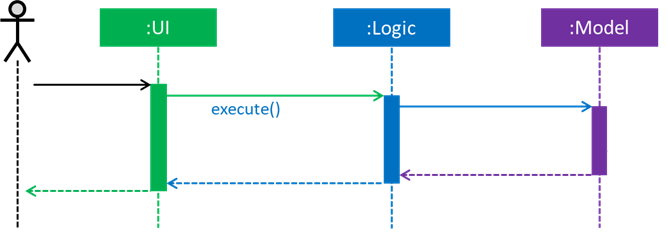
Figure 24. High-Level Sequence diagram of logging in a member.
4.2.2. Changing Password
changepass
mechanism is facilitated by the ChangePasswordCommand
class.
It allows Members
to change their current password to a new password.
The ChangePasswordCommand
consist fo the following fields:
-
Username - Username of the member.
-
Password - Current Password of the member.
-
NewPassword - New Password given by the member.
ChangePasswordCommand
extends from Command
and not from UndoableCommand
, as it is not an undoable command.
Figure 25 (shown below) depicts the UML representation of the ChangePasswordCommand
.
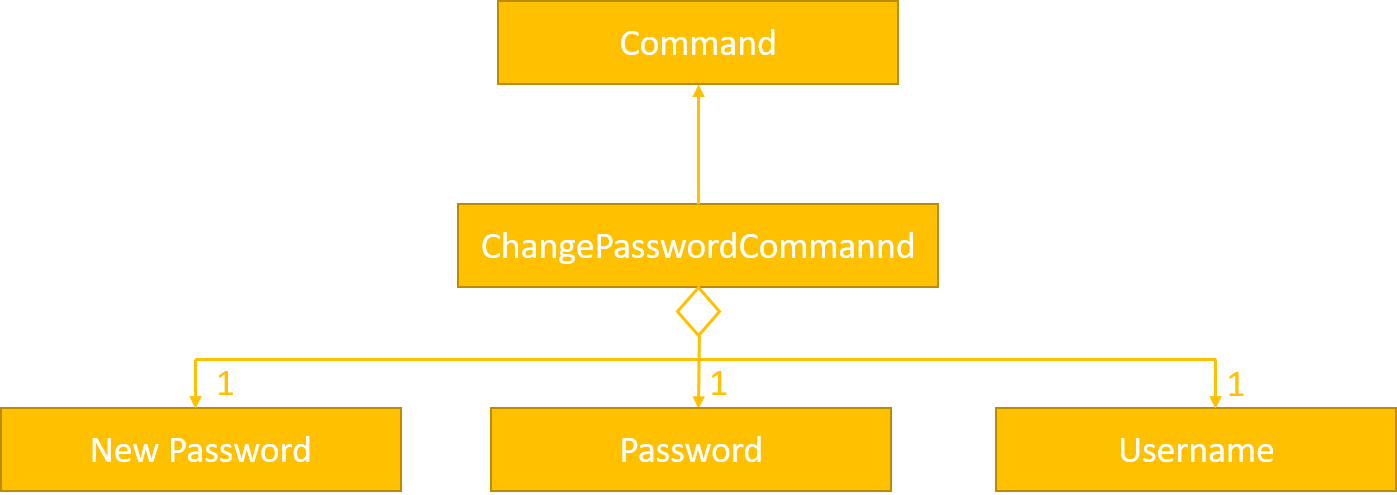
_Figure 25. UML Diagram of ChangePasswordCommand
.
Parsing of command is performed by ChangePasswordCommandParser
, which returns a ChangePassword
object after parsing Username, Password and Newpassword.
Figure 26 below shows the sequence diagram of the ChangePasswordCommandParser
.
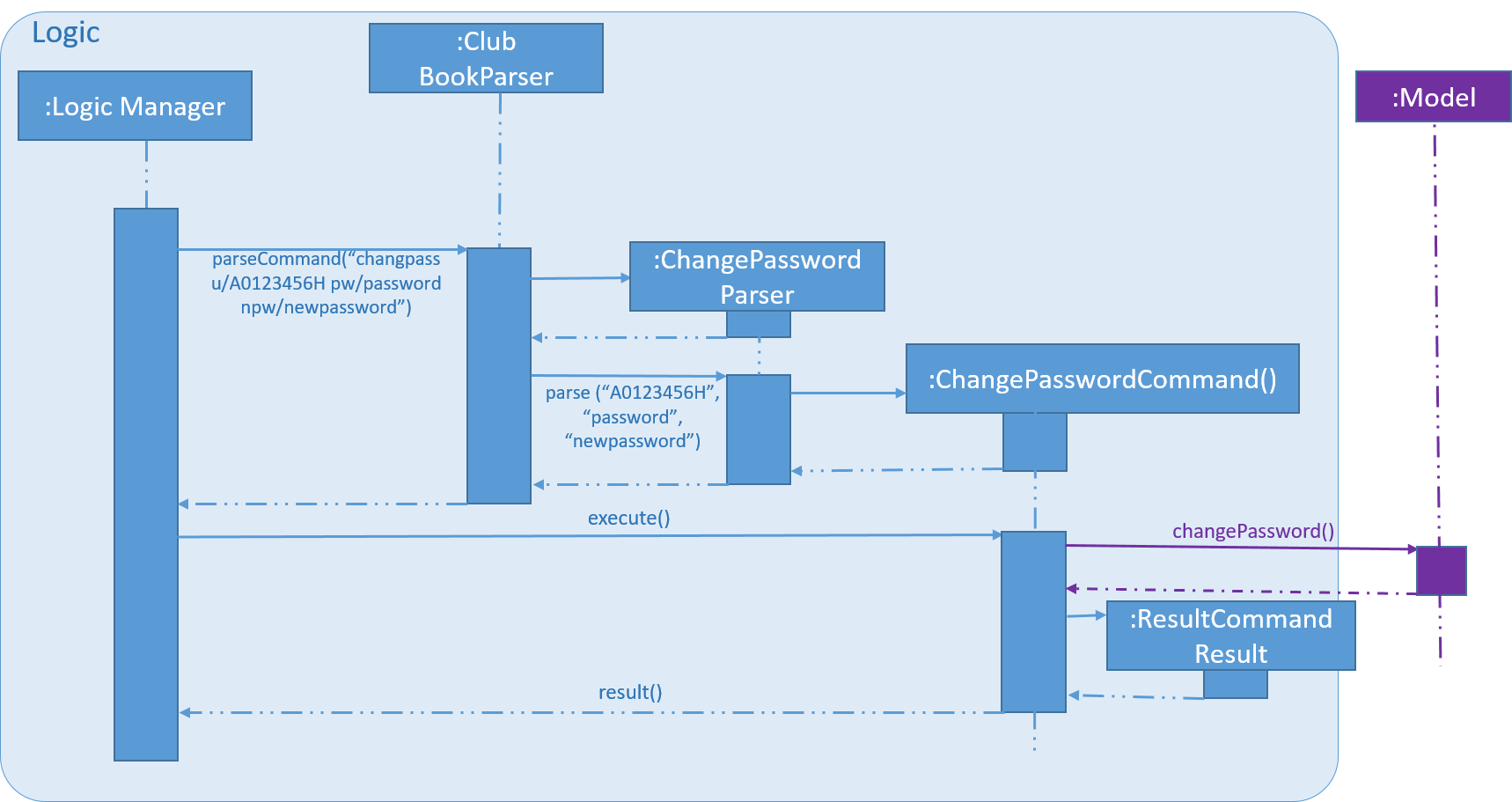
_Figure 26. Sequence diagram of ChangePasswordCommmandParser
.
Figure 27 below shows the high-level sequence diagram of the command execution.
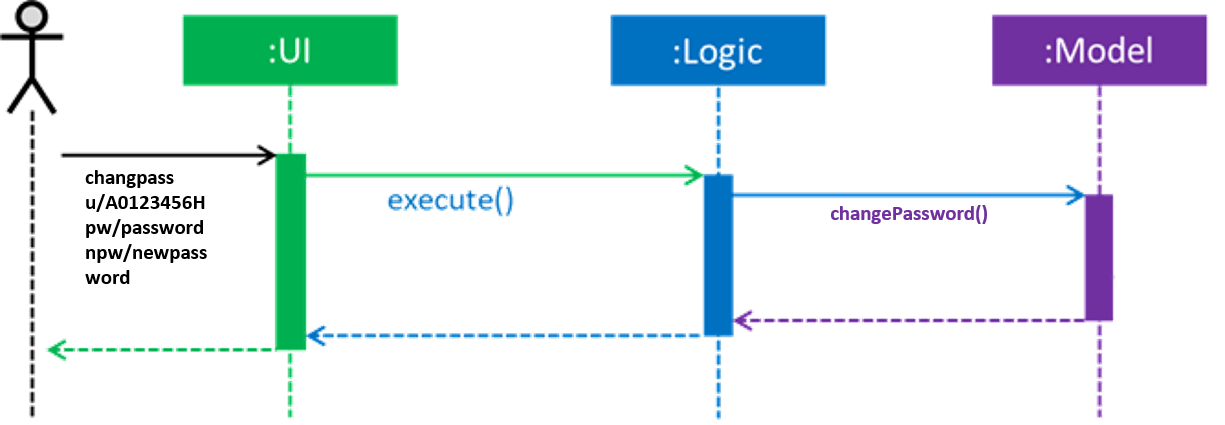
_Figure 27. High-Level Sequence diagam of changing the password of a member
4.3. Email Mechanism
4.3.1. Current Implementation
The email
mechanism of Club Connect is facilitated by the EmailCommand
class and is event-driven.
The EmailCommand
consists of the following fields:
-
Client - the mail client used to send the email (currently restricted to GMail and Outlook).
-
Subject - the subject of the email (optional field).
-
Body - the body of the email (optional field).
-
Group - the club group to whom the user wishes to send an email to.
-
Tag - the tag to which the user wishes to send an email to.
Emails can only be sent to members belonging to EITHER a Group OR a Tag. Club Connect currently doesn’t support sending emails to members belonging to BOTH a Group and a Tag. |
The EmailCommand
extends from Command
and not from UndoableCommand
, as it is not an undoable command.
Refer to Figure 28 for the UML diagram.
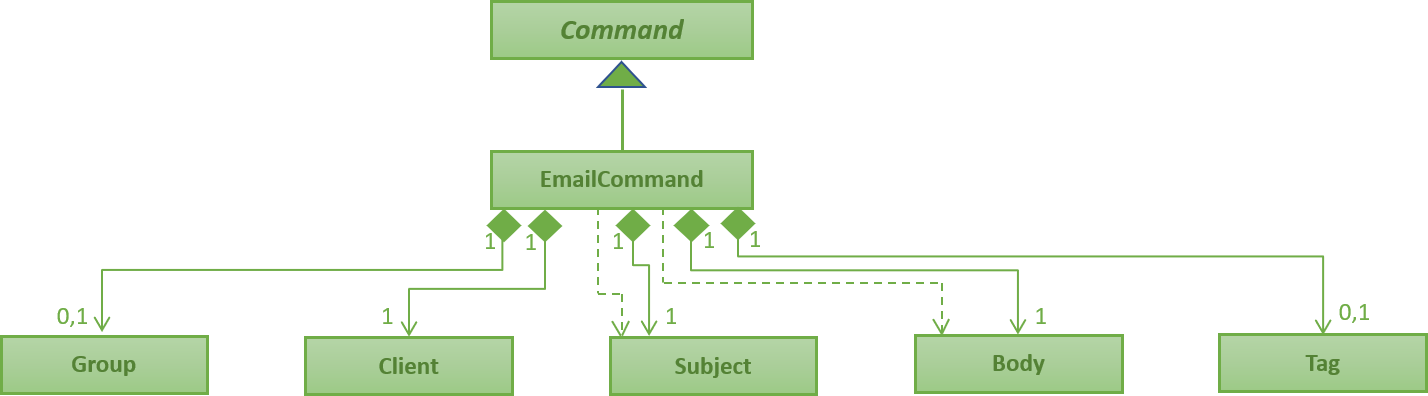
Figure 28. EmailCommand UML Diagram
EmailCommandParser
is responsible for parsing the email
command. It returns a EmailCommand
object after parsing Client
, Subject
, Body
, Group
, and Tag
.
Figure 29 depicts the sequence of parsing the command.
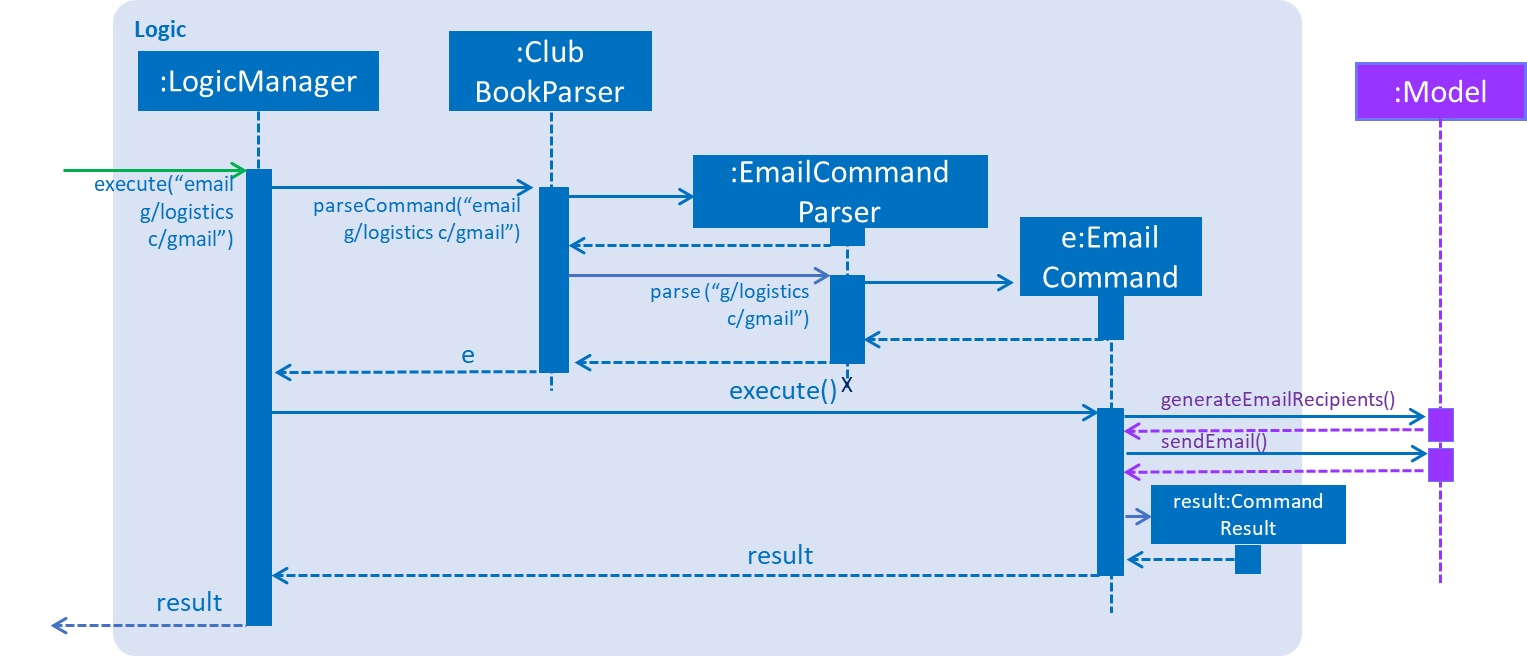
Figure 29. Sequence Diagram for Email Command parsing
Since Subject and Body are optional fields, their default values are EMPTY_SUBJECT_STRING and EMPTY_BODY_STRING which store blank Strings.
|
As Club Connect only allows emails to be sent to members of EITHER a Group OR a Tag , a valid command will result in either one of them being assigned to null .
|
The EmailCommand#execute()
calls the following methods of model
:
-
- takes in agenerateEmailRecipients()
Group
andTag
object. Depending on the object that is notnull
, it returns a string of recipients. -
- takes in the recipient string, and an object each ofsendEmail()
Client
,Subject
, andBody
.
EmailCommand#sendEmail()
raises an event SendEmailRequestEvent
, which triggers the system’s default browser to open the chosen mail client’s 'Compose Message' page with all the relevant fields filled-in.
Figure 30 depicts the high-level sequence of events that take place.
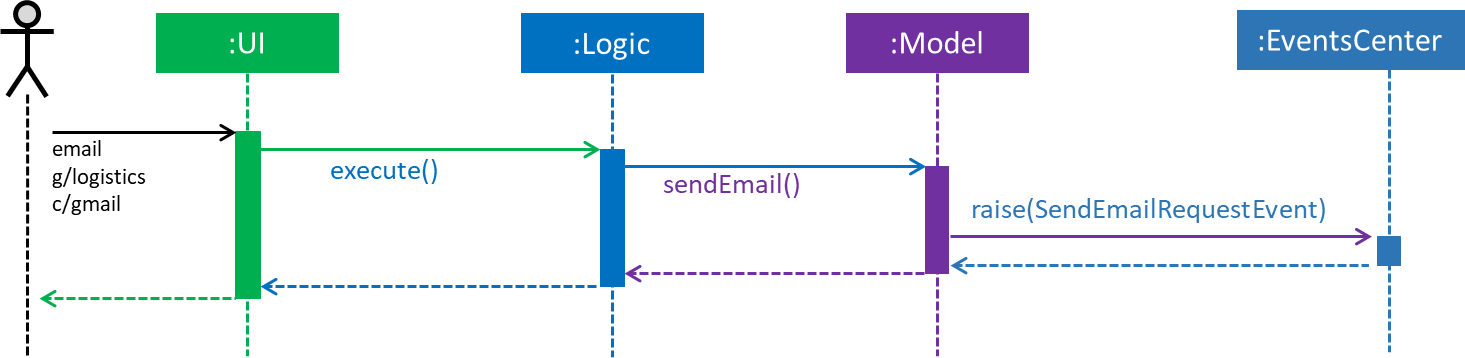
Figure 30. Sequence Diagram for Email Command
The browser URL for composing the email is generated as follows:
public static final String GMAIL_EMAIL_URL =
"https://mail.google.com/mail/?view=cm&fs=1&tf=1&source=mailto&to=%1$s&su=%2$s&body=%3$s";
public static final String OUTLOOK_EMAIL_URL =
"https://outlook.office.com/?path=/mail/action/compose&to=%1$s&subject=%2$s&body=%3$s";
Desktop.getDesktop().browse(new URI(String.format(GMAIL_EMAIL_URL, recipients, subject, body)));
4.3.2. Design Considerations
Aspect: Location of opening the mail client
-
Alternative 1 (current choice): Open up the mail client in system’s default web browser.
-
Pros: Easy to implement
-
Cons: Since Club Connect can be used by multiple people via user authentication, the default browser will have the owner of the system logged into his/her mail account.
-
-
Alternative 2: Open up the mail client in
BrowserPanel
.-
Pros: No dependency on third party apps.
-
Cons: Older version browser will disallow auto-filling of email fields.
-
4.4. Task-Management
Tasks are pieces of work to be done or undertaken. Members can create tasks for themselves, and can also be assigned to a task by Exco
members.
4.4.1. Current Implementation
The task-management mechanism is facilitated by several command classes in Club Connect, which will be covered in subsequent sub-sections.
Task-management commands require a member to be logged in (and thus by extension, an initial sign-up).
Figure 31 below shows the UML diagram of the Task
class.
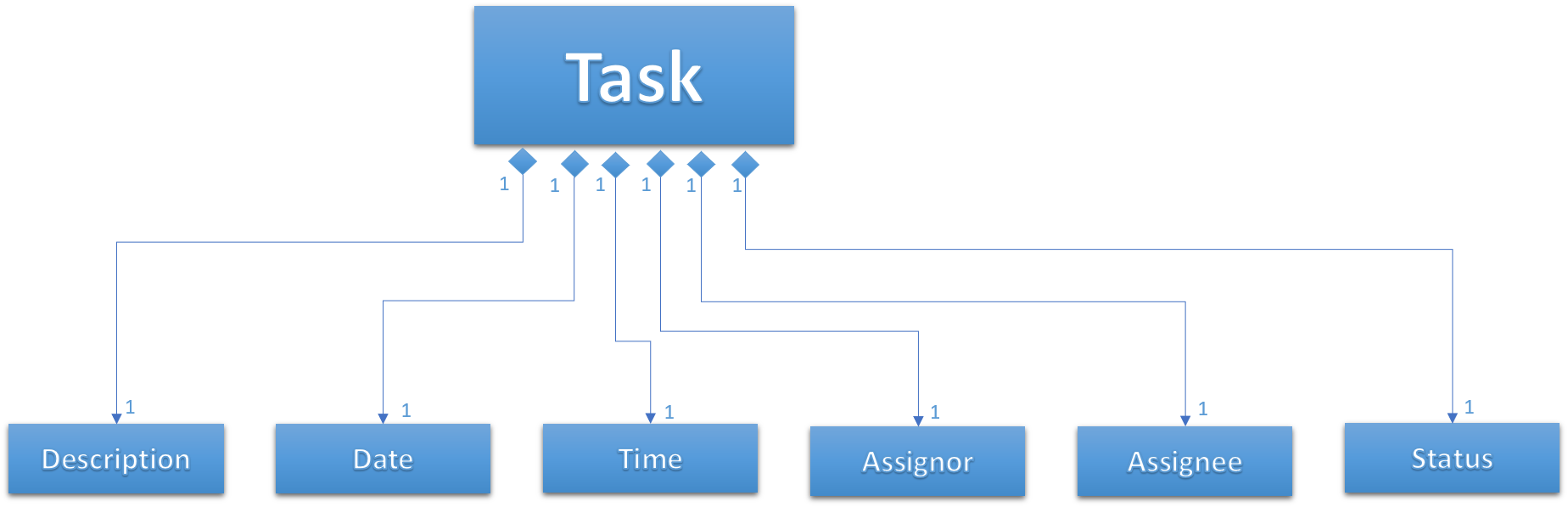
Figure 31. Task UML Diagram
Adding Tasks
Adding Tasks is facilitated by the AddTaskCommand
. When this command is invoked, it adds a task with the input Description
, Date
, and Time
. The status of every newly created task is by default set to Yet To Begin
. The Assignor
and Assignee
of the task is set to the Matric Number
of the member who is currently logged into Club Connect
.
Figure 32 below shows the UML representation of AddTaskCommand
.
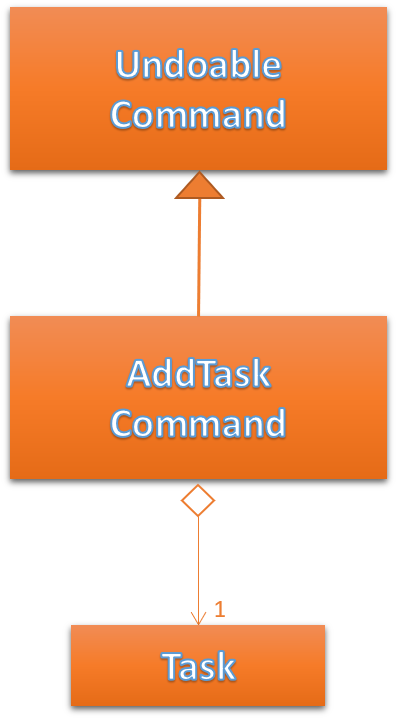
Figure 32. UML diagram of AddTaskCommand
.
Figure 33 shows the flow of parsing an AddTaskCommand
object:
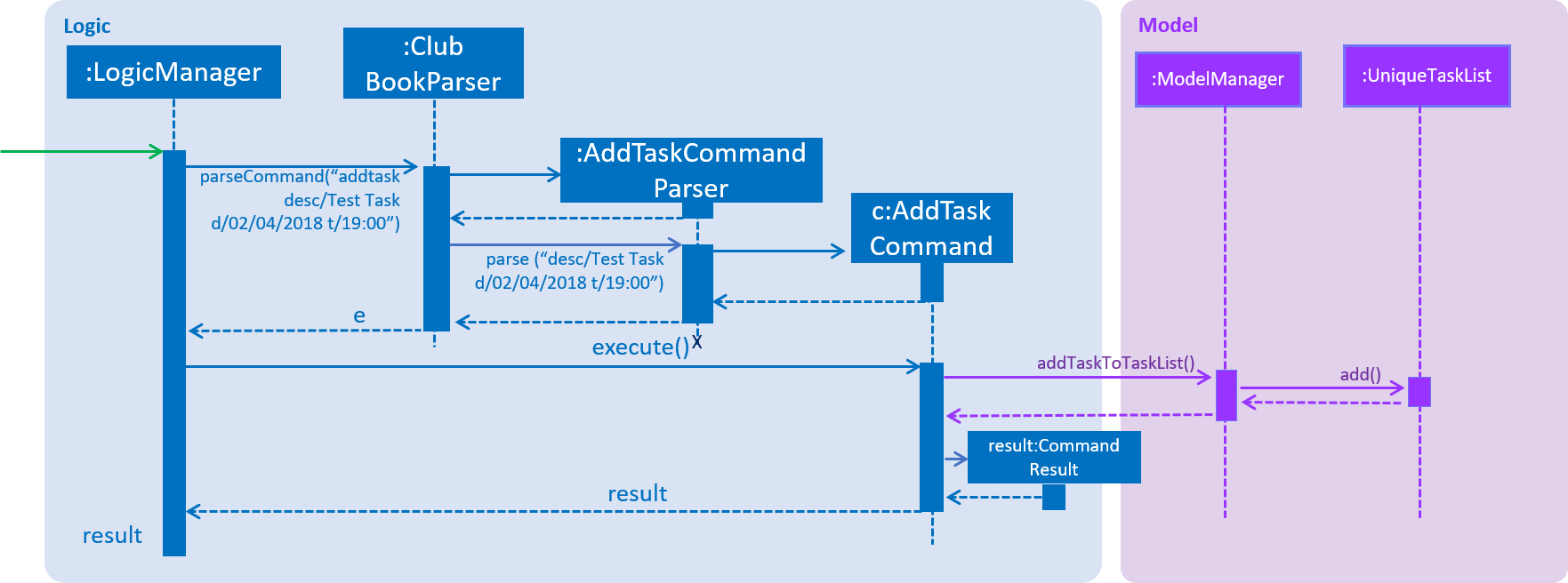
Figure 33. Sequence Diagram for AddTaskCommand
parsing.
Figure 34 depicts the high-level sequence of events that take place.

Figure 34. Sequence Diagram for adding a task.
4.4.2. Design Considerations
Aspect: Displaying Assignor and Assignee
-
Alternative 1 (current choice): Displays currently logged-in member as Assignor and Assignee.
-
Pros: It is required for
assigntask
. -
Cons: Displaying the same matriculation number twice is redundant.
-
-
Alternative 2: Don’t display Assignor and Assignee as it is a personal to-do list.
-
Pros: Takes care of redundancies in Alternative 1 above.
-
Cons: The implementation of
assigntask
becomes more complex.
-
Assigning Tasks
Assigning tasks can be accomplished with the AssignTaskCommand
. Tasks are assigned to members through the Matric Number
attribute.
Figure 35 below shows the UML representation of AssignTaskCommand
.
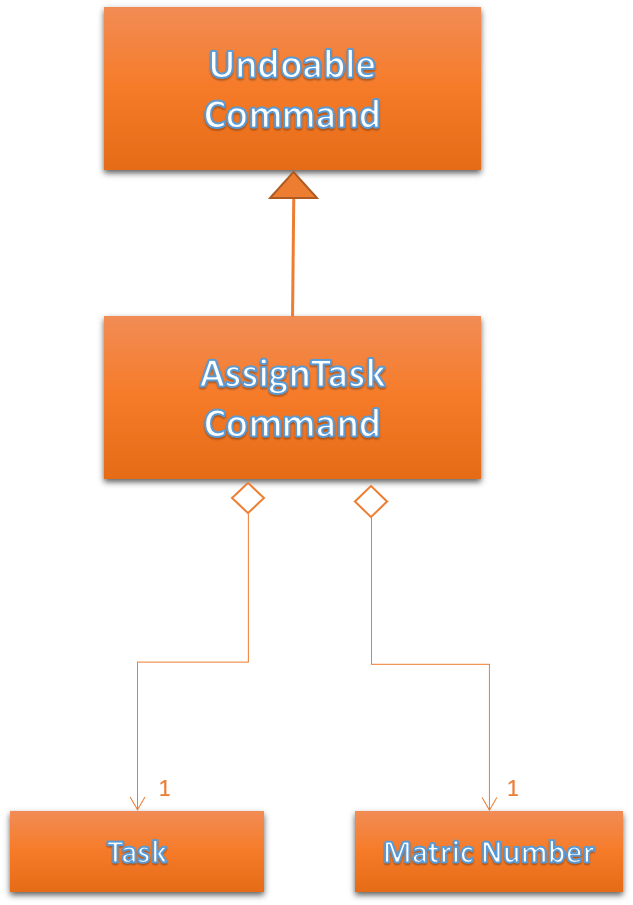
Figure 35. UML diagram of AssignTaskCommand
.
The implementation of assigning tasks is similar to that of adding tasks. The only difference is that the Assignor is set to the Matric Number
of the member who is currently logged in.
The parsing of an AssignTaskCommand
is performed by the AssignTaskCommandParser
.
Figure 36 shows the flow of parsing an AssignTaskCommand
object:
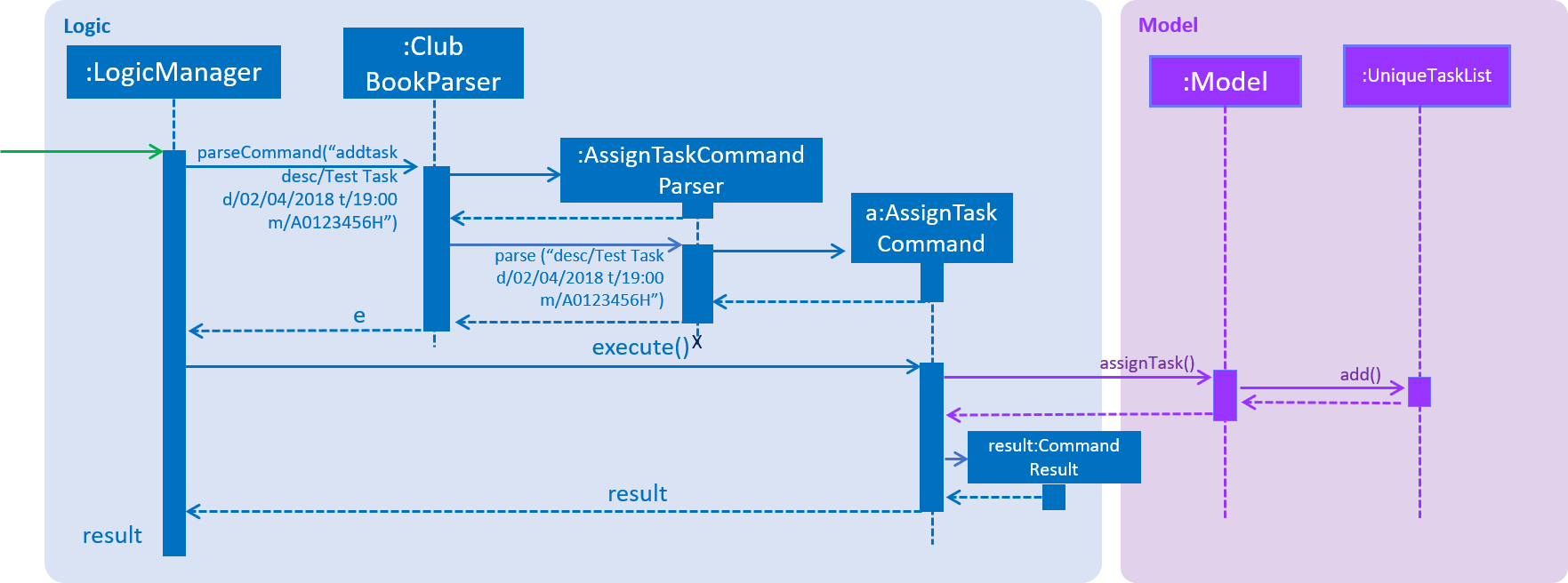
Figure 36. Sequence Diagram for AssignTaskCommand
parsing.
Figure 37 depicts the high-level sequence of events that take place.

Figure 37. Sequence Diagram for assigning a task.
4.4.3. Design Considerations
Aspect: Displaying similar tasks for different Assignees
-
Alternative 1 (current choice): Make a copy of the task for every new
Assignee
if all other parameters are same.-
Pros: Easy to implement
-
Cons: Clutters the
TaskListPanel
because of redundancies.
-
-
Alternative 2: Maintain a list of
Assignee
s in a task .-
Pros: Reduces clutter in the
TaskListPanel
and is easy on the eye. -
Cons: Relatively difficult to implement.
-
Deleting Tasks
The 'DeleteTaskCommand` is used to delete a task. A task can only be deleted if the member who is currently logged in is either the Assignor
or the Assignee
.
The command object takes in the INDEX
of the task to be deleted.
Figure 38 below shows the UML representation of DeleteTaskCommand
.
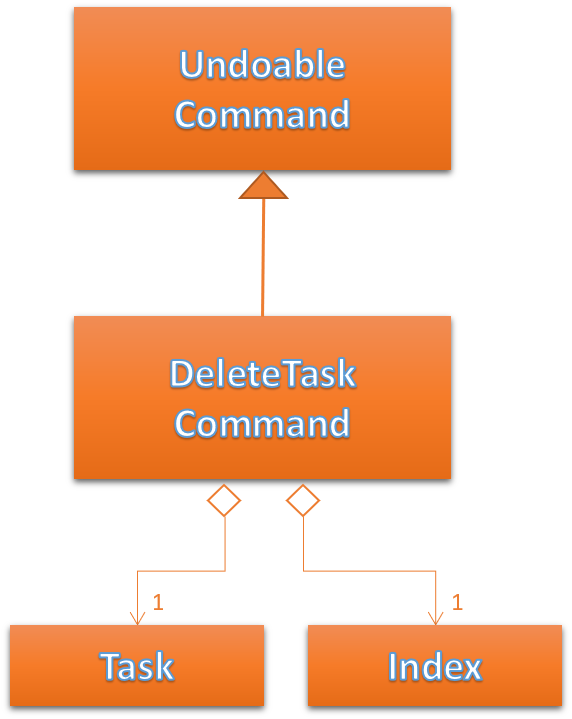
Figure 38. UML diagram of DeleteTaskCommand
.
DeleteTaskCommandParser
is responsible for parsing the user input into a DeleteTaskCommand
object.
Figure 39 illustrates the sequence in which the parsing is done.
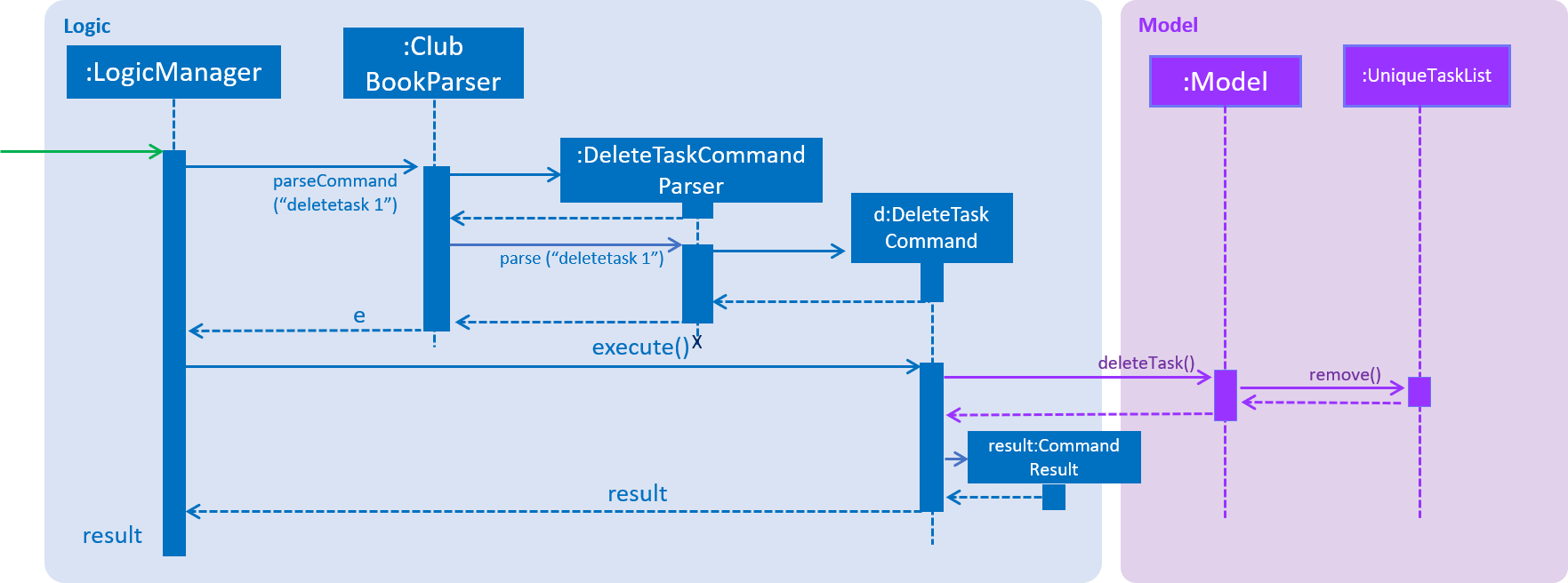
Figure 39. Sequence Diagram for DeleteTaskCommand
parsing.
The high-level sequence of events is shown below in Figure 40.

Figure 40. Sequence Diagram for deleting a task.
4.4.4. Design Considerations
Aspect: Who can delete tasks?
-
Alternative 1 (current choice): Only Assignors can delete tasks.
-
Pros: It improves accountability of the club.
-
Cons: All the workload falls on the Assignor.
-
-
Alternative 2: Assignor or Assignee can delete task.
-
Pros: The workload is evenly distributed.
-
Cons: Reduces transparency of club and accountability of club members.
-
Changing Task Assignee
The ChangeAssigneeCommand
is used to change the Assignee
of a task.
Figure 41 below shows the UML representation of ChangeAssigneeCommand
.
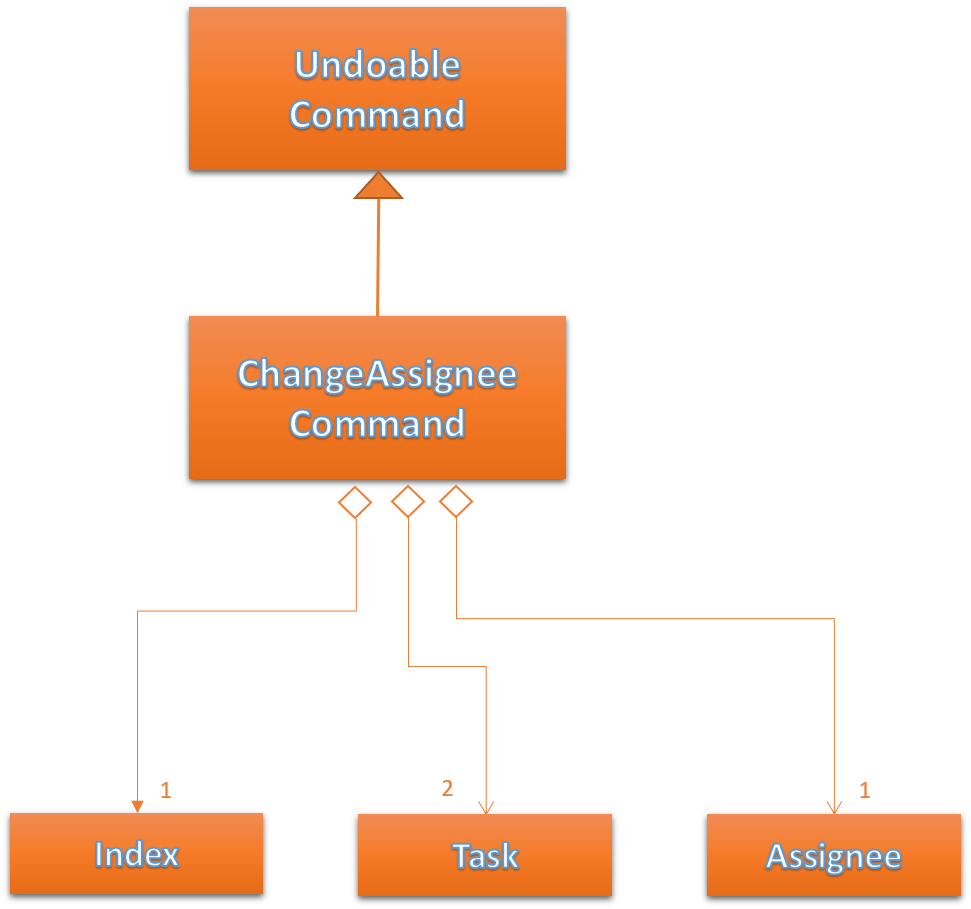
Figure 41. UML Diagram of ChangeAssigneeCommand
.
ChangeAssigneeCommandParser
is responsible for parsing the changeassignee
command. It returns a ChangeAssigneeCommand
object after parsing the INDEX
and the Assignee
.
Figure 42 shows the sequence diagram of the ChangeAssigneeCommandParser
.
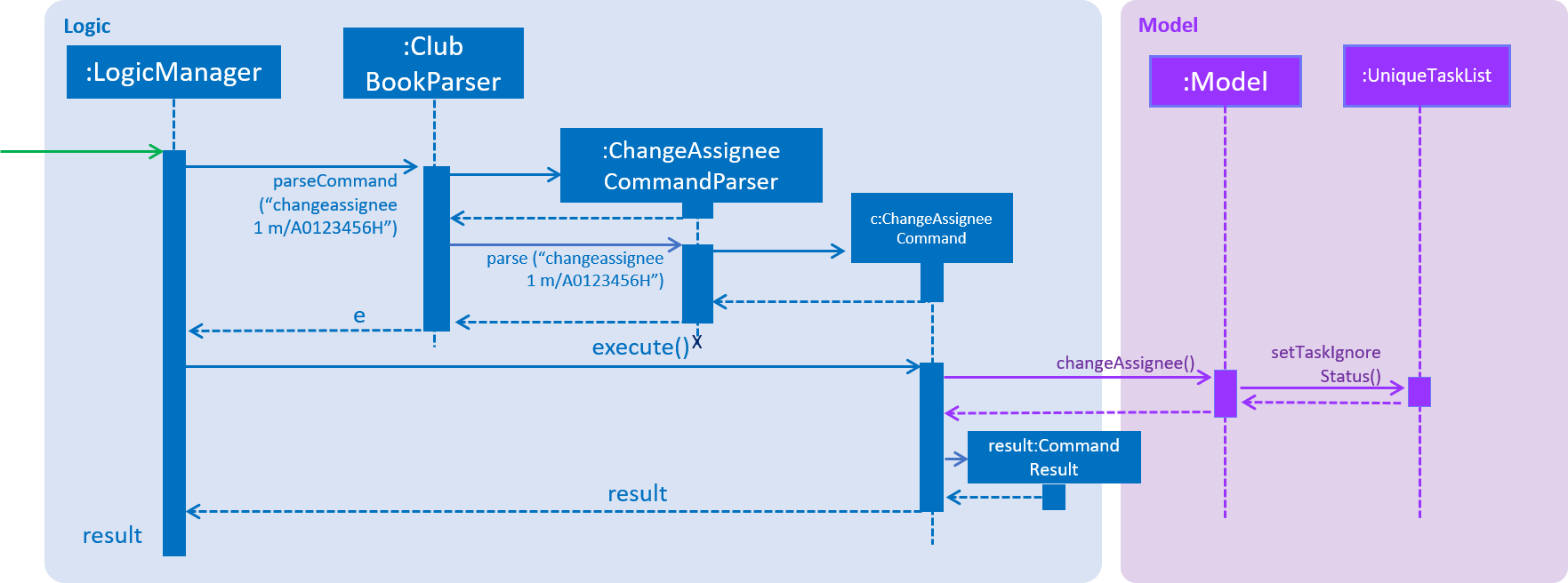
Figure 42. Sequence Diagram of ChangeAssigneeCommandParser
.
Figure 43 below shows the high-level sequence diagram of the command execution.

Figure 43. Sequence Diagram of changing the Assignee
of a task.
4.4.5. Design Considerations
Aspect: Who can change assignees?
-
Alternative 1 (current choice): Only Exco members can change assignees of tasks.
-
Pros: It improves accountability of the club.
-
Cons: All the workload falls on the Exco members.
-
-
Alternative 2: Assignor or Assignee can change the assignee task.
-
Pros: People who are directly related to the task take up resonsibility. This boosts accountability of members.
-
Cons: Similar to deleting a task, it reduces transparency of club and accountability of club members.
-
Changing Task Status
Changing a task’s status is made possible by the ChangeTaskStatusCommand
.
Figure 44 below shows the UML representation of ChangeTaskStatusCommand
.
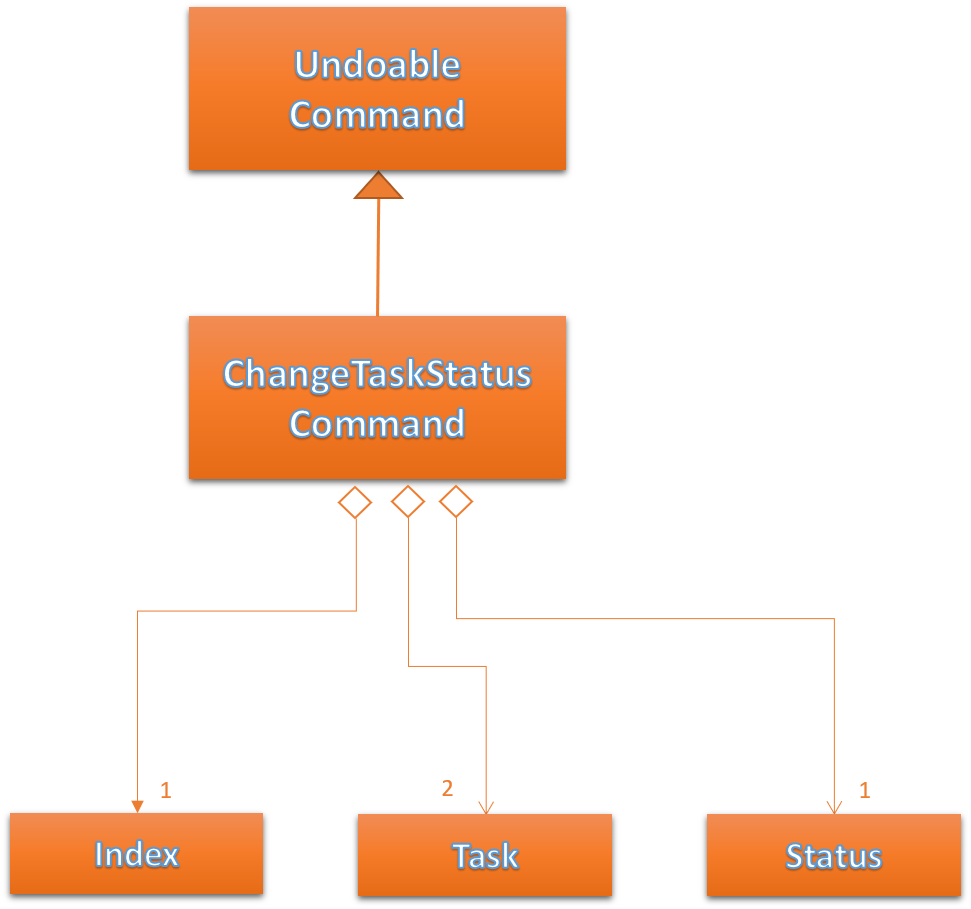
Figure 44. UML Diagram of ChangeTaskStatusCommand
.
Parsing of the command is performed by ChangeTaskStatusCommandParser
, which returns a ChangeTaskStatusCommand
object.
Figure 45 below depicts the parsing of the ChangeTaskStatusObject
.
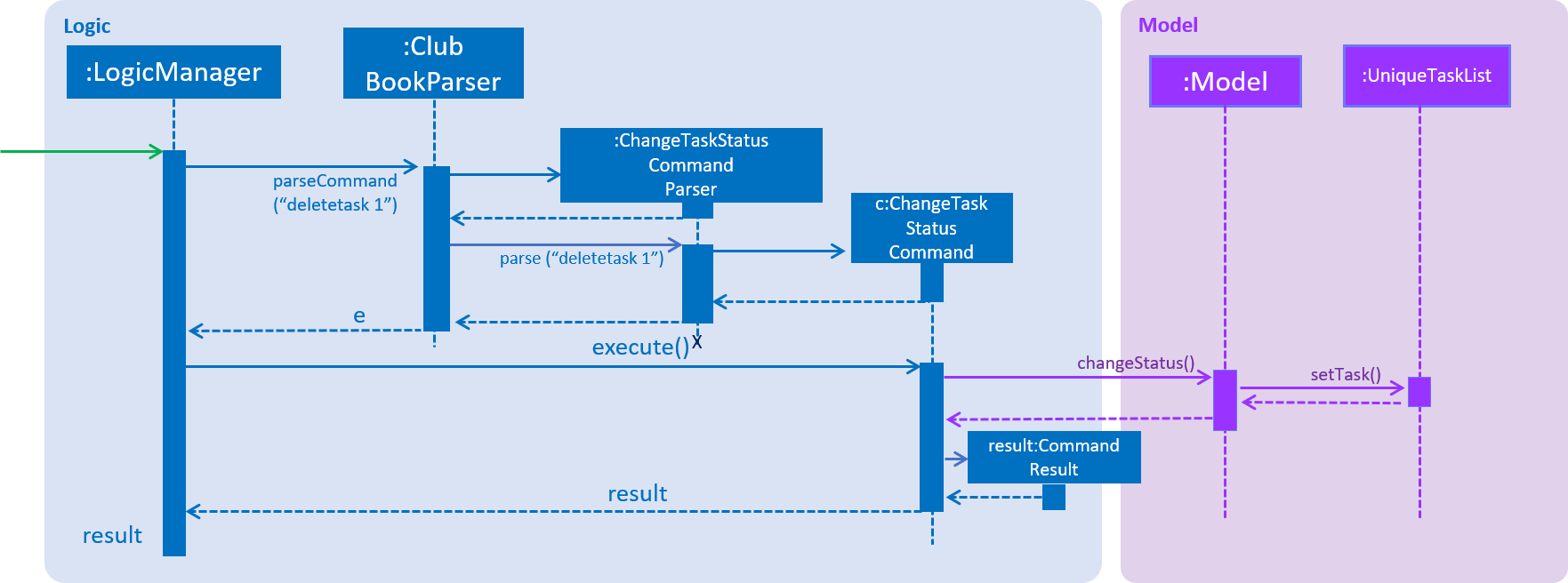
Figure 45. Sequence Diagram for ChangeTaskStatusCommand
parsing.
Figure 46 below describes the high-level sequence of events that take place.

Figure 46. Sequence Diagram for changing the status of a task.
4.4.6. Design Considerations
Aspect: Who can change task status?
-
Alternative 1 (current choice): Assignor or Assignee can change the task status.
-
Pros: They are directly associated with the task.
-
Cons: Exco should also have a say.
-
-
Alternative 2: Exco, Assignee or Assignor can change status.
-
Pros: Exco’s powers kept in check.
-
Cons: Exco may misinterpret status of the task.
-
Viewing All Tasks
Exco
members can view all the tasks in Club Connect
using the ViewAllTasksCommand
.
The high-level sequence of events on executing the ViewAllTasksCommand
is described below in Figure 47.
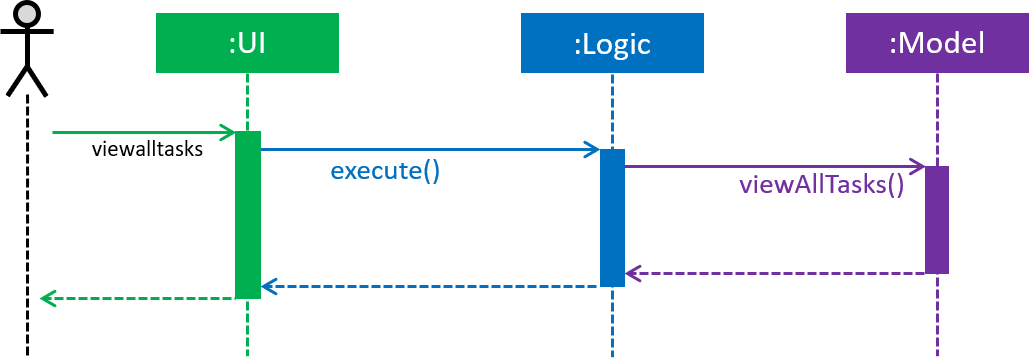
Figure 47. Sequence Diagram for viewing all tasks.
Viewing My Tasks
Exco
members need a way to toggle between all tasks in Club Connect
and the tasks that are related to them (i.e. tasks that they have assigned or been assigned to). This is achieved by the ViewMyTasksCommand
.
The Predicate
used is TaskIsRelatedToMemberPredicate
. Here is the overridden TaskIsRelatedToMemberPredicate#test
method.
@Override
public boolean test(Task task) {
return member.getMatricNumber().toString().equalsIgnoreCase(task.getAssignor().getValue())
|| member.getMatricNumber().toString().equalsIgnoreCase(task.getAssignee().getValue());
}
The sequence diagram of the viewmytasks
command is shown below in Figure 48.
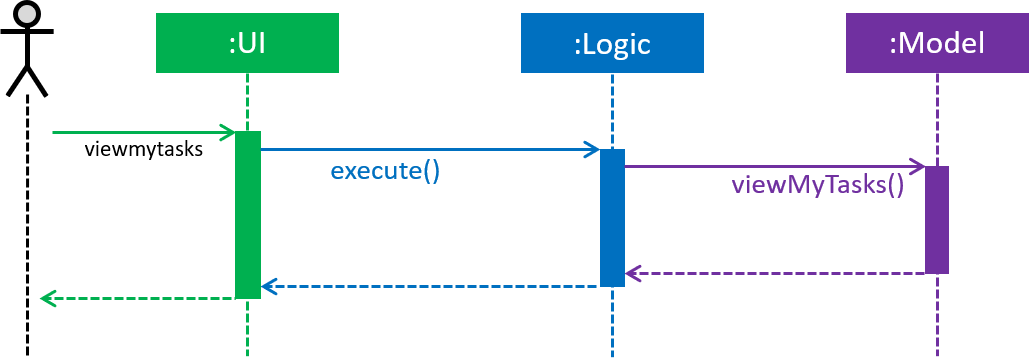
Figure 48. Sequence Diagram for viewing tasks of the currently logged-in member.
4.5. Polls-feature
A poll contains a question and any number of answers. Any member can vote in a poll but only once. Only 'Exco' members
are allowed to add/delete polls and view/hide poll results. Polls are displayed in the poll ListView
GUI of Club Connect.
4.5.1. Current Implementation
The poll feature is facilitated by the command classes below:
-
AddPollCommand
-
DeletePollCommand
-
VoteCommand
-
ViewResultsCommand
-
HideResultsCommand
Since all the commands above require the user be logged in, the statements below are present in their execute
methods.
requireToSignUp();
requireToLogIn();
To restrict the commands that only Exco
members can execute, the AddPollCommand
, DeletePollCommand
,
ViewResultsCommand
and HideResultsCommand
will have the statement below in their execute
methods.
requireExcoLogIn();
Figure 49 below shows the UML diagram of the Poll
class.
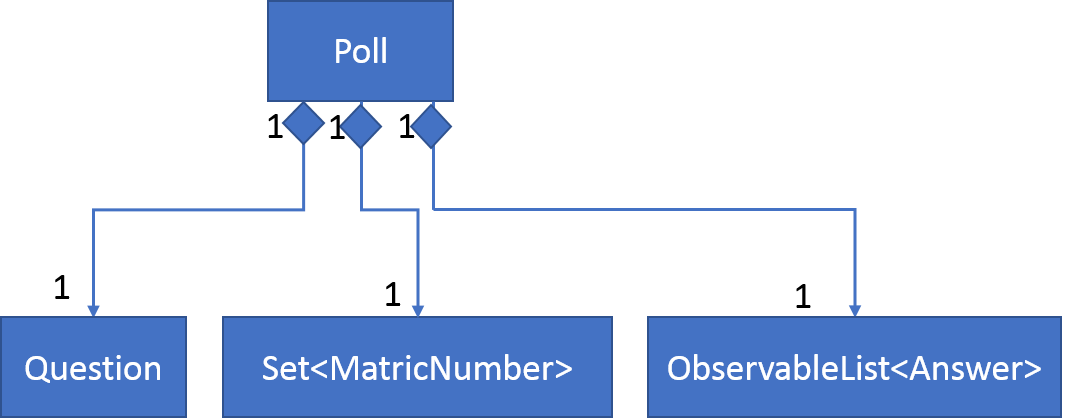
Figure 49. Poll UML Diagram
A Poll
consists of the following:
-
Question: Represents the question of the poll.
-
ObservableList<Answer>: Represents the answers of the poll.
-
Set<MatricNumber>: Represents all members that have voted in the poll.
Figure 50 below shows the UML diagram of the Answer
class.
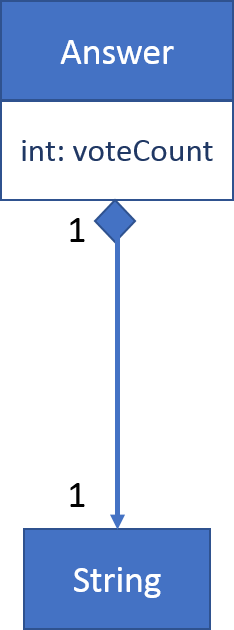
Figure 50. Poll UML Diagram
An Answer
consists of the following:
-
String: Represents the sequence of characters making up the answer
-
int: Represents the number of members who voted for this answer
Adding Polls
Adding Polls is facilitated by the AddPollCommand
which inherits from UndoableCommand
to make adding polls undoable.
When a poll is created by the AddPollCommand
, it’s Set<MatricNumber>
is initialized as empty because no member has voted in the poll.
Figure 51 shows the flow of adding a poll:
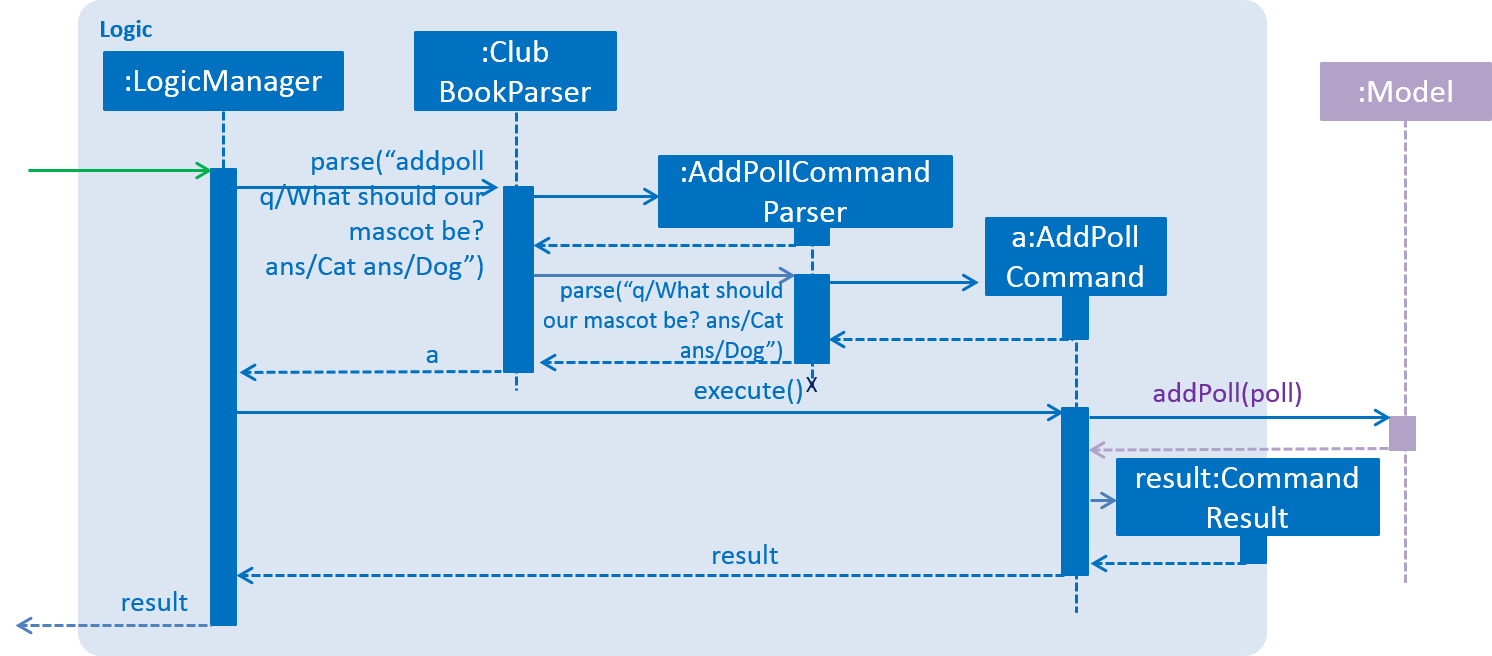
Figure 51. Sequence Diagram for adding a poll
The execution of the command invokes Model#addPoll(poll)
.
The following code snippet shows the implementation:
@Override
public synchronized void addPoll(Poll poll) throws DuplicatePollException {
requireNonNull(poll);
clubBook.addPoll(poll);
updateFilteredPollList(new PollIsRelevantToMemberPredicate(getLoggedInMember()));
indicateClubBookChanged();
}
After successful execution, the poll will be added to the Club Book and the poll ListView
GUI.
Deleting Polls
Deleting Polls is facilitated by the DeletePollCommand
which inherits from UndoableCommand
to make deleting polls undoable.
Figure 52 shows the flow of deleting a poll:
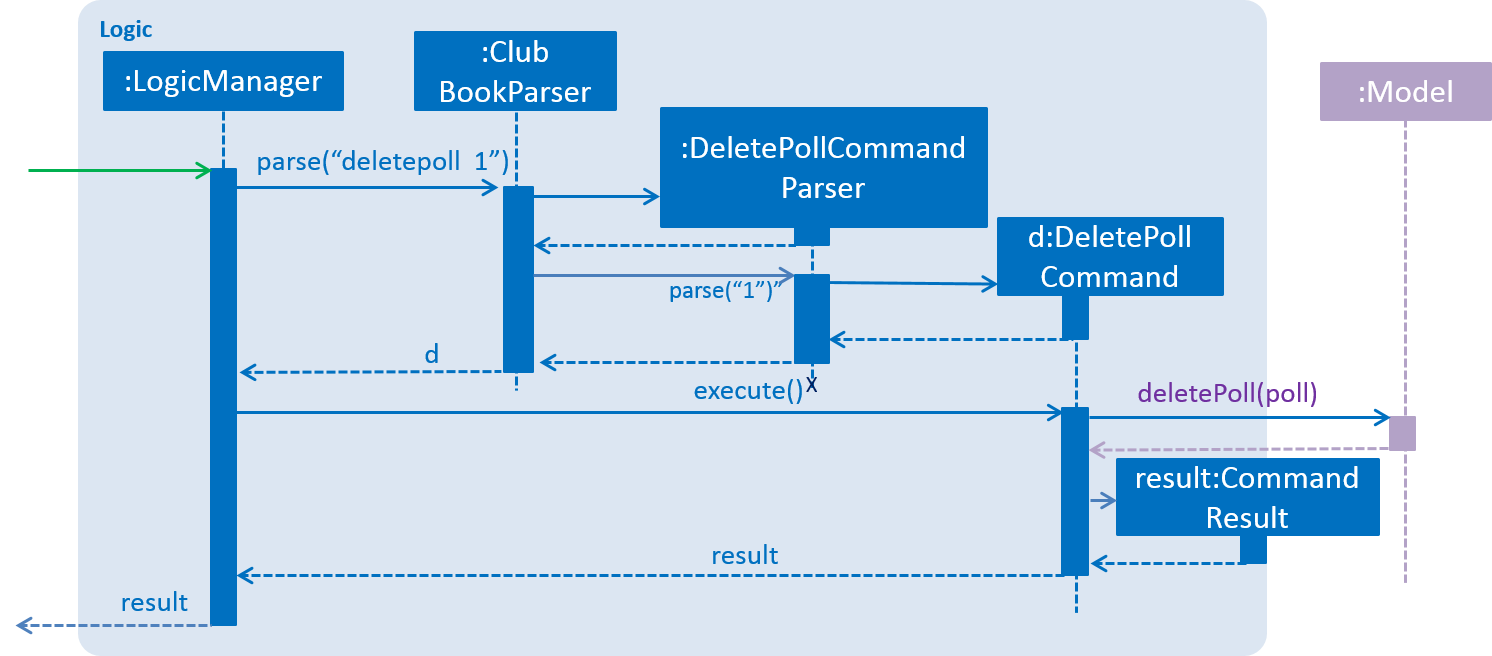
Figure 52. Sequence Diagram for deleting a poll
The execution of the command invokes Model#deletePoll()
.
The following code snippet shows the implementation:
@Override
public synchronized void deletePoll(Poll target) throws PollNotFoundException {
requireNonNull(target);
clubBook.removePoll(target);
indicateClubBookChanged();
}
After successful execution, the poll will be deleted from the Club Book and the poll ListView
GUI.
Voting in Polls
Voting in polls is facilitated by the VoteCommand
which inherits from UndoableCommand
to make voting undoable.
The input of the command is the POLL_INDEX
of a poll and ANSWER_INDEX
of the answer of the poll
Figure 53 shows the flow of voting in a poll:
![width]"550"](images/SDforVote.png)
Figure 53. Sequence Diagram for voting in a poll
The execution of the command invokes Model#voteInPoll()
.
The following code snippet shows the implementation:
@Override
public String voteInPoll(Poll poll, Index answerIndex)
throws PollNotFoundException, AnswerNotFoundException, UserAlreadyVotedException {
requireAllNonNull(poll, answerIndex);
String voteDetails = clubBook.voteInPoll(poll, answerIndex, getLoggedInMember().getMatricNumber());
indicateClubBookChanged();
return voteDetails;
}
After successful execution, the voteCount of the answer in the poll will increment by 1. If the user is not an Exco
member
the poll will be removed from the poll ListView
GUI, otherwise it will remain in the GUI for Exco
members to monitor the results.
Viewing/Hiding All Poll Results
Viewing and Hiding Poll Results is facilitated by the ViewResultsCommand
and HideResultsCommand
.
By default poll results are not shown(Figure 54), but when ViewResultsCommand
is executed, results consisting of
how many people voted in a poll and how many voted for each answer will be shown(Figure 55).
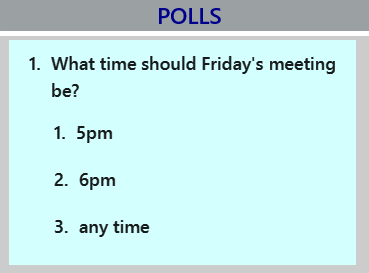
Figure 54. Poll Without Results
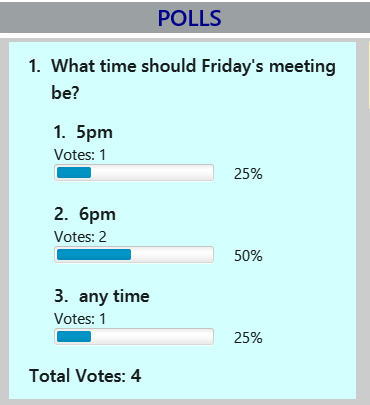
Figure 55. Poll With Results
Since viewing and hiding results of polls are similar in implementation, only viewing results of polls will be discussed.
When an Exco
member inputs 'viewresults', the sequence diagram (Figure 56) below shows how the different components interact
to post a ViewResultsRequestEvent
.
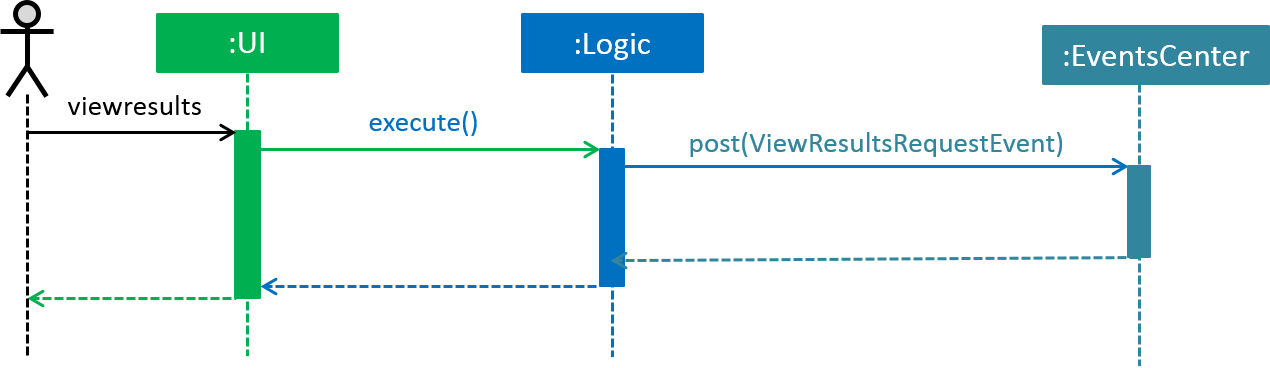
Figure 56. Sequence Diagram to EventsCenter
for viewing poll results .
Then, the PollListPanel
which contains the poll ListView
, will handle the event (Figure 57) by showing results of results of all polls (Figure 46).
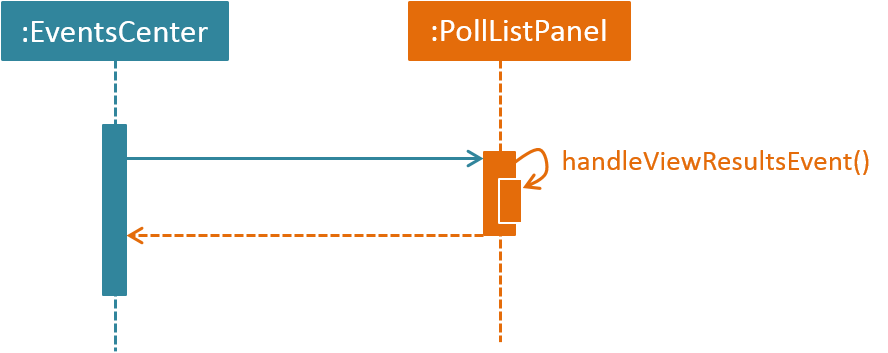
Figure 57. Sequence Diagram from EventsCenter
for viewing poll results.
The following code snippet shows the implementation:
@Subscribe
private void handleViewResultsEvent(ViewResultsRequestEvent event) {
logger.info(LogsCenter.getEventHandlingLogMessage(event));
showPollResults();
}
Hiding results of polls will be done in a similar manner except HideResultsRequestEvent
is posted and handled instead
of ViewResultsRequestEvent
.
4.5.2. Design Considerations
Aspect: Keeping track of how many members voted for each answer of a poll and who has voted in a poll
-
Alternative 1 (current choice): Make each
Answer
keep track of how many votes it has received and eachPoll
keep track of who has voted in it using a Set<MatricNumber>-
Pros: Voters are not tied to any specific answer hence ensuring anonymity
-
Cons: Harder to ensure sum of vote count of all
Answer`s in a `Poll
must equal to size of Set<MatricNumber> in the poll
-
-
Alternative 2: Make each
Answer
contain a Set<MatricNumber>. It’s size is the number of voters and to keep track of who voted, check user’sMatricNumber
with allMatricNumber
in allAnswer
of aPoll
-
Pros: Easy to implement
-
Cons: No anonymity since information of who voted for which Answer is stored inside Set<MatricNumber> of an
Answer
-
4.6. Delete Group Mechanism
4.6.1. Current Implementation
The deletegroup
mechanism is facilitated by the DeleteGroupCommand
class. It allows Exco
members to delete a group from Club Connect
. The group of all members part of the group that is to be deleted will be changed to the default group - member
.
The DeleteGroupCommand
extends from UndoableCommand
as it is an undoable command. Figure 58 below depicts the UML representation of the Command
.
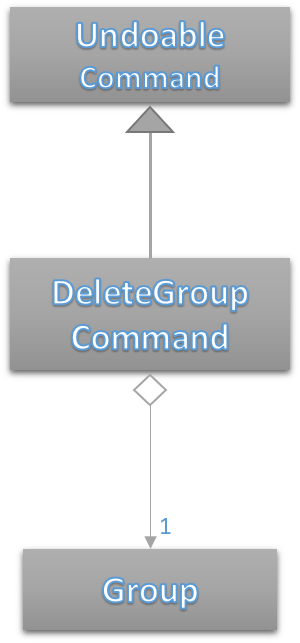
Figure 58. UML Diagram of DeleteGroupCommand
.
DeleteGroupCommandParser
is responsible for parsing the deletegroup
command. It returns a DeleteGroupCommand
object after parsing the Group
. The parsing of the command is shown below in Figure 59.
The method where the deletion takes place in each member is ClubBook#deleteGroupFromMember
.
private void deleteGroupFromMember(Group toRemove, Member member)
throws MemberNotFoundException {
if (!member.getGroup().toString().equalsIgnoreCase(toRemove.toString())) {
return;
}
Group defaultGroup = new Group(Group.DEFAULT_GROUP);
Member newMember = new Member(member.getName(), member.getPhone(), member.getEmail(), member.getMatricNumber(),
defaultGroup, member.getTags());
try {
updateMember(member, newMember);
} catch (DuplicateMatricNumberException dme) {
throw new AssertionError("Deleting a member's group only should not result in a duplicate. "
+ "See member#equals(Object).");
}
}
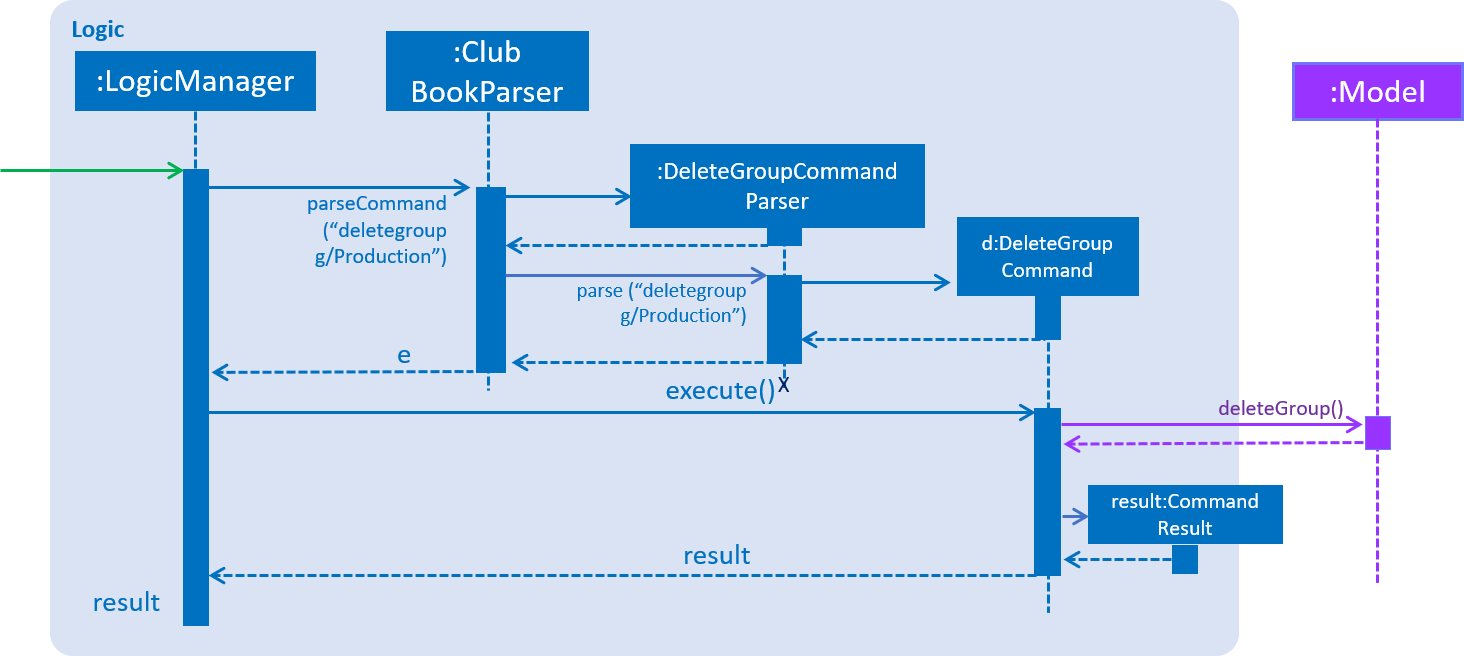
Figure 59. Sequence Diagram for the parsing of DeleteGroupCommand
.
The high-level sequence of events is depicted in Figure 60 below.

Figure 60. Sequence Diagram for delete a group from Club Connect
.
4.6.2. Design Considerations
Aspect: Implementation of DeleteGroupCommand
-
Alternative 1 (current choice): Overwrite the relevant
Member
objects with newMember
objects.-
Pros: Easy to implement.
-
Cons: Requires looping through all the members in
Club Connect
.
-
-
Alternative 2: To maintain a
UniqueGroupList
-
Pros: Cleaner implementation.
-
Cons: Relatively difficult to implement.
-
4.7. Importing Members
4.7.1. Current Implementation
The import
mechanism of Club Connect is facilitated by the ImportCommand
class and is event-driven.
It allows members to add the list of members from a CSV file to Club Connect at one go.
To facilitate this, it makes use of the CsvUtil
class, which converts Member
objects to CSV format.
The ImportCommand
extends from the UndoableCommand
class, and only consists of a File
attribute.
This is illustrated in the UML diagram shown in Figure 61.
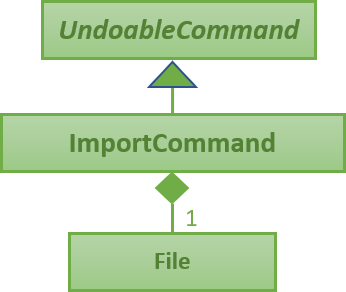
Figure 61. ImportCommand UML Diagram
The import
command involves the use of multiple components of the Club Connect application.
Figure 62 (below) shows the high-level Sequence Diagram for the import
command.
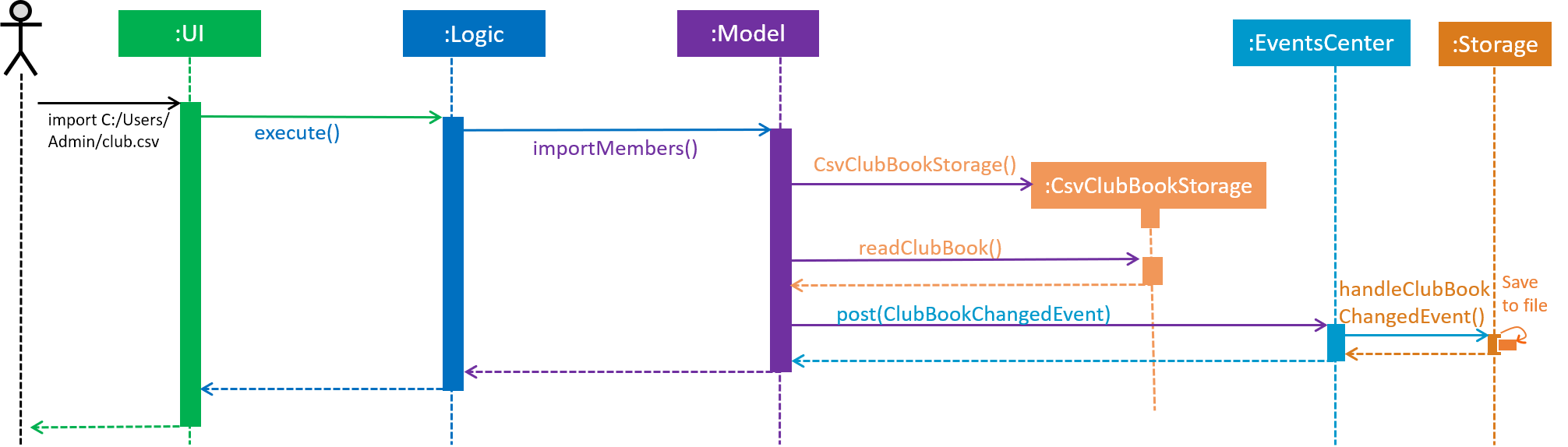
Figure 62. High Level Sequence Diagram for the import C:/Users/Admin/club.csv
command
Logic
is responsible for parsing the import
command.
It returns an ImportCommand
object after parsing the CSV file path.
The ImportCommand#execute()
method invokes the importMembers()
method from model
.
The Model
component invokes the readClubBook()
method of the CsvClubBookStorage
object to get the list of Member
objects that are to be added to Club Connect. The members from the list are then sequentially added to the club book.
If a DuplicateMatricNumberException
is encountered while adding a member, then that member is not added to the club book
and execution continues. This way, even if there are a few members with invalid data values, the import command will
still work for those members with valid data. This implementation is shown in the code snipped below:
public int importMembers(File importFile) throws IOException {
CsvClubBookStorage csvStorage = new CsvClubBookStorage(importFile);
UniqueMemberList importedMembers = csvStorage.readClubBook();
int numberMembers = 0;
for (Member member: importedMembers) {
try {
clubBook.addMember(member);
numberMembers++;
} catch (DuplicateMatricNumberException dmne) {
//...logging...
}
}
indicateClubBookChanged();
return numberMembers;
}
The conversion of data from the CSV file to Member
objects is facilitated by the CsvUtil
class. Part of the implementation is shown here:
public static UniqueMemberList getDataFromFile(File file) throws IOException {
UniqueMemberList importedMembers = new UniqueMemberList();
String data = FileUtil.readFromFile(file);
String[] membersData = data.split("\n");
for (int i = 1; i < membersData.length; i++) { //membersData[0] contains column headers
try {
Member member = getMember(membersData[i]);
importedMembers.add(member);
} catch (Exception e) {
//...logging...
}
}
return importedMembers;
}
4.7.2. Design Considerations
Aspect: Implementation of ImportCommand
-
Alternative 1 (current choice): Implement parser for CSV files ourselves
-
Pros: Greater control over what the format in the file to be imported from should be
-
Cons: Harder to implement, less flexible in terms of how data can be stored in the file as the data values are not binded to tags
-
-
Alternative 2: Use existing libraries (such as OpenCSV and Jackson)
-
Pros: More flexible in terms of how the data can be stored in the import file
-
Cons: Security issues or bugs in the external library cannot be fixed, licensing issues, difficult to use due to poor documentation
-
Aspect: Input file format
-
Alternative 1 (current choice): Import from a CSV file
-
Pros: Easier for users to create and modify their data in a CSV file
-
Cons: Implementation becomes more complex and may become less robust as support for parsing data from CSV files has to be added.
-
-
Alternative 2: Import from a XML file
-
Pros: Easier to implement and parse data, as the code already supports the reading of data from XML format
-
Cons: Harder for non-technical users to create and modify their data in a XML file
-
4.8. Exporting Members
4.8.1. Current Implementation
The export
mechanism of Club Connect is facilitated by the ExportCommand
class and is event-driven.
It allows members to export the list of members in Club Connect as a CSV file.
It makes use of the CsvUtil
class to convert Member
objects to CSV format.
The ExportCommand
extends from the Command
class, as it is not an undoable command and only consists of a File
attribute.
Figure 63 depicts the UML diagram for the ExportCommand
class.
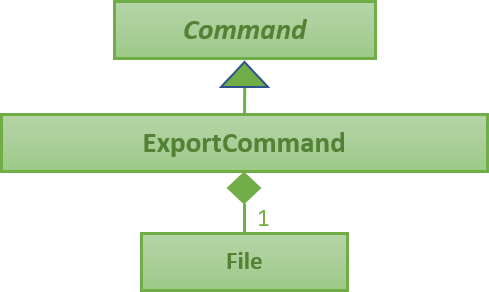
Figure 63. ExportCommand UML Diagram
The export
command uses multiple components of the Club Connect application.
The Sequence Diagram below (Figure 64) shows the interactions between some of these components.
As you can see, the ExportCommand
is driven by the NewExportDataAvailableEvent
.
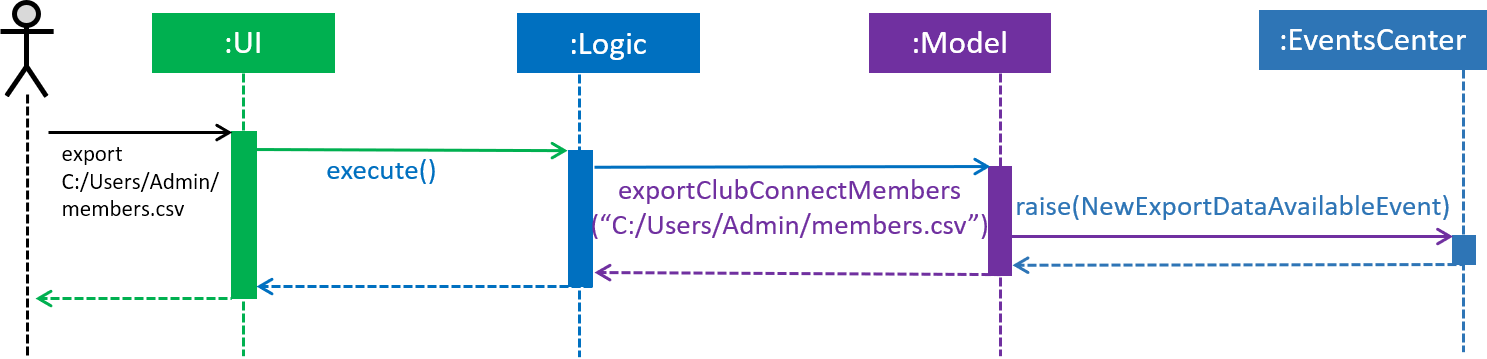
Figure 64. High Level Sequence Diagram for the export C:/Users/Admin/members.csv
command
This event is handled by the Storage
component as shown in Figure 65.
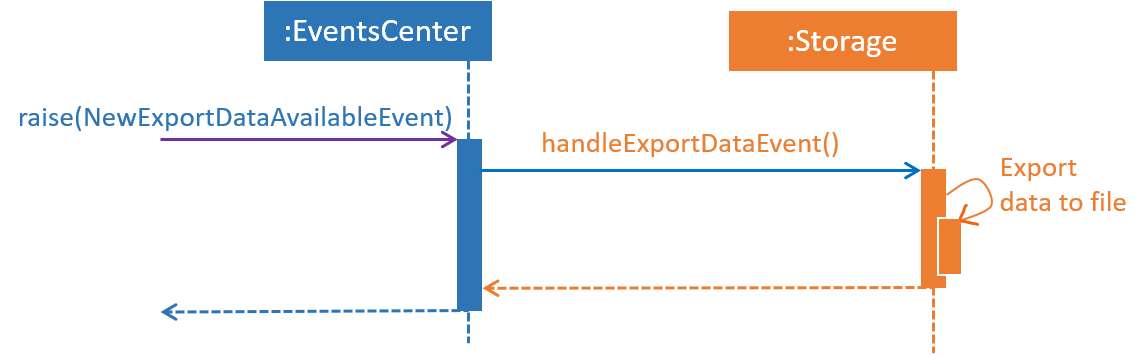
_Figure 65. High Level Sequence Diagram showing how the Storage
component handles the NewExportDataAvailableEvent
Storage
makes use of a CsvClubBookStorage
object to write data to the file specified by the export path.
This is shown in the code snippet below:
public void exportDataToFile(String data, File exportFile) throws IOException {
csvClubBookStorage.setClubBookFile(exportFile);
// ... logging ...
csvClubBookStorage.saveData(data);
}
Then, the csvClubBookStorage
object gets the column headers for the file from the CsvUtil
class:
public void saveData(String data, File file) throws IOException {
// ... logging ...
String columnHeaders = CsvUtil.getHeaders();
String dataToExport = columnHeaders + data;
CsvFileStorage.saveDataToFile(file, dataToExport);
}
Finally, the CsvFileStorage
class saves the data to the file by using the writeToFile
method from the FileUtil
class.
public static void saveDataToFile(File file, String data) throws IOException {
FileUtil.writeToFile(file, data);
}
4.8.2. Design Considerations
Aspect: Output file format
-
Alternative 1 (current choice): Export to a CSV file
-
Pros: More user-friendly in terms of understandability, generated file is compatible with the
import
command -
Cons: Harder to implement as support for converting data of
Member
to CSV format needs to be added
-
-
Alternative 2: Export to a XML file
-
Pros: Easier to implement and as the code already supports the writing of data in XML format
-
Cons: Raw file provides a less intuitive view of data, generated file is not compatible with the
import
command
-
4.9. Changing Profile Photo Mechanism
4.9.1. Current Implementation
The changepic
mechanism of Club Connect is facilitated by the ChangeProfilePhotoCommand
class and is event-driven.
It allows members to modify their profile photos displayed in the application.
To facilitate this, it makes use of the ProfilePhoto
class.
Currently, the ChangeProfilePhotoCommand
extends from the Command
class, and not from UndoableCommand
.
Refer to Figure 66 for the UML diagram.
The ProfilePhoto
class consists of a String
attribute to store the file path of the profile photo.
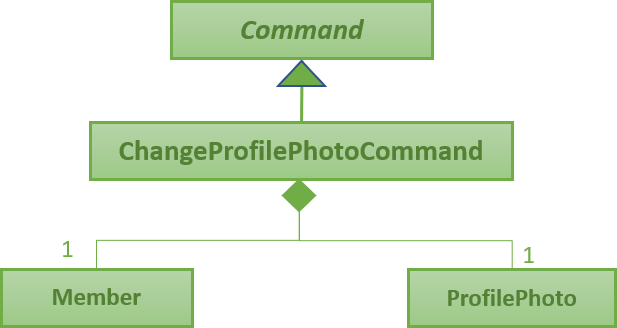
Figure 66. ChangeProfilePhotoCommand UML Diagram
The displaypic
command involves the use of multiple components of Club Connect.
Figure 67 (below) shows the interactions between these components.
As you can see, the ChangeProfilePhotoCommand
is driven by the ProfilePhotoChangedEvent
.
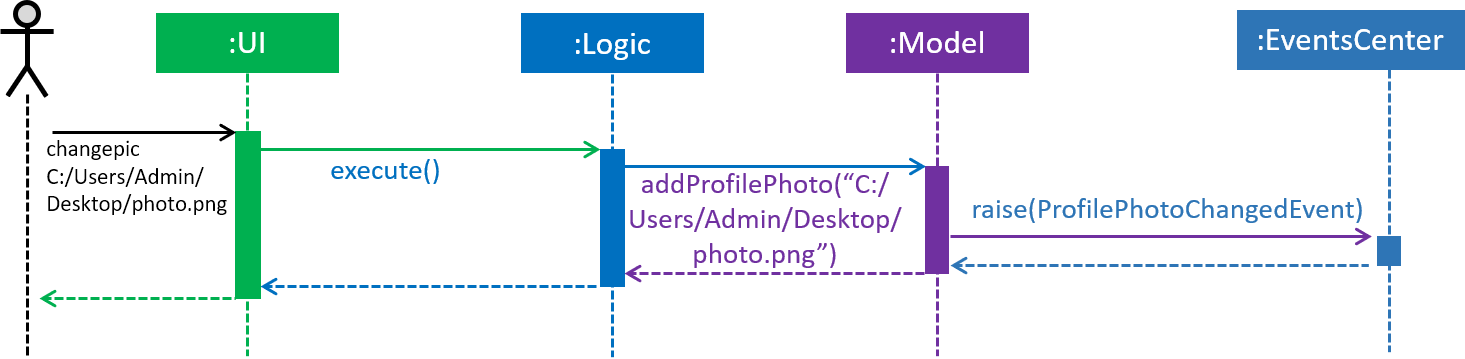
Figure 67. High Level Sequence Diagram for the changepic C:/Users/Admin/Desktop/photo.png
command
ChangeProfilePhotoCommandParser is responsible for parsing the changepic
command.
It returns a ChangeProfilePhotoCommand
object after parsing the photo file path.
Figure 68 depicts the Sequence Diagram for interactions within the Logic
component for the execute("changepic C:/Users/Admin/Desktop/ photo.png")
API call.
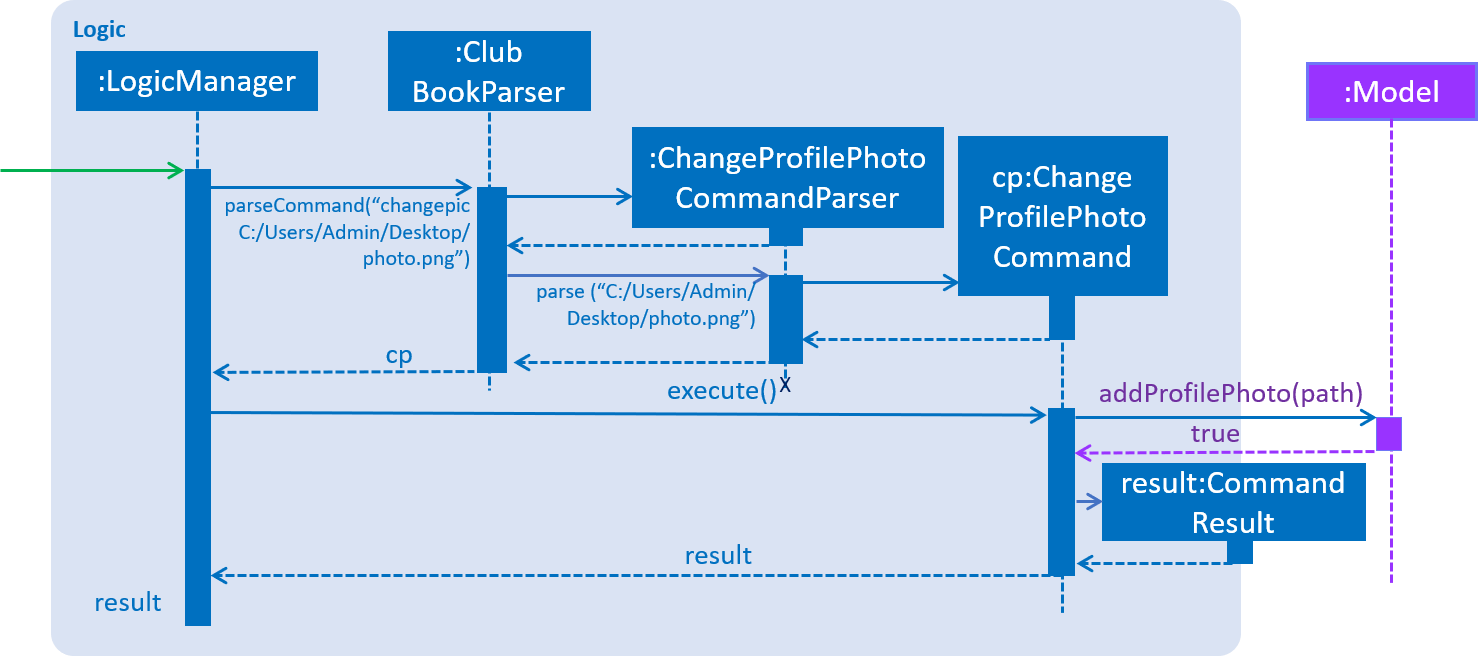
_Figure 68. Interactions Inside the Logic Component for the changepic C:/Users/Admin/Desktop/photo.png
command
The ChangeProfilePhotoCommand#execute()
method invokes the addProfilePhoto()
method from model
.
Complying with the rules of abstraction, the Logic
component calls on Model
to handle the internal details for updation.
The actual reading of the profile photo from the path provided is done by the Storage
component.
It copies the photo as a bitmap image file (.bmp) to the Club Connect application’s resources.
The code used for reading and copying the file is as follows:
@Override
public void copyOriginalPhotoFile(String originalPhotoPath, String newPhotoName) throws PhotoException {
BufferedImage originalPhoto = null;
try {
logger.info("Profile Photo is being read from " + originalPhotoPath);
URL photoUrl = new URL(URL_PREFIX + originalPhotoPath);
newPath = SAVE_PHOTO_DIRECTORY + newPhotoName + PHOTO_FILE_EXTENSION;
InputStream photoStream = photoUrl.openStream();
createPhotoFileCopy(photoStream, newPath);
} catch (IOException ioe) {
// ... exception handling ...
}
}
public void createPhotoFileCopy(InputStream photoStream, String newPath) throws PhotoWriteException {
// ... logging ...
try {
FileUtil.createDirs(new File(SAVE_PHOTO_DIRECTORY));
Files.copy(photoStream, Paths.get(newPath), StandardCopyOption.REPLACE_EXISTING);
} catch (IOException ioe) {
// ... exception handling ...
}
}
The logged in member’s details are then updated to include this new profile photo.
The photo specified by the path is set to the ImageView
object by the following code in the Ui
component:
private ImageView profilePhoto;
private void setProfilePhoto(Member member) {
Image photo;
String photoPath = member.getProfilePhoto().getPhotoPath();
if (photoPath.equals(EMPTY_STRING) || photoPath.equals(DEFAULT_PHOTO_PATH)) {
photo = new Image(MainApp.class.getResourceAsStream(DEFAULT_PHOTO_PATH), photoWidth, photoHeight, false, true);
} else {
photo = new Image("file:" + photoPath, photoWidth, photoHeight, false, true);
}
profilePhoto.setImage(photo);
}
The actual displaying of the profile photo is done by using this fxml code:
<HBox alignment="CENTER_LEFT">
<ImageView fx:id="profilePhoto" fitWidth="100" fitHeight="130">
<HBox.margin>
<Insets left="7.5" bottom="7.5" right="5.0" top="7.5" />
</HBox.margin>
</ImageView>
</HBox>
4.9.2. Design Considerations
Aspect: Implementation of ChangeProfilePhotoCommand
-
Alternative 1 (current choice): Logged in member can only change his/her own profile photo
-
Pros: Easy to implement, makes intuitive sense
-
Cons: There is no way for Exco members to ensure that members have appropriate profile photos
-
-
Alternative 2: Exco members can change any member’s profile photo
-
Pros: Exco members have the ability to exercise control over members' profile photos
-
Cons: Implementation becomes more complicated
-
Aspect: Source files of profile photos
-
Alternative 1 (current choice): Make a copy the source image provided to the applications resources
-
Pros: Application becomes portable and independent from the rest of the system. Members can delete the original file from the computer, without affecting the Club Connect Application
-
Cons: Changes made to the original source images are not reflected in the application
-
-
Alternative 2: Always read the profile photo from the file path provided
-
Pros: Changes made in the source image are reflected in the application
-
Cons: Application becomes highly dependent on the system, in terms of profile photos
-
4.10. Compress/Decompress Mechanism
4.10.1. Current Implementation
Member details are shown as cards in the GUI. Cards can either be decompressed(Figure 69) or compressed(Figure 70).
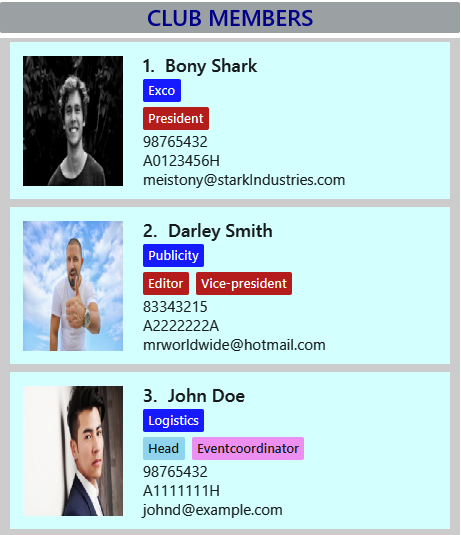
Figure 69. Decompressed Member Cards
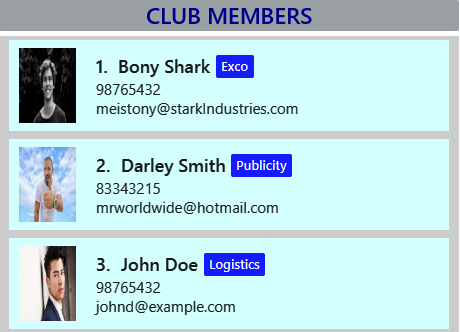
Figure 70. Compressed Member Cards
The MemberCard
class provides an abstraction for the member card shown in the GUI. By default, it shows a member card that is decompressed.
To differentiate between compressed and decompressed member card, we introduced a CompressedMemberCard
class. This class extends MemberCard
(Figure 71) since a CompressedMemberCard
is a MemberCard
.
Each of these classes contain a different static String showing location of the actual FXML
file that determines layout of the member card, hence we get different layouts.
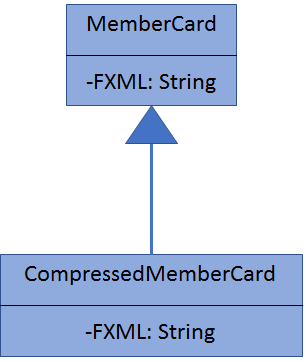
Figure 71. MemberCard
class diagram
The compress
and decompress
commands allows members to select whether they want to see detailed information of members or see a compressed version for easy viewing.
These commands do not extend from UndoableCommand
but just from Command
since they do not change the state of the club book.
Due to the similar nature of the compress
and decompress
commands, only the compress
command will be discussed.
Suppose that the user has just launched the application. The member cards would be decompressed (Figure 69).
The user inputs 'compress' and the sequence diagram (Figure 72) below shows how the different components interact.
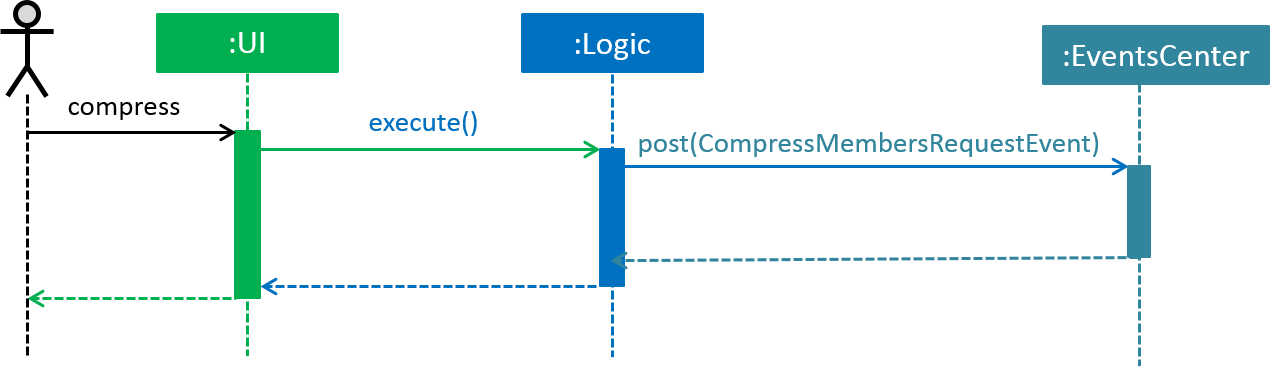
Figure 72. Sequence diagram for compress command to Events Center
Then, the MemberListPanel
, which contains the MemberCard
objects will handle the event (Figure 73). If the cards are already compressed, no changes occur.
However, if the cards are decompressed, the MemberCard
objects would be converted to CompressedMemberCard
objects.
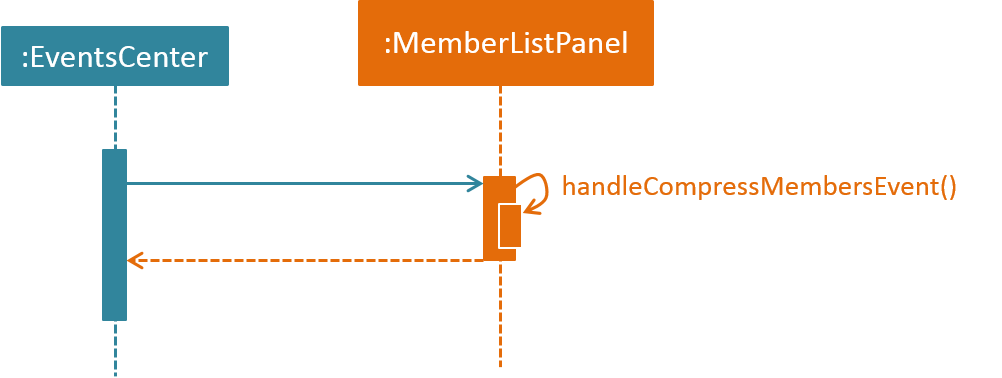
Figure 73. Sequence diagram for compress command from Events Center
4.10.2. Design Considerations
Aspect: How to interact from Logic to Ui
-
Alternative 1 (current choice): Use Event-Driven approach to interact from
CompressCommand
/DecompressCommand
toMemberListPanel
-
Pros: Reduces coupling.
-
Cons: Slower than just directly changing the
Ui
since a seperate class(EventsCenter
) has to manage interactions, not just for compress/decompress but for other events as well.
-
-
Alternative 2: Keep a
Ui
attribute in every command just likeModel
-
Pros: Easy to implement.
-
Cons: Increases coupling
-
4.11. Auto-Complete Command Mechanism
4.11.1. Current Implementation
The auto-complete mechanism is an enhancement added to reduce the frequency pf typos and thus by extension, invalid commands.
It makes use of the key press event KeyEvent
. Pressing
cycles through all possible commands based on user input.TAB
The ArrayList<String> commandList
in CommandList.java
stores all the command formats.
Here is the implementation for handling the key-press event:
private void handleKeyPress(KeyEvent keyEvent) {
switch (keyEvent.getCode()) {
.
.
case TAB:
keyEvent.consume();
completeCommandIndex++;
autoComplete(commandTextField.getText());
break;
.
.
}
}
Shown below is the implementation of the autoComplete
method.
private void autoComplete(String input) {
if (!input.equals("")) {
if (oldInput == null) {
oldInput = input;
} else if (!input.startsWith(oldInput)) {
oldInput = input;
completeCommandIndex = 0;
}
List<String> completedCommands = LogicManager.COMMAND_LIST.stream().filter(s -> s.startsWith(oldInput))
.collect(Collectors.toList());
if (!completedCommands.isEmpty()) {
replaceText(completedCommands.get(completeCommandIndex % completedCommands.size()));
} else {
replaceText("");
}
}
}
4.11.2. Design Considerations
Implementation of cycling through commands
-
Alternative 1 (current choice): Use string manipulation to store old input and only change it if the new input doesn’t start with the old input.
-
Pros: It’s easy to implement.
-
Cons: It’s not so good in terms of code quality.
-
-
Alternative 2: If there are 'N' commands that start with
XXX
, create 'N' strings with each concatenated one after the other in increasing length i.e. XXX1, XXX1XXX2, … ,XXX1XXX2XXX3…XXXN. Keep truncating the commands not needed from the front.-
Pros: Possibly cleaner code.
-
Cons: It’s relatively hard to implement.
-
4.12. [Proposed] Data Encryption
4.12.1. Rationale
Data encryption is key to any App that deals with personal data of individuals.
We plan to use Symmetric Key Encryption to ensure the confidentiality of data.
4.12.2. How Symmetric Key Encryption works
Symmetric key encryption is an encryption philosophy where the two communicating parties share a pre-established secret key k.
It consists of 2 algorithms E
(Encrypting or Encoding) and D
(Decryption or Decoding) which take in the same key k to perform their respective operations.
The 2 algorithms E
and D
are efficient algorithms, such that:
-
D(E(k,m)) = m, where 'm' is the message that needs to be kept confidential.
-
For k chosen uniformly at random, E(k,m) gives no additional information about 'm' to an adversary.
4.12.3. Proposed Implementation
We plan to make use of classes that are defined in Javax’s Crypto package.
The classes that would feature in the implementation are:
-
KeyGenerator → constructs a secret (symmetric) key.
-
Cipher → provides the functionality of a cryptographic cipher for encryption and decryption.
The construction of a symmetric key is done by passing the algorithm the encryption will use. The Advanced Encryption Scheme (AES) algorithm is a secure encryption algorithm that has proven been proven secure. A 128-bit AES symmetric key will be used to encrypt all data files.
Once the key is generated, all data will be encrypted with AES. Anyone who wishes to view the decrypted form of the data must possess the secret key.
Figure 74 below describes the encryption procedure used in AES – specifically, the Cipher Block Chaining (CBC) mode.
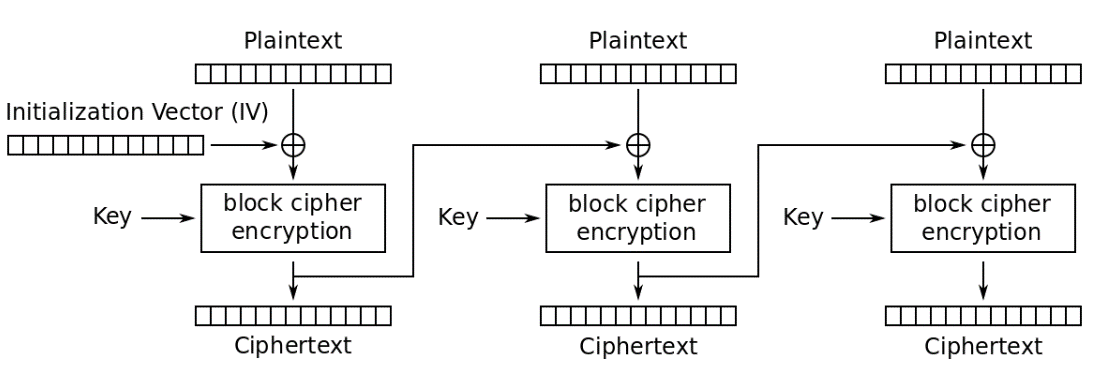
Figure 74. AES – CBC mode encryption.
Figure 75 below shows the decryption process.
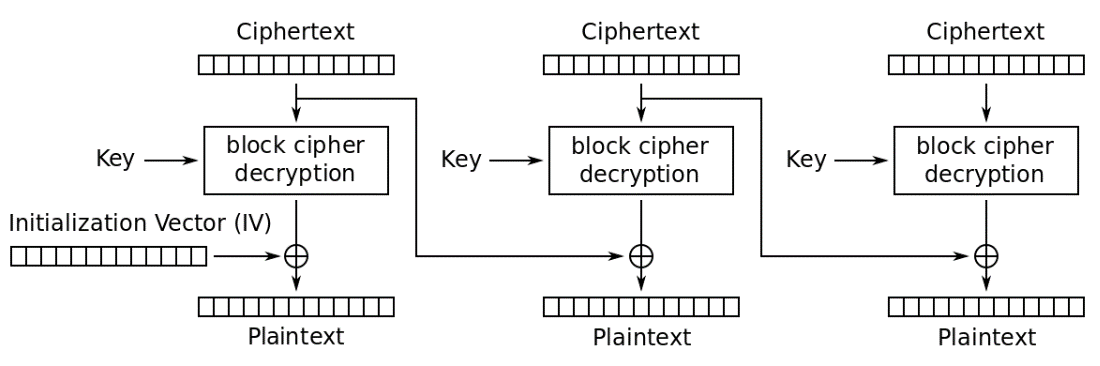
Figure 75. AES – CBC mode decrption.
4.13. Logging
We are using java.util.logging
package for logging.
The LogsCenter
class is used to manage the logging levels and logging destinations.
-
The logging level can be controlled using the
logLevel
setting in the configuration file (See Section 4.14, “Configuration”). -
The
Logger
for a class can be obtained usingLogsCenter.getLogger(Class)
which will log messages according to the specified logging level. -
Currently log messages are output through:
Console
and to a.log
file.
Logging Levels
-
SEVERE
: Critical problem detected which may possibly cause the termination of the application. -
WARNING
: Can continue, but with caution. -
INFO
: Information showing the noteworthy actions by the App. -
FINE
: Details that is not usually noteworthy but may be useful in debugging e.g. print the actual list instead of just its size.
4.14. Configuration
Certain properties of the application can be controlled (e.g App name, logging level) through the configuration file (default: config.json
).
5. Documentation
We use asciidoc for writing documentation.
We chose asciidoc over Markdown because asciidoc, although a bit more complex than Markdown, provides more flexibility in formatting. |
5.1. Editing Documentation
See UsingGradle.adoc to learn how to render .adoc
files locally to preview the end result of your edits.
Alternatively, you can download the AsciiDoc plugin for IntelliJ, which allows you to preview the changes you have made to your .adoc
files in real-time.
5.2. Publishing Documentation
See UsingTravis.adoc to learn how to deploy GitHub Pages using Travis.
5.3. Converting Documentation to PDF format
We use Google Chrome for converting documentation to PDF format, as Chrome’s PDF engine preserves hyperlinks used in webpages.
Here are the steps to convert the project documentation files to PDF format.
-
Follow the instructions in UsingGradle.adoc to convert the AsciiDoc files in the
docs/
directory to HTML format. -
Go to your generated HTML files in the
build/docs
folder, right click on them and selectOpen with
→Google Chrome
. -
Within Chrome, click on the
Print
option in Chrome’s menu. -
Set the destination to
Save as PDF
, then clickSave
to save a copy of the file in PDF format. For best results, use the settings in Figure 76 below.
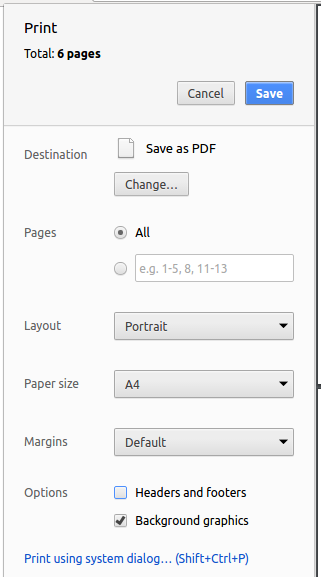
Figure 76. Screenshot of saving documentation as PDF in Chrome
6. Testing
6.1. Running Tests
There are three ways to run tests.
The most reliable way to run tests is the 3rd one. The first two methods might fail some GUI tests due to platform/resolution-specific idiosyncrasies. |
Method 1: Using IntelliJ JUnit test runner
-
To run all tests, right-click on the
src/test/java
folder and chooseRun 'All Tests'
-
To run a subset of tests, you can right-click on a test package, test class, or a test and choose
Run 'ABC'
Method 2: Using Gradle
-
Open a console and run the command
gradlew clean allTests
(Mac/Linux:./gradlew clean allTests
)
See UsingGradle.adoc for more info on how to run tests using Gradle. |
Method 3: Using Gradle (headless)
Thanks to the TestFX library we use, our GUI tests can be run in the headless mode. In the headless mode, GUI tests do not show up on the screen. That means the developer can do other things on the Computer while the tests are running.
To run tests in headless mode, open a console and run the command gradlew clean headless allTests
(Mac/Linux: ./gradlew clean headless allTests
)
6.2. Types of tests
We have two types of tests:
-
GUI Tests - These are tests involving the GUI. They include,
-
System Tests that test the entire App by simulating user actions on the GUI. These are in the
systemtests
package. -
Unit tests that test the individual components. These are in
seedu.club.ui
package.
-
-
Non-GUI Tests - These are tests not involving the GUI. They include,
-
Unit tests targeting the lowest level methods/classes.
e.g.seedu.club.commons.StringUtilTest
-
Integration tests that are checking the integration of multiple code units (those code units are assumed to be working).
e.g.seedu.club.storage.StorageManagerTest
-
Hybrids of unit and integration tests. These test are checking multiple code units as well as how the are connected together.
e.g.seedu.club.logic.LogicManagerTest
-
6.3. Troubleshooting Testing
Problem: HelpWindowTest
fails with a NullPointerException
.
-
Reason: One of its dependencies,
UserGuide.html
insrc/main/resources/docs
is missing. -
Solution: Execute Gradle task
processResources
.
7. Dev Ops
7.1. Build Automation
See UsingGradle.adoc to learn how to use Gradle for build automation.
7.2. Continuous Integration
We use Travis CI and AppVeyor to perform Continuous Integration on our projects. See UsingTravis.adoc and UsingAppVeyor.adoc for more details.
7.3. Coverage Reporting
We use Coveralls to track the code coverage of our projects. See UsingCoveralls.adoc for more details.
7.4. Documentation Previews
When a pull request has changes to asciidoc files, you can use Netlify to see a preview of how the HTML version of those asciidoc files will look like when the pull request is merged. See UsingNetlify.adoc for more details.
7.5. Making a Release
Here are the steps to create a new release.
-
Update the version number in
MainApp.java
. -
Generate a JAR file using Gradle.
-
Tag the repo with the version number. e.g.
v0.1
-
Create a new release using GitHub and upload the JAR file you created.
7.6. Managing Dependencies
A project often depends on third-party libraries. For example, Club Book depends on the Jackson library for XML parsing. Managing these dependencies can be automated using Gradle. For example, Gradle can download the dependencies automatically, which is better than these alternatives.
a. Include those libraries in the repo (this bloats the repo size)
b. Require developers to download those libraries manually (this creates extra work for developers)
Appendix A: Suggested Programming Tasks to Get Started
Suggested path for new programmers:
-
First, add small local-impact (i.e. the impact of the change does not go beyond the component) enhancements to one component at a time. Some suggestions are given in Section A.1, “Improving each component”.
-
Next, add a feature that touches multiple components to learn how to implement an end-to-end feature across all components. Section A.2, “Creating a new command:
remark
” explains how to go about adding such a feature.
A.1. Improving each component
Each individual exercise in this section is component-based (i.e. you would not need to modify the other components to get it to work).
Logic
component
Scenario: You are in charge of logic
. During dog-fooding, your team realize that it is troublesome for the user to type the whole command in order to execute a command.
Your team devise some strategies to help cut down the amount of typing necessary, and one of the suggestions was to implement aliases for the command words.
Your job is to implement such aliases.
Do take a look at Section 3.3, “Logic component” before attempting to modify the Logic component.
|
-
Add a shorthand equivalent alias for each of the individual commands. For example, besides typing
clear
, the user can also typec
to remove all members in the list.
Model
component
Scenario: You are in charge of model
. One day, the logic
-in-charge approaches you for help. He wants to implement a command such that the user is able to remove a particular tag from everyone in the address book, but the model API does not support such a functionality at the moment. Your job is to implement an API method, so that your teammate can use your API to implement his command.
Do take a look at Section 3.4, “Model component” before attempting to modify the Model component.
|
-
Add a
removeTag(Tag)
method. The specified tag will be removed from everyone in the address book.
Ui
component
Scenario: You are in charge of ui
. During a beta testing session, your team is observing how the users use your address book application. You realize that one of the users occasionally tries to delete non-existent tags from a contact, because the tags all look the same visually, and the user got confused. Another user made a typing mistake in his command, but did not realize he had done so because the error message wasn’t prominent enough. A third user keeps scrolling down the list, because he keeps forgetting the index of the last member in the list. Your job is to implement improvements to the UI to solve all these problems.
Do take a look at Section 3.2, “UI component” before attempting to modify the UI component.
|
-
Use different colors for different tags inside member cards. For example,
friends
tags can be all in brown, andcolleagues
tags can be all in yellow as shown in Figure 78.Figure 77 below shows the member details before the modification.
Before
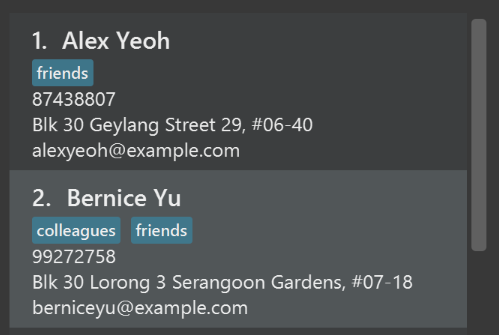
Figure 77. Member details before modification
Figure 78 below shows the member details after the modification.
After
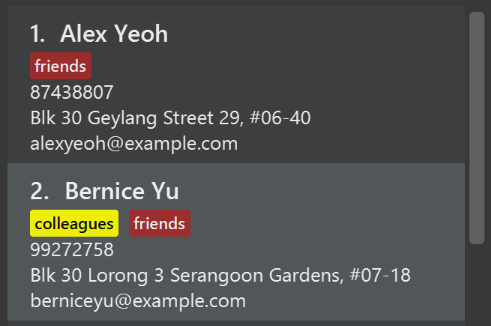
Figure 78. Member details after modification
-
Modify
NewResultAvailableEvent
such thatResultDisplay
can show a different style on error (currently it shows the same regardless of errors).Figure 79 below shows the
ResultDisplay
before the modification.
BeforeFigure 79. ResultDisplay before modification
After
Figure 80 below shows the
ResultDisplay
after the modification.Figure 80. ResultDisplay after modification
-
Modify the
StatusBarFooter
to show the total number of people in the address book.Figure 81 below shows the
StatusBarFooter
before the modification.Before
Figure 81. StatusBarFooter before modification+ Figure 82 below shows the
StatusBarFooter
after the modification.After
Figure 82. StatusBarFooter after modification
Storage
component
Scenario: You are in charge of storage
. For your next project milestone, your team plans to implement a new feature of saving the address book to the cloud. However, the current implementation of the application constantly saves the address book after the execution of each command, which is not ideal if the user is working on limited internet connection. Your team decided that the application should instead save the changes to a temporary local backup file first, and only upload to the cloud after the user closes the application. Your job is to implement a backup API for the address book storage.
Do take a look at Section 3.5, “Storage component” before attempting to modify the Storage component.
|
-
Add a new method
backupAddressBook(ReadOnlyAddressBook)
, so that the address book can be saved in a fixed temporary location.
A.2. Creating a new command: remark
By creating this command, you will get a chance to learn how to implement a feature end-to-end, touching all major components of the app.
Scenario: You are a software maintainer for addressbook
, as the former developer team has moved on to new projects. The current users of your application have a list of new feature requests that they hope the software will eventually have. The most popular request is to allow adding additional comments/notes about a particular contact, by providing a flexible remark
field for each contact, rather than relying on tags alone. After designing the specification for the remark
command, you are convinced that this feature is worth implementing. Your job is to implement the remark
command.
A.2.1. Description
Edits the remark for a member specified in the INDEX
.
Format: remark INDEX r/[REMARK]
Examples:
-
remark 1 r/Likes to drink coffee.
Edits the remark for the first member toLikes to drink coffee.
-
remark 1 r/
Removes the remark for the first member.
A.2.2. Step-by-step Instructions
[Step 1] Logic: Teach the app to accept 'remark' which does nothing
Let’s start by teaching the application how to parse a remark
command. We will add the logic of remark
later.
Main:
-
Add a
RemarkCommand
that extendsUndoableCommand
. Upon execution, it should just throw anException
. -
Modify
AddressBookParser
to accept aRemarkCommand
.
Tests:
-
Add
RemarkCommandTest
that tests thatexecuteUndoableCommand()
throws an Exception. -
Add new test method to
AddressBookParserTest
, which tests that typing "remark" returns an instance ofRemarkCommand
.
[Step 2] Logic: Teach the app to accept 'remark' arguments
Let’s teach the application to parse arguments that our remark
command will accept. E.g. 1 r/Likes to drink coffee.
Main:
-
Modify
RemarkCommand
to take in anIndex
andString
and print those two parameters as the error message. -
Add
RemarkCommandParser
that knows how to parse two arguments, one index and one with prefix 'r/'. -
Modify
AddressBookParser
to use the newly implementedRemarkCommandParser
.
Tests:
-
Modify
RemarkCommandTest
to test theRemarkCommand#equals()
method. -
Add
RemarkCommandParserTest
that tests different boundary values forRemarkCommandParser
. -
Modify
AddressBookParserTest
to test that the correct command is generated according to the user input.
[Step 3] Ui: Add a placeholder for remark in PersonCard
Let’s add a placeholder on all our PersonCard
s to display a remark for each member later.
Main:
-
Add a
Label
with any random text insidePersonListCard.fxml
. -
Add FXML annotation in
PersonCard
to tie the variable to the actual label.
Tests:
-
Modify
PersonCardHandle
so that future tests can read the contents of the remark label.
[Step 4] Model: Add Remark
class
We have to properly encapsulate the remark in our Person
class. Instead of just using a String
, let’s follow the conventional class structure that the codebase already uses by adding a Remark
class.
Main:
-
Add
Remark
to model component (you can copy fromAddress
, remove the regex and change the names accordingly). -
Modify
RemarkCommand
to now take in aRemark
instead of aString
.
Tests:
-
Add test for
Remark
, to test theRemark#equals()
method.
[Step 5] Model: Modify Person
to support a Remark
field
Now we have the Remark
class, we need to actually use it inside Person
.
Main:
-
Add
getRemark()
inPerson
. -
You may assume that the user will not be able to use the
add
andedit
commands to modify the remarks field (i.e. the member will be created without a remark). -
Modify
SampleDataUtil
to add remarks for the sample data (delete yourclubBook.xml
so that the application will load the sample data when you launch it.)
[Step 6] Storage: Add Remark
field to XmlAdaptedPerson
class
We now have Remark
s for Person
s, but they will be gone when we exit the application. Let’s modify XmlAdaptedPerson
to include a Remark
field so that it will be saved.
Main:
-
Add a new Xml field for
Remark
.
Tests:
-
Fix
invalidAndValidPersonAddressBook.xml
,typicalPersonsClubBook.xml
,validAddressBook.xml
etc., such that the XML tests will not fail due to a missing<remark>
element.
[Step 6b] Test: Add withRemark() for PersonBuilder
Since Person
can now have a Remark
, we should add a helper method to PersonBuilder
, so that users are able to create remarks when building a Person
.
Tests:
-
Add a new method
withRemark()
forPersonBuilder
. This method will create a newRemark
for the member that it is currently building. -
Try and use the method on any sample
Person
inTypicalPersons
.
[Step 7] Ui: Connect Remark
field to PersonCard
Our remark label in PersonCard
is still a placeholder. Let’s bring it to life by binding it with the actual remark
field.
Main:
-
Modify
PersonCard
's constructor to bind theRemark
field to thePerson
's remark.
Tests:
-
Modify
GuiTestAssert#assertCardDisplaysPerson(…)
so that it will compare the now-functioning remark label.
[Step 8] Logic: Implement RemarkCommand#execute()
logic
We now have everything set up… but we still can’t modify the remarks. Let’s finish it up by adding in actual logic for our remark
command.
Main:
-
Replace the logic in
RemarkCommand#execute()
(that currently just throws anException
), with the actual logic to modify the remarks of a member.
Tests:
-
Update
RemarkCommandTest
to test that theexecute()
logic works.
A.2.3. Full Solution
See this PR for the step-by-step solution.
Appendix B: Product Scope
Club Connect is targeted at Student Organizations, such as clubs and societies, that are characterized by a well-established hierarchy. Student organizations can have hundreds of members, who are divided into sub-committees that have narrower focuses. These subcommittee members are often assigned individual and group tasks. With time, it becomes very difficult to keep track of everything manually. This is why these organizations require a system for enrolling members, delegating tasks, organising events, and opening polls and getting feedback. Club Connect provides student organisations with a one-stop shop for all their managerial and organisation needs.
Target user profile:
-
Exco member of a Club
-
Member of a club
Value proposition: clubs can efficiently manage its activities and members faster than a typical mouse/GUI driven application
Feature Contribution:
-
Yash Chowdhary
-
Major Feature: Task Management
Each member of a student club / organization is responsible for carrying out tasks that are assigned to him/her. Managing tasks encompasses adding tasks or maintaining a To-Do List for yourself, being assigned tasks by Exco members, removing a task from the list once it has been completed, and updating a task’s status. This ensures transparency and accountability of the club.
-
Minor Feature: Email Command + Auto-Complete + Group Management
-
Every student club / organisation makes use of email blasts to communicate with its members. Whether it is for general communication or for club-related events, the ability to email members is essential to the smooth functioning of an organization.
-
In situations where it is quite tedious to enter all of the parameters of a command, the auto-complete feature is a life-saver. Designed to reduce the chances of entering an invalid command format, it also comes to the aid of forgetful users of the application.
-
Every student club/organisation is sub-divided into sub-committees - each with a different set of responsibilities.
Groups
inClub Connect
emulate this. Furthermore, a club should be given the option to do as it pleases with its sub-committees - rename them, shuffle members, and even remove the sub-committee if need be.
-
-
-
Amrut Prabhu
-
Major Feature: Importing and Exporting Members List
After recruitment events like SLF at NUS or other situations in which details of incoming members are recorded on an Excel sheet, the data can be imported from the Club Connect application instead of manually adding all the members. The list of members from the Club Connect application can be exported to formats such as .csv or .txt so that the club members can have access to a readable and sharable version of the member list outside the Club Connect application.
-
Minor Feature: Profile photo
All the members of a club are not known by each other, which is especially the case in larger clubs. Adding a profile photo makes it easier to know what other members look like and to differentiate between members with similar names.
-
-
Muhammad Nur Kamal Bin Mohammed Ariff
-
Major Feature: Polling system
Exco members can create polls and look at the results to help ascertain the needs and wants of other members. Members can vote for polls to express what they require from the club.
-
Minor Feature: Improve navigability of member list by adding compress/decompress mechanism and revamping
Find
commandUsers can use compress command to clear clutter from member information in member list. Users can also use decompress command to see more information about members in member list.
Find
command can now search for members by partial matches, search by specified field (e.g. by email) or just search by all fields.
-
-
Song Weiyang
-
Major Feature: Log In Function
Members can log in to their own accounts and excess their unique storage files. This can allow allocation of task to certain members and memebrs can organise their datas uniquely.
-
Minor Feature: AutoSorting
After Members edited their list of contacts, the contact list will be automatically sorted in alphabetical order.
-
Appendix C: User Stories
Priorities: High (must have) - * * *
, Medium (nice to have) - * *
, Low (unlikely to have) - *
Priority | As a/an … | I want to … | So that I can… |
---|---|---|---|
|
New member |
see usage instructions |
refer to instructions when I forget how to use the App |
|
add a new member to the club |
begin assigning groups and tasks to the member |
|
|
Exco member |
delete a member from the club |
remove entries that the club no longer needs |
|
Exco member |
divide members into groups using tags |
manage the committee members more efficiently |
|
Exco member |
remove a particular tag/group |
remove redundancies or remove irrelevant tags |
|
Exco member |
remove a member from a particular group |
keep the App up-to-date, and also take into account students that opt-out or graduate |
|
Exco member |
add a task for certain members |
assign members to do a task |
|
Exco member |
view the status of a task |
see the progress made towards completing the task |
|
Exco member |
remove a task |
delete tasks that have been completed |
|
Exco member |
create a poll |
get the opinions of the club members |
|
Exco member who created a poll |
view results of the poll |
see the opinions of the members |
|
Member |
edit my contact details |
keep my contact information updated |
|
Member |
undo my command |
reverse any undesirable commands or mistakes |
|
Member |
redo a command |
return to the state before I undid a command |
|
Member |
select a member |
view the member’s details |
|
Member |
find a member by name |
locate details of members without having to go through the entire list |
|
Member |
find members by a specified field |
locate details of members without having to go through the entire list |
|
Member |
get notifications for new tasks |
be aware of new tasks assigned to me |
|
Member |
add a profile photo |
be identified by others |
|
Member |
add multiple entries for attributes |
provide all alternative contact details to others |
|
Member |
logout of the application |
maintain integrity of my data and actions |
|
Member |
login to my account |
access my data |
|
Member |
vote in a poll |
provide my opinions |
|
Member |
remove a task assigned to me |
focus on the yet to be done tasks |
|
Busy member |
be able to directly email members using my default mail client |
save time and reduce errors by not needing to add recipients myself |
|
Member |
export members' info |
so that I can share the details easily |
|
Exco member |
import data |
add members' info to Club Connect efficiently |
|
Member |
hide private contact details by default |
minimize chance of someone else seeing them by accident |
|
Member |
be notified on members' birthdays |
wish them on time |
|
Member |
compress the display of members |
browse through the list of members with less distractions |
|
Efficient member |
use shortcuts for commands |
enter commands quickly |
|
Forgetful member |
use multiple names for commands |
use the right command by using any intuitive name |
|
Forgetful member |
add an alternative name for a command |
use names that I am used to |
|
Forgetful member |
have suggestions for commands |
correct myself easily when I make a mistake |
|
Exco member |
view anonymous feedback |
see members' opinions of how the club can be improved |
|
Member with many members in the address book |
sort members by name |
locate a member easily |
|
Member |
choose fields that should be displayed in the members list |
view only those attributes that I want |
|
Member |
submit anonymous feedback |
give my opinions to improve the club system and facilities |
|
Member |
create a group chat |
broadcast messages |
|
Member |
chat with other members |
communicate with them |
|
Member |
know who is online |
I can chat with members in real time. |
|
Member |
see a list of my frequently viewed members |
I can quickly access them |
|
Member |
email profiles of members to others |
I can share the information easily |
|
Member |
print profiles of selected members |
view the information in the absence of a computer |
|
Socially active member |
link my social media |
I can directly post things that I do in the club |
|
Member |
change the theme of the application |
use the application with an appearance that I think looks best |
{More to be added}
Appendix D: Use Cases
(For all use cases below, the System is Club Connect
and the Actor is the user
, unless specified otherwise)
Use case: Delete member
System : Club Connect
Actor : Exco member
Precondition : User is logged in.
MSS
-
User requests to list members.
-
Club Connect shows a list of members.
-
User requests to delete a specific member in the list.
-
Club Connect deletes the member.
Use case ends.
Extensions
-
2a. The list only contains the User.
Use case ends.
-
3a. The given index is invalid.
-
3a1. Club Connect shows an error message.
Use case resumes at step 2.
-
Use case: Add member
System : Club Connect
Actor : Exco member
Precondition : User is logged in.
MSS
-
User requests to list members.
-
Club Connect shows a list of members.
-
User requests to add a member to the list.
-
Club Connect adds the member.
Use case ends.
Extensions
-
3a. The syntax of the add command is invalid.
-
3a1. Club Connect shows an error message.
-
3a1. Club Connect shows correct format for add command.
Use case resumes at step 2.
-
-
3a. The matric number of member already exists in Club Connect.
-
3a1. Club Connect shows an error message.
Use case resumes at step 2.
-
Use case: Show Help
System : Club Connect
Actor : Member
Precondition : User is logged in.
MSS
-
User requests help.
-
Club Connect shows usage instructions.
Use case ends.
Use case: Sort members
System : Club Connect
Actor : Member
Precondition : User is logged in.
MSS
-
User requests to sort by specified field.
-
Club Connect lists members sorted according to specified field.
Use case ends.
Extensions
-
1a. Invalid field specified.
-
1a1. Club Connect shows an error message and displays all possible valid fields.
Use case resumes at step 1.
-
-
1b. No field specified.
-
1b2. Club Connect shows list of members sorted by name.
Use case ends.
-
Use case: Exit
System : Club Connect
Actor : Member
Precondition : User is logged in.
MSS
-
User requests to exit Club Connect.
-
Club Connect exits.
Use case ends.
Use case: Undo command
System : Club Connect
Actor : Member
Precondition : User is logged in.
MSS
-
User requests to undo previous command.
-
Club Connect undoes previous command.
Use case ends.
Extensions
-
1a. Previous command is undoable.
-
1a1. Club Connect undoes latest undoable command.
Use case ends.
-
-
1b. No previous commands given.
Use case ends.
Use case: Redo command
System : Club Connect
Actor : Member
Precondition : User is logged in.
MSS
-
User requests to redo.
-
Club Connect redoes the change made by previous undo command.
Use case ends.
Extensions
-
1a. There are no redoable commands.
Use case ends.
Use case: Login
System : Club Connect
Actor : Member
Precondition : User is logged out.
MSS
-
Club Connect shows login screen.
-
User enters username and password.
-
Club Connect login as member with given username and password.
Use case ends.
Extensions
-
1a. Username and password combination invalid.
-
1a1. Club Connect shows an error message.
Use case resumes at step 1.
-
Use case: Logout
System : Club Connect
Actor : Member
Precondition : User is logged in.
MSS
-
User requests to logout.
-
Club Connect logouts to login screen.
Use case ends.
Use case: Find member
System : Club Connect
Actor : Member
Precondition : User is logged in.
MSS
-
User requests to find member by specified field using keyword.
-
Club Connect shows a list of members containing keyword in specified field.
Use case ends.
Extensions
-
1a. Invalid field specified.
-
1a1. Club Connect shows an error message and displays all possible valid fields.
Use case resumes at step 1.
-
-
1b. No field specified.
-
1b2. Club Connect shows a list of members containing keyword in any possible field.
Use case ends.
-
Appendix E: Non Functional Requirements
-
Should work on any mainstream OS as long as it has Java
1.8.0_60
or higher installed. -
Should be able to hold up to 1000 members without a noticeable sluggishness in performance for typical usage.
-
A user with above average typing speed for regular English text (i.e. not code, not system admin commands) should be able to accomplish most of the tasks faster using commands than using the mouse.
-
Should respond within 1 second.
-
Should work on 32- and 64- bit environments.
-
Should be easy to use for a first-time user.
-
Should be able to handle any sort of input, i.e. should recover from invalid input.
-
Should have friendly user guides and developer guides.
-
Should allow certain data to be private.
-
Should have command names that concisely describe their function.
-
Should be open-source.
-
Development should not cost money.
-
Should be able to work offline.
-
Should take up as little memory on the hard disk.
-
Should be compatible with all Operating System, i.e Windows and Macintosh.
-
Should save data regularly.
-
Current versions must be backward compatible with older versions to support undo.
{More to be added}
Appendix F: Glossary
- Abstraction
-
In Object-oriented Programming, abstraction is the mechanism by which users are provided with only the functionality, and not the implementation details. So, abstraction provides users with information on what an object does, rather than how it does it.
- Attribute
-
An attribute is a type of detail of a member. For example, an attribute of a member could be phone number, email, matriculation number and so on.
- Bitmap Image File
-
Bitmap Image file (BMP) is a file format that stores bitmap graphics data. It is device independent and you do not need a graphics adapter to display it. Images stored as BMP files can be losslessly compressed.
- Club
-
A student organisation or association at the National University of Singapore. These include (but are not limited to) Faculty/Non-Faculty clubs, Academic/Non-Academic Societies, Interest Groups and Sports groups. Some examples include Computing Club, Mathematics Society and Basketball Varsity Team.
- Club Book
-
The internal database of Club Connect that stores member, task and poll information.
- CLI
-
Acronym for Command Line Interface. It is a purely text-based interface for software. User respond to visual prompts by typing single commands into the interface and receive results as text as well. An example of CLI would be MS-DOS.
- CSV
-
A comma-separated values (CSV) file is a text file that uses a comma (",") to separate values. This allows data to be saved in a table structured format.
- Entry
-
A value added to a member’s attribute.
- Exco member
-
A member who is part of the Executive Committee of the club. Exco members are seen as the leaders of the club. Exco members can execute certain commands and view features that are not available to other members of the club.
An Exco member is still regarded as a member. |
- GUI
-
Acronnym for Graphical User Interface. In a GUI, the software interface consists of graphical icons, menus and/or other visual indicators to display information. Users can typically interact with these graphics, rather than just using text in the command line. For example, all Windows operating systems have a GUI.
- Mainstream OS
-
Windows, Linux, Unix, OS-X
- Member
-
One of the members who compose a group organization. They are the target users of our application.
- Private contact detail
-
A contact detail that is not meant to be shared with others
- Profile
-
Visual display of the information (attributes and entries) of a member.
Appendix G: Instructions for Manual Testing
Given below are instructions to test the app manually.
These instructions only provide a starting point for testers to work on; testers are expected to do more exploratory testing. |
G.1. Launch and Shutdown
-
Initial launch
-
Download the jar file and copy into an empty folder
-
Double-click the jar file
Expected: Shows the GUI with a set of sample contacts. The window size may not be optimum.
-
-
Saving window preferences
-
Resize the window to an optimum size. Move the window to a different location. Close the window.
-
Re-launch the app by double-clicking the jar file.
Expected: The most recent window size and location is retained.
-
G.2. Deleting a member
-
Deleting a member while all members are listed
-
Prerequisites: You must be logged in to Club Connect as an
Exco
member. List all members using thelist
command. Multiple members in the list. -
Test case:
delete 1
Expected: First contact is deleted from the list. Details of the deleted contact shown in the status message. Timestamp in the status bar is updated. -
Test case:
delete 0
Expected: No member is deleted. Error details shown in the status message. Status bar remains the same. -
Other incorrect delete commands to try:
delete
,delete x
(where x is larger than the list size),delete -1
(index must be a positive integer)
Expected: Similar to previous.
-
G.3. Adding a task
-
Adding a task
-
Prerequisites: You must be logged in to Club Connect.
-
Test case:
addtask desc/Test Task 1 d/20/05/2018 t/19:00
Expected: A task is created and its details are displayed on the task pane(far right). Timestamp in the status bar is updated. -
Test case:
addtask desc/Test Task 2 d/20/05/2018
Expected: No task is added. Error details shown in the status message. Status bar remains the same. -
Other incorrect commands to try:
addtask desc/Test Task 3 d/20/05/2018 t/19:65
(invalid time)addtask desc/Test Task 4 d/29/02/2018 t/19:00
(invalid leap day) Expected: Similar to previous.
-
G.4. Assigning a task
-
Assigning a task
-
Prerequisites: You must be logged in to Club Connect as an
Exco
member. -
Test case:
assigntask desc/Assigned Task 1 d/20/05/2018 t/18:00 m/A0123456H
Expected: A task is created and assigned toA0123456H
. Task details displayed on the task pane. Timestamp in the status bar is updated. -
Test case:
assigntask desc/Assigned Task 1 d/20/05/2018
Expected: No task is added. Error details shown in the status message. Status bar remains the same. -
Other incorrect commands to try:
assigntask
,assigntask desc/Assigned Task 1 d/20/05/2018 t/18:00 m/A1234T
,assigntask desc/Assigned Task 1 d/20/05/2018 m/A0123456K
(missing time field)
Expected: Similar to previous.
-
G.5. Deleting a task
-
Deleting a task while all tasks are listed
-
Prerequisites: List all your tasks using the
viewmytasks
command. Multiple tasks in the list. -
Test case:
deletetask 1
Expected: First task is deleted from the list. Details of the deleted task shown in the status message. Timestamp in the status bar is updated. -
Test case:
delete 0
Expected: No task is deleted. Error details shown in the status message. Status bar remains the same. -
Other incorrect commands to try:
deletetask
,deletetask x
(where x is larger than the task list size),deletetask -1
(index must be a positive integer)
Expected: Similar to previous.
-
G.6. Changing a task’s status
-
Changing a task’s status while all tasks are listed
-
Prerequisites: List all your tasks using the
viewmytasks
command. Multiple tasks in the list. All tasks are set toYet To Begin
. -
Test case:
changestatus 1 st/Completed
Expected: First task’s status is changed toCompleted
. Description of the modified task shown in the status message. Timestamp in the status bar is updated. -
Test case:
changestatus 0 st/Completed
Expected: No task is affected. Error details shown in the status message. Status bar remains the same. -
Other incorrect commands to try:
changestatus
,changestatus x st/In Progress
(where x is larger than the task list size),changestatus -1 st/Completed
(index must be a positive integer)
Expected: Similar to previous.
-
G.7. Changing a task’s assignee
-
Changing a task’s assignee while all tasks are listed
-
Prerequisites: You must be logged in to Club Connect as an
Exco
member. List all the tasks using theviewalltasks
command. Multiple tasks in the list. -
Test case:
changeassignee 1 m/A0123456H
Expected: First task’s assignee is changed toA0123456H
. Description of the modified task shown in the status message. Timestamp in the status bar is updated. -
Test case:
changeassignee 0 m/A0123456H
Expected: No task is affected. Error details shown in the status message. Status bar remains the same. -
Other incorrect commands to try:
changeassignee
,changeassignee x m/A1234567H
(where x is larger than the task list size),changeassignee -1 m/A2233445G
(index must be a positive integer)
Expected: Similar to previous.
-
G.8. Deleting a group
-
Deleting a group from Club Connect
-
Prerequisites: You must be logged in to Club Connect as an
Exco
member. -
Test case:
deletegroup g/Logistics
Expected: The group of all members previously part of theLogistics
group will be changed toMember
. The name of the deleted group shown in the status message. Timestamp in the status bar is updated. -
Test case:
deletegroup g/Member
Expected: No group is deleted. Error details shown in the status message. Status bar remains the same. -
Test case:
deletegroup
,deletegroup g/Exco
(mandatory group) Expected: Similar to previous.
-
G.9. Adding a poll
-
Adding a poll as an
Exco
member-
Prerequisites: Login as
Exco member
. -
Test case:
addpoll q/Which is the right answer? ans/Left ans/Right
Expected: New poll added in poll list with question "Which is the right answer?" and answers "Left" and "Right". Details of the added poll shown in the status message. Timestamp in the status bar is updated. -
Test case:
addpoll q/hello ans/bye ans/bye ans/jane
Expected: New poll added in poll list with question "hello" and answers "bye" and "jane". Details of the added poll shown in the status message. Timestamp in the status bar is updated. -
Test case: Type
addpoll q/hi ans/a ans/b ans/c
twice
Expected: For first command, new poll added in poll list with question "hi" and answers "a" and "b". Details of the added poll shown in the status message. Timestamp in the status bar is updated. Then for the second, no poll is added. Duplicate poll error will be shown in status message. Status bar remains the same. -
Other incorrect addpoll commands to try:
addpoll
,addpoll x
(where x is larger than the list size or is negative)
Expected: No poll is added. Error details shown in the status message. Status bar remains the same.
-
-
Adding a poll as an a non-
Exco
member-
Prerequisites: Login as a non-
Exco
member -
Test case:
addpoll q/Which is the right answer? ans/Left ans/Right
Expected: No poll is deleted. Error details shown in the status message. Status bar remains the same.
-
G.10. Deleting a poll
-
Deleting a poll as an
Exco
member-
Prerequisites: Login as
Exco member
and there are polls listed. -
Test case:
deletepoll 1
Expected: First poll is deleted from the list. Details of the deleted poll shown in the status message. Timestamp in the status bar is updated. -
Test case:
deletepoll 0
Expected: No poll is deleted. Error details shown in the status message. Status bar remains the same. -
Other incorrect delete commands to try:
deletepoll
,deletepoll x
(where x is larger than the list size or is negative)
Expected: Similar to previous.
-
-
Deleting a poll as an a non-
Exco
member-
Prerequisites: Login as a non-
Exco
member and there are polls listed. -
Test case:
deletepoll 1
Expected: No poll is deleted. Command requires Exco-member shown in status message. Status bar remains the same.
-
G.11. Voting in a poll
-
Voting in a poll as an
Exco
member-
Prerequisites: Login as
Exco member
and all polls in poll list are not yet voted by user. -
Test case:
vote 1 1
Expected: Vote success shown the status message. Timestamp in the status bar is updated. -
Other incorrect vote commands to try:
vote
,vote x y
(where x and/or y is larger than the poll list size or is negative)
Expected: No poll is voted for. Error details shown in the status message. Status bar remains the same.
-
-
Voting in a poll as a non-
Exco
member-
Prerequisites: Login as a non-
Exco
member and all polls in poll list are not yet voted by user. -
Test case:
vote 1 1
Expected: Vote success shown in the status message. Timestamp in the status bar is updated. Poll is removed from poll list. -
Other incorrect vote commands to try:
vote
,vote x y
(where x and/or y is larger than the poll list size or is negative)
Expected: No poll is voted for. Error details shown in the status message. Status bar remains the same.
-
G.12. Viewing results of polls
-
Viewing results of polls as an
Exco
member-
Prerequisites: Login as
Exco member
, there are polls in the poll list and polls don’t display results. -
Test case:
viewresults
Expected: Results of polls shown in poll list. View results success shown in status message. Timestamp in the status bar is updated.
-
-
Viewing results of polls as a non-
Exco
member-
Prerequisites: Login as a non-
Exco
member and there are polls in the poll list. -
Test case:
viewresults
Expected: No results are shown in poll list. Command requires Exco-member shown in status message. Status bar remains the same.
-
G.13. Hiding results of polls
-
Hiding results of polls as an
Exco
member-
Prerequisites: Login as
Exco member
and there are polls in the poll list and polls display results. -
Test case:
hideresults
Expected: Results of polls hidden in poll list. Hide results success shown in status message. Timestamp in the status bar is updated.
-
-
Hiding results of polls as a non-
Exco
member-
Prerequisites: Login as a non-
Exco
member and there are polls in the poll list. -
Test case:
vote 1 1
Expected: No results are shown in poll list. Command requires Exco-member shown in status message. Status bar remains the same.
-
G.14. Compressing member cards
-
Compressing members cards when they are decompressed
-
Prerequisites: Login and membercards are decompressed
-
Test case:
compress
Expected: Member cards become compressed. Compress success shown in status message. Timestamp in the status bar is updated.
-
-
Compressing members cards when they are already compressed
-
Prerequisites: Login and membercards are compressed
-
Test case:
compress
Expected: Member cards remain compressed. Compress success shown in status message. Timestamp in the status bar is updated.
-
G.15. Decompressing member cards
-
Decompressing members cards when they are compressed
-
Prerequisites: Login and membercards are decompressed
-
Test case:
decompress
Expected: Member cards become decompressed. Decompress success shown in status message. Timestamp in the status bar is updated.
-
-
Decompressing members cards when they are already compressed
-
Prerequisites: Login and membercards are decompressed
-
Test case:
compress
Expected: Member cards remain decompressed. Decompress success shown in status message. Timestamp in the status bar is updated.
-
G.16. Finding members
-
Find by specified field
-
Prerequisites: Login and there are more than 5 members in the application
-
Test case:
find n/John
Expected: Member list filtered to members with name containing John. Number of members found shown in status message. Timestamp in the status bar is updated. -
Other find commands to try:
find PREFIX
(where PREFIX refers to the prefix of the field to narrow down the search. Possible prefixes defined in UserGuide.adoc)
Expected: Find command will narrow down search by the specified field given by PREFIX
-
-
Find by all fields
-
Prerequisites: Login and there are more than 5 members in the application
-
Test case:
find lala
Expected: Member list filtered to members with fields containing lala. Number of members found shown in status message. Timestamp in the status bar is updated.
-
G.17. Importing members
-
Importing members as an
Exco
member-
Prerequisites: You must be logged in to Club Connect as an
Exco
member. -
Test case:
import C:/Users/John/Desktop/correctly_formatted_data.csv
Expected: All members in the fileC:/Users/John/Desktop/correctly_formatted_data.csv
who have valid entries and do not share the same matriculation member with any member in Club Connect are added toClub Members
. The number of members imported is shown in the status message. Timestamp in the status bar is updated. -
Test case:
import C:/Users/John/Desktop/data_in_incorrect_format.csv
Expected: No members are added to Club Connect. Error details are shown in the status message. Timestamp in the status bar is updated. -
Test case:
import C:/Users/John/Desktop/does_not_exist.csv
Expected: No members are added to Club Connect. Error window pops up. Error details are shown in the status message. Status bar remains the same. -
Incorrect import commands to try:
import
,import C:/Users/John/members.txt
Expected: No members are added to Club Connect. Error details are shown in the status message. Status bar remains the same.
-
-
Importing members as a non-
Exco
member-
Prerequisites: You must be logged in to Club Connect and you must not be an
Exco
member. -
Test case:
import C:/Users/John/Desktop/correctly_formatted_data.csv
Expected: No members are added to Club Connect. Command requires Exco-member shown in status message. Status bar remains the same. -
Test case:
import C:/Users/John/Desktop/data_in_incorrect_format.csv
Expected: Similar to previous. -
Incorrect import commands to try:
import
,import C:/Users/John/members.txt
Expected: No members are added to Club Connect. Error details are shown in the status message. Status bar remains the same.
-
G.18. Exporting members
-
Exporting members as a member
-
Prerequisites: You must be logged in to Club Connect.
-
Test case:
export C:/Users/John/Desktop/does_not_exist.csv
Expected: Writes data of all members in Club Connect toC:/Users/John/Desktop/does_not_exist.csv
. Success message is shown in the status message. Status bar remains the same. -
Test case:
export C:/Users/John/Desktop/already_exists.csv
Expected: OverwritesC:/Users/John/Desktop/already_exists.csv
with data of all members in Club Connect. Success message is shown in the status message.Status bar remains the same. -
Incorrect export commands to try:
export
,export C:/Users/John/members.txt
Expected: No members are exported to a file. Error details are shown in the status message. Status bar remains the same.
-
G.19. Changing profile photo
-
Changing your profile photo when you have selected yourself
-
Prerequisites: You must be logged in to Club Connect.
-
Test case:
changepic C:/Users/John/Desktop/valid_photo.jpg
Expected: Your profile photo changes to the image atC:/Users/John/Desktop/valid_photo.jpg
. Your image in theLogged in
panel, theClub Members
panel, and theMember Profile
panel changes to this new photo. Timestamp in the status bar is updated. -
Test case:
changepic C:/Users/John/Desktop/does_not_exist.jpg
Expected: Your profile photo is unchanged. Error window pops up. Invalid photo path is shown in status message. Your image in theLogged in
panel, theClub Members
panel, and theMember Profile
panel remains the same. Status bar remains the same. -
Test case:
changepic C:/Users/John/Desktop/
Expected: Your profile photo is unchanged. Error details are shown in status message. Your image in theLogged in
panel, theClub Members
panel, and theMember Profile
panel remains the same. Status bar remains the same. -
Other incorrect change photo commands to try:
changepic
,changepic C:/Users/John/image.bmp
Expected: Similar to previous.
-
-
Changing your profile photo when you have not selected yourself
-
Prerequisites: You must be logged in to Club Connect.
-
Test case:
changepic C:/Users/John/Desktop/valid_photo.jpg
Expected: Your profile photo changes to the image atC:/Users/John/Desktop/valid_photo.jpg
. Your image in theLogged in
panel and theClub Members
panel changes. Timestamp in the status bar is updated. -
Test case:
changepic C:/Users/John/Desktop/does_not_exist.jpg
Expected: Your profile photo is unchanged. Error window pops up. Invalid photo path is shown in status message. Your image in theLogged in
panel and theClub Members
panel remains the same. Status bar remains the same. -
Test case:
changepic C:/Users/John/Desktop/
Expected: Your profile photo is unchanged. Error details are shown in status message. Your image in theLogged in
panel and theClub Members
panel panel remains the same. Status bar remains the same. -
Other incorrect change photo commands to try:
changepic
,changepic C:/Users/John/image.bmp
Expected: Similar to previous.
-
G.20. Removing profile photo
-
Removing your profile photo when it is not the default photo and you have selected yourself
-
Prerequisites: You must be logged in to Club Connect. You must have changed your profile photo.
-
Test case:
removepic
Expected: Your profile photo changes to Club Connect’s default profile photo. Your image in theLogged in
panel, theClub Members
panel, and theMember Profile
panel changes. Timestamp in the status bar is updated.
-
-
Removing your profile photo when it is not the default photo and you have not selected yourself
-
Prerequisites: You must be logged in to Club Connect. You must have changed your profile photo.
-
Test case:
removepic
Expected: Your profile photo changes to Club Connect’s default profile photo. Your image in theLogged in
panel and theClub Members
panel changes. Timestamp in the status bar is updated.
-
-
Removing your profile photo when it is the default photo
-
Prerequisites: You must be logged in to Club Connect. You must have not changed your profile photo.
-
Test case:
removepic
Expected: Your profile photo remains as Club Connect’s default profile photo. Your image in theLogged in
panel, theClub Members
panel, and theMember Profile
panel remains the same. Timestamp in the status bar is updated.
-
G.21. Deleting a tag
-
Deleting a tag as an
Exco
member-
Prerequisites: You must be logged in to Club Connect as an
Exco
member. -
Test case:
deletetag t/Head
Expected: The tag from all members previously tagged withHead
will be removed. The name of the deleted taglogin u/A0123456H pw/password shown in the status message. Timestamp in the status bar is updated. -
Test case:
deletetag t/This-does-not-exist
Expected: No tag is deleted as the tag does not exist. Error details are shown in the status message. Status bar remains the same. -
Incorrect delete tag commands to try:
deletetag
,deletetag t/invalid format tag
Expected: No tag is deleted as the tag name format is invalid. Error details are shown in the status message. Status bar remains the same.
-
-
Deleting a tag as a non-
Exco
member-
Prerequisites: You must be logged in to Club Connect and you must not be an
Exco
member. -
Test case:
deletetag t/Head
Expected: No tags are deleted from members. Command requires Exco-member shown in status message. Status bar remains the same. -
Test case:
deletetag t/This-does-not-exist
Expected: Similar to previous. -
Incorrect delete tag commands to try:
deletetag
,deletetag t/invalid format tag
Expected: No tag is deleted as the tag name format is invalid. Relevant error details are shown in the status message. Status bar remains the same.
-